


Simplest Two-Way Encryption in PHP
Important Note:
Before proceeding, it's crucial to emphasize that this article aims to provide a simplified understanding of unauthenticated encryption. For robust data protection, always consider using industry-standard authenticated encryption libraries or bcrypt/argon2 for password storage.
Native Cryptographic Methods
If using PHP 5.4 or later, consider employing the openssl_* functions for cryptographic operations. These offer robust encryption functionalities with native support.
Simple Encryption and Decryption
OpenSSL's openssl_encrypt() and openssl_decrypt() functions provide an accessible way to encrypt and decrypt data. The recommended encryption algorithm is AES-CTR in its 128, 192, or 256-bit variants.
Caution: Avoid using mcrypt due to its deprecation and potential security risks.
Simple Encryption/Decryption Wrapper
To simplify the encryption and decryption process, you can utilize the following wrapper class:
class UnsafeCrypto { // Encryption method (CTR mode) const METHOD = 'aes-256-ctr'; /** * Encrypts data using the specified key. * * @param string $message Plaintext message * @param string $key Encryption key * @param bool $encode Whether to encode the result * * @return string Ciphertext */ public static function encrypt($message, $key, $encode = false) { // Generate random IV $ivSize = openssl_cipher_iv_length(self::METHOD); $iv = openssl_random_pseudo_bytes($ivSize); // Encrypt using OpenSSL $ciphertext = openssl_encrypt($message, self::METHOD, $key, OPENSSL_RAW_DATA, $iv); // Concatenate IV and ciphertext if ($encode) { return base64_encode($iv . $ciphertext); } return $iv . $ciphertext; } /** * Decrypts data using the specified key. * * @param string $message Ciphertext * @param string $key Encryption key * @param bool $encoded Whether the message is encoded * * @return string Decrypted message */ public static function decrypt($message, $key, $encoded = false) { if ($encoded) { $message = base64_decode($message, true); if ($message === false) { throw new Exception('Encryption failure'); } } // Extract IV and ciphertext $ivSize = openssl_cipher_iv_length(self::METHOD); $iv = substr($message, 0, $ivSize); $ciphertext = substr($message, $ivSize); // Decrypt using OpenSSL $plaintext = openssl_decrypt($ciphertext, self::METHOD, $key, OPENSSL_RAW_DATA, $iv); return $plaintext; } }
Authenticated Encryption
The above approach only provides encryption, leaving data vulnerable to tampering. To address this, implement an authenticated encryption scheme:
class SaferCrypto extends UnsafeCrypto { // MAC algorithm const HASH_ALGO = 'sha256'; /** * Encrypts and authenticates data using the specified key. * * @param string $message Plaintext message * @param string $key Encryption key * @param bool $encode Whether to encode the result * * @return string Encrypted and authenticated data */ public static function encrypt($message, $key, $encode = false) { // Split key into encryption and authentication keys list($encKey, $authKey) = self::splitKeys($key); // Encrypt using UnsafeCrypto::encrypt $ciphertext = parent::encrypt($message, $encKey); // Calculate MAC $mac = hash_hmac(self::HASH_ALGO, $ciphertext, $authKey, true); // Concatenate MAC and ciphertext if ($encode) { return base64_encode($mac . $ciphertext); } return $mac . $ciphertext; } /** * Decrypts and authenticates data using the specified key. * * @param string $message Encrypted and authenticated data * @param string $key Encryption key * @param bool $encoded Whether the message is encoded * * @throws Exception * * @return string Decrypted message */ public static function decrypt($message, $key, $encoded = false) { // Split key list($encKey, $authKey) = self::splitKeys($key); // Decode message if necessary if ($encoded) { $message = base64_decode($message, true); if ($message === false) { throw new Exception('Encryption failure'); } } // Extract MAC and ciphertext $hs = strlen(hash(self::HASH_ALGO, '', true), '8bit'); $mac = substr($message, 0, $hs); $ciphertext = substr($message, $hs); // Calculate expected MAC $expectedMac = hash_hmac(self::HASH_ALGO, $ciphertext, $authKey, true); // Verify MAC if (!self::hashEquals($mac, $expectedMac)) { throw new Exception('Encryption failure'); } // Decrypt message using UnsafeCrypto::decrypt $plaintext = parent::decrypt($ciphertext, $encKey); return $plaintext; } /** * Splits a key into two separate keys for encryption and authentication. * * @param string $masterKey Master key * * @return string[] Array of encryption and authentication keys */ protected static function splitKeys($masterKey) { return [ hash_hmac(self::HASH_ALGO, 'ENCRYPTION', $masterKey, true), hash_hmac(self::HASH_ALGO, 'AUTHENTICATION', $masterKey, true) ]; } /** * Compares two strings without leaking timing information * (PHP 7+). * * @param string $a * @param string $b * * @return bool */ protected static function hashEquals($a, $b) { if (function_exists('hash_equals')) { return hash_equals($a, $b); } $nonce = openssl_random_pseudo_bytes(32); return hash_hmac(self::HASH_ALGO, $a, $nonce) === hash_hmac(self::HASH_ALGO, $b, $nonce); } }
Remember, for robust security, consider utilizing reputable encryption libraries.
The above is the detailed content of How Can I Implement Simple Two-Way Encryption in PHP Using OpenSSL?. For more information, please follow other related articles on the PHP Chinese website!
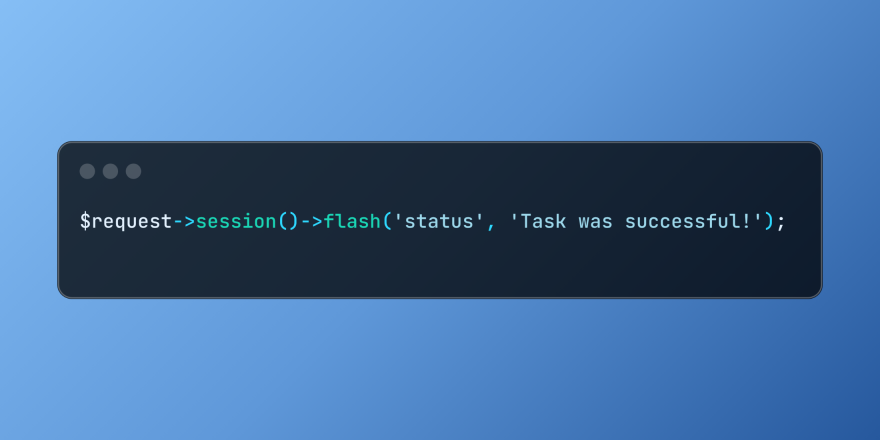
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
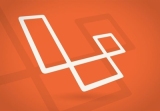
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
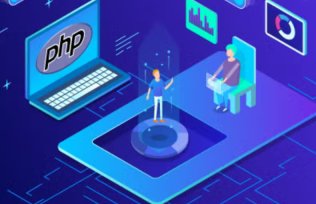
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
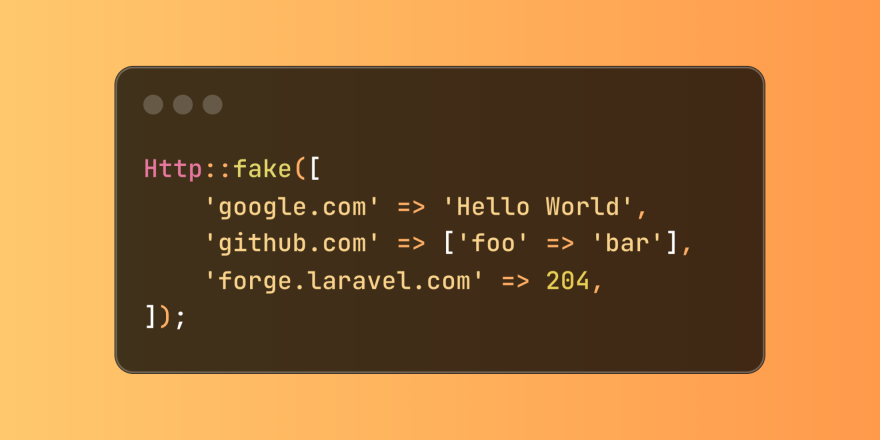
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
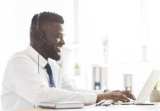
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
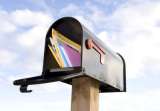
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov
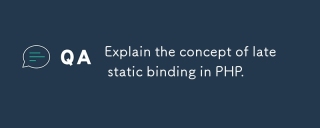
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
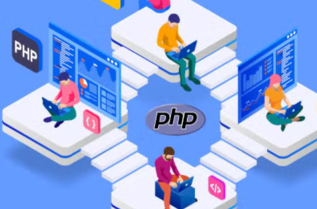
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
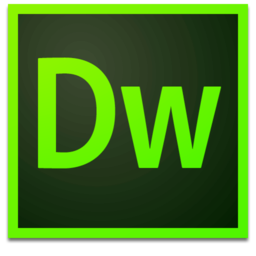
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
