


How Do I Use Enumerations in PHP, Including Workarounds for Versions Before 8.1?
Enumerations in PHP: PHP 8.1 Support and Workarounds
PHP has long lacked native enumerations, leaving developers seeking workarounds. However, with the release of PHP 8.1, full-fledged enum support has finally arrived.
PHP 8.1: Native Enums
Starting with PHP 8.1, enums are officially supported. They provide a concise and type-safe way to define sets of predefined values:
enum DaysOfWeek: int { case Sunday = 0; case Monday = 1; // etc. }
Workarounds for PHP 8.0 and Earlier
Prior to PHP 8.1, several workarounds were commonly used:
- Constants: Constants provide global, immutable values. However, they can lead to namespace collisions and are not as versatile as true enums.
- Arrays: Arrays are more flexible but can be overridden and lack autofill support in IDEs.
A more advanced workaround involves creating a base enum class with static validation methods:
abstract class BasicEnum { // ... public static function isValidName($name, $strict = false) {} public static function isValidValue($value, $strict = true) {} }
Extending this class allows for straightforward input validation:
abstract class DaysOfWeek extends BasicEnum { // ... } DaysOfWeek::isValidName('Humpday'); // false DaysOfWeek::isValidValue(7); // false
SplEnum for PHP 5.3
If using PHP 5.3 or later, SplEnum provides a more robust workaround:
$days = new SplEnum(array( 'Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday' )); if ($days->isValid('Monday')) { // ... }
Conclusion
Native enumerations in PHP 8.1 simplify code and improve type safety. For earlier versions, various workarounds exist, including constants, arrays, and the BasicEnum or SplEnum classes.
The above is the detailed content of How Do I Use Enumerations in PHP, Including Workarounds for Versions Before 8.1?. For more information, please follow other related articles on the PHP Chinese website!
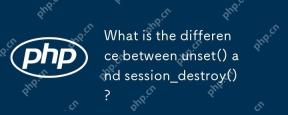
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
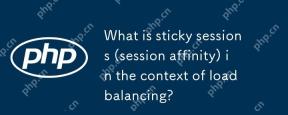
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
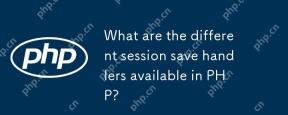
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
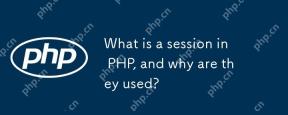
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
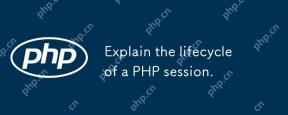
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
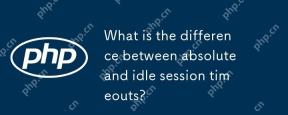
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
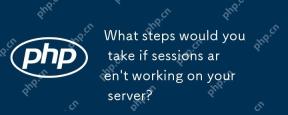
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
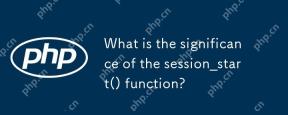
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
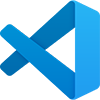
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
