


How Does Variable Scope and Shadowing Enhance Code Control and Data Integrity in Go?
Variable Scope and Shadowing: Applications in Go
Variable scoping and shadowing in Go are powerful techniques that provide control over variable visibility and data integrity. Here are various scenarios where these techniques find useful applications:
Different Forms of Shadowing
Using Short-hand Assignment
package main import "fmt" func main() { i := 10 // scope: main j := 4 // Shadowing i within this block for i := 'a'; i <h4 id="Using-Pairs">Using { } Pairs</h4><pre class="brush:php;toolbar:false">package main import "fmt" func main() { i := 1 j := 2 // Create new scope with { } block { // Shadow i with a new local variable i := "hi" // Increment j j++ fmt.Println(i, j) // hi 3 } // Original i comes into scope fmt.Println(i, j) // 1 3 }
Using Function Calls
package main import "fmt" func fun(i int, j *int) { i++ // Implicitly shadowing (used as local) *j++ // Explicitly shadowing (used as global) fmt.Println(i, *j) // 11 21 } func main() { i := 10 // scope: main j := 20 fun(i, &j) fmt.Println(i, j) // 10 21 }
Shadowing Global Variables
package main import "fmt" var i int = 1 // Global variable func main() { j := 2 fmt.Println(i, j) // 1 2 // Shadowing global i i := 10 fmt.Println(i, j) // 10 2 fun(i, j) // 10 2 } func fun(i, j int) { fmt.Println(i, j) // 10 2 }
Advantages of Scope and Shadowing
- Preserved Data Integrity: Variables in outer scopes cannot be accessed from inner scopes, preventing data corruption.
Conclusion
Variable scope and shadowing techniques in Go offer flexibility, data protection, and opportunities for efficient code organization. By understanding their applications, developers can optimize their Go codebase and effectively handle variable visibility and manipulation.
The above is the detailed content of How Does Variable Scope and Shadowing Enhance Code Control and Data Integrity in Go?. For more information, please follow other related articles on the PHP Chinese website!
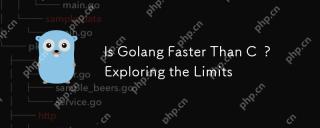
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
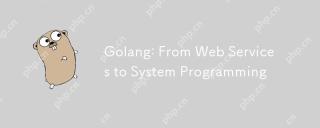
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
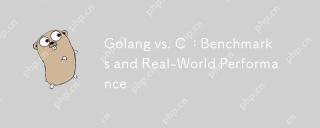
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
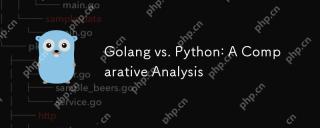
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.
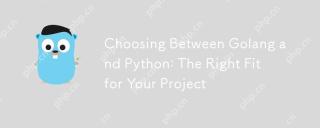
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
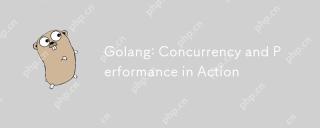
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
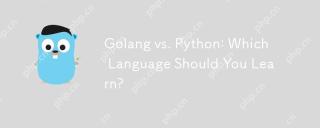
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
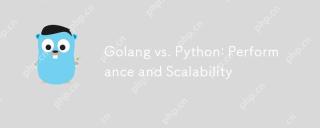
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
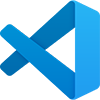
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft