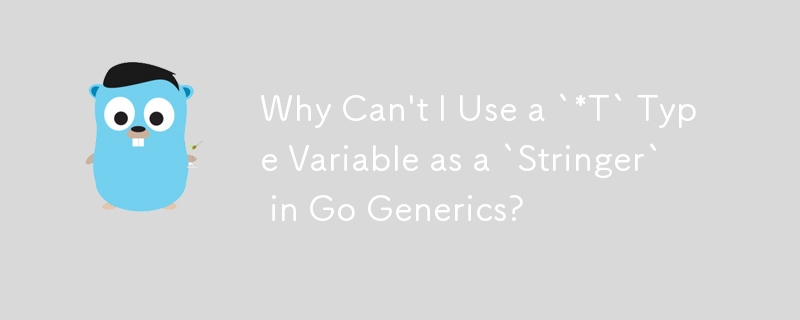
Understanding the "Cannot Use Variable of Type *T as Type Stringer in Argument" Error in Go Generics
In the code snippet provided, you're attempting to generically invoke a function do that expects a parameter of type stringer, but you're passing a pointer to a type parameter T instead.
The Go compiler is raising an error because:
-
Type Identity and Parameters: T is not inherently equivalent to its constraint FooBar. Therefore, *T is not the same as *FooBar or *bar.
-
Missing Method: The stringer interface requires a method named a(). Currently, your foo and bar types implement this method on pointer receivers, but *T does not inherently possess this method.
Fixing the Issue
There are two main approaches to resolving this issue:
1. Assert Type Safety with Type Assertion (Less Optimal)
- Assert that *T implements stringer using any(t).(stringer) within the do function.
- This approach may lead to panics if T does not actually implement stringer.
2. Redefine Constraint and Customize Generics (Preferred)
- Add stringer as a constraint to the FooBar interface.
- Use pointer receivers for the foo and bar types to align with the constraint.
- Introduce a second type parameter that specifies the constraint within the FooBar interface.
- Pass an instance of the constrained type as an argument to blah.
Revised Code:
type FooBar[T foo | bar] interface {
*T
stringer
}
func blah[T foo | bar, U FooBar[T]]() {
var t T
do(U(&t))
}
func main() {
blah[foo]()
}
In this revised code:
- The FooBar interface includes stringer as a constraint.
- The foo and bar types have pointer receivers for their a() methods.
- U is constrained by FooBar[T], ensuring that it satisfies both FooBar and stringer constraints.
- blah takes an argument of type U, which is an instance of the constrained FooBar interface.
The above is the detailed content of Why Can't I Use a `*T` Type Variable as a `Stringer` in Go Generics?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn