


Difference between make_shared and Constructor-Initialized shared_ptr
Scenario
Consider the following code snippets:
std::shared_ptr<object> p1 = std::make_shared<object>("foo"); std::shared_ptr<object> p2(new Object("foo"));</object></object></object>
Understanding why make_shared is more efficient than using the shared_ptr constructor directly requires a step-by-step analysis of the operations involved.
Operations Comparison
make_shared
- Performs a single heap allocation to create a contiguous memory block for both the control block (meta data) and the managed object.
Constructor-Initialized shared_ptr
- Invokes new Obj("foo"), which creates a heap allocation for the managed object.
- Then, the shared_ptr constructor is called, which performs another heap allocation for the control block.
Memory Allocations
make_shared allocates memory only once, while the constructor-initialized shared_ptr allocates memory twice. This makes make_shared more efficient.
Exception Safety
In C 17, the evaluation order of function arguments was revised, eliminating an exception safety concern with the constructor-initialized shared_ptr approach. However, let's consider the example:
void F(const std::shared_ptr<lhs> &lhs, const std::shared_ptr<rhs> &rhs) { /* ... */ } F(std::shared_ptr<lhs>(new Lhs("foo")), std::shared_ptr<rhs>(new Rhs("bar")));</rhs></lhs></rhs></lhs>
If an exception is thrown during the Rhs constructor, the memory allocated for Lhs will be lost because it was not immediately passed to the shared_ptr constructor. make_shared avoids this issue by eliminating this intermediate step.
Disadvantage of make_shared
However, make_shared has a disadvantage: since it allocates the control block and managed object in a single heap block, the memory for both cannot be deallocated independently. This means that weak pointers can keep the control block alive indefinitely, potentially preventing both the control block and the managed object from being deallocated.
The above is the detailed content of `make_shared` vs. Constructor-Initialized `shared_ptr`: What's the Performance and Exception Safety Difference?. For more information, please follow other related articles on the PHP Chinese website!
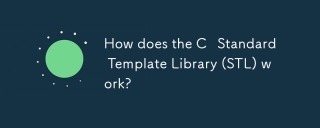
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
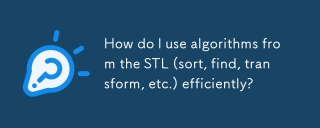
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
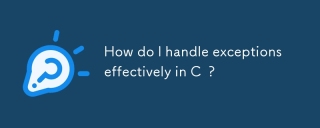
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
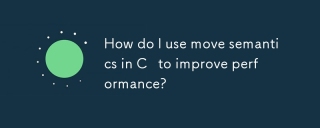
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
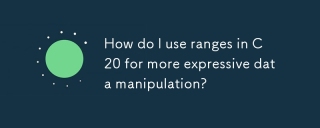
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
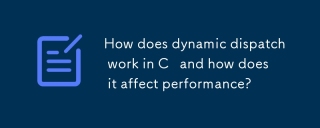
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
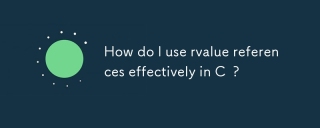
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
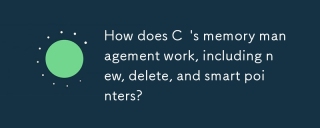
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
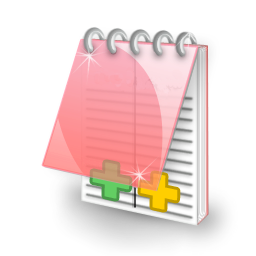
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
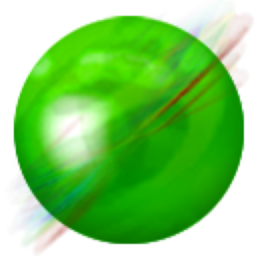
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
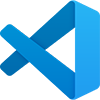
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
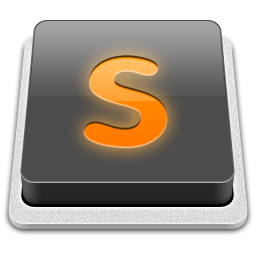
SublimeText3 Mac version
God-level code editing software (SublimeText3)
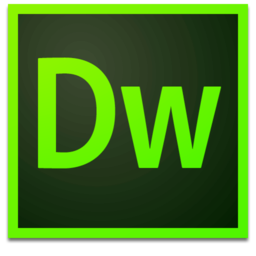
Dreamweaver Mac version
Visual web development tools