Concurrent Map Errors in Go: Resolving "fatal error: concurrent map read and map write"
When working with concurrent maps in Go, it's crucial to ensure thread safety to prevent race conditions. One such error that can occur is "fatal error: concurrent map read and map write." This error indicates that multiple goroutines are concurrently attempting to access the same map, causing access conflicts.
Possible Solutions
To address this error, several approaches can be employed:
1. Using sync.RWMutex
This method involves controlling access to the map using a sync.RWMutex. It is suitable for scenarios where single reads and writes occur, but not loops over the map. Here's an example:
var ( someMap = map[string]string{} someMapMutex = sync.RWMutex{} ) // Read operation func getSomeValue() { someMapMutex.RLock() defer someMapMutex.RUnlock() val := someMap["key"] // Use val... } // Write operation func setSomeValue() { someMapMutex.Lock() defer someMapMutex.Unlock() someMap["key"] = "value" }
2. Using syncmap.Map
syncmap.Map is a thread-safe map type that automatically handles concurrent access, providing a more efficient solution than sync.RWMutex, especially for scenarios involving loops over the map.
import "sync" var ( someMap = syncmap.Map{} ) func iterateOverMap() { someMap.Range(func(key, value interface{}) bool { // Use key and value... return true // Continue iterating }) }
Additional Considerations
- Utilize the "-race" option when running your server to detect and resolve potential race conditions.
- Consider using a channel or queue to communicate map-related operations between goroutines.
- Employ a mutex to protect sensitive sections of code that access the map concurrently.
These solutions effectively resolve concurrent map access issues and enhance the stability of your Go application.
The above is the detailed content of How to Solve 'fatal error: concurrent map read and map write' in Go?. For more information, please follow other related articles on the PHP Chinese website!
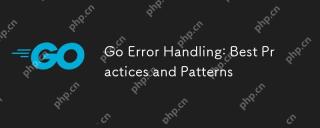
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
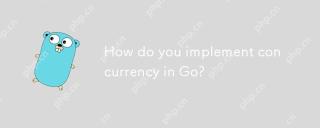
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
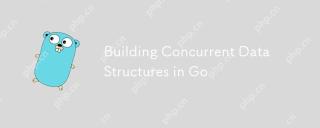
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
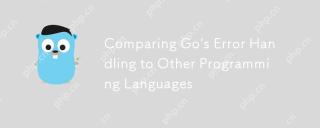
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand
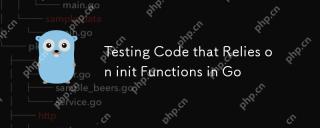
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
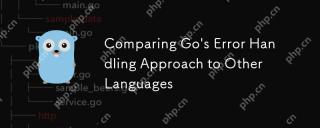
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
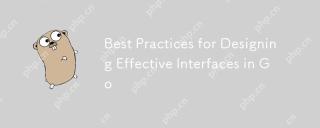
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
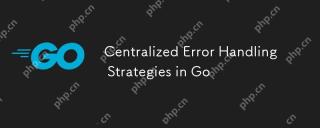
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment
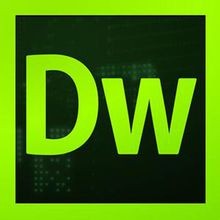
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
