Defer Usage Clarification
In Go, the "defer" statement allows us to schedule a function to be executed just before the surrounding function returns. However, this can lead to confusion when dealing with variables that are modified within the enclosing function.
Original Issue
Consider the following function:
func printNumbers() { var x int defer fmt.Println(x) for i := 0; i <p>According to the Go specification, when a "defer" statement is executed, the function value and parameters are evaluated and stored for later execution. This means that when the function is eventually called, the value of x will still be 0 because it was evaluated at the time of deferral.</p><h3 id="Solution-with-Anonymous-Function">Solution with Anonymous Function</h3><p>To resolve this issue, we can use an anonymous function within the "defer" statement:</p><pre class="brush:php;toolbar:false">defer func() { fmt.Println(x) }()
Here, x is not a parameter of the anonymous function, so it will not be evaluated when the "defer" statement is executed. Instead, the value of x will be captured at the time the anonymous function is called, ensuring that the most up-to-date value is printed.
Alternative Solutions
-
Using a Pointer:
var x int defer func() { fmt.Println(&x) }()
This approach uses a pointer to x as the parameter of the deferred function. When the "defer" statement is executed, only the pointer is evaluated, not the value of x. When the deferred function is called, it will access the current value of x through the pointer.
-
Using a Custom Type:
type MyInt int func (m *MyInt) String() string { return strconv.Itoa(int(*m)) } var x MyInt defer fmt.Println(&x)
This solution is similar to the pointer approach but uses a custom type (MyInt) that implements the String() method. By implementing String(), we can control how the value of x is printed.
-
Using a Slice:
var x []int defer fmt.Println(x)
Slicing is a descriptor type in Go, which means that its value is a reference to an underlying array. When we defer a slice, only the reference is evaluated, not the actual array elements. As a result, any changes made to the slice elements after the deferral will be reflected in the printed output.
-
Wrapping in a Struct:
type Wrapper struct { Value int } var x Wrapper defer fmt.Println(&x)
This approach is similar to using a pointer, but we wrap the value in a struct to avoid having to dereference the pointer in the deferred function.
The above is the detailed content of How to Correctly Use `defer` in Go with Modified Variables?. For more information, please follow other related articles on the PHP Chinese website!
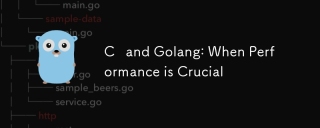
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
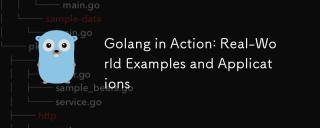
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
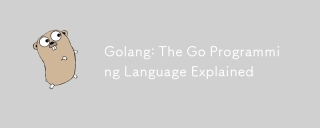
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
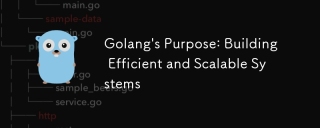
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
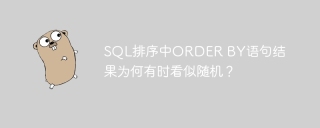
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
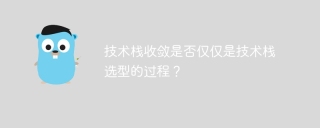
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
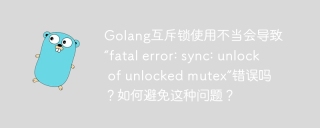
Golang ...
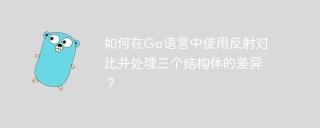
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.