Accessing Values in Multidimensional PHP Arrays
Accessing elements within arrays can be tricky, especially when dealing with multidimensional arrays. Let's address a common issue faced when trying to retrieve values from a multidimensional array containing nested arrays.
Scenario
Consider the following multidimensional array:
$array = [ [ 'id' => 1, 'name' => 'Bradeley Hall Pool', // ... other properties ], [ 'id' => 2, 'name' => 'Farm Pool', // ... other properties 'suitability' => [ // A nested array [ 'fk_species_id' => 4, 'species_name' => 'Barbel', // ... other properties ] ], ] ];
The goal is to access the species_name property within the nested suitability array.
Solution
To access a value within a nested array, you need to use the appropriate indexes or keys to traverse the array structure. Here's how you would access the species_name property in the example array:
$speciesName = $array[1]['suitability'][0]['species_name'];
In this case, $array[1] retrieves the second top-level array, $array[1]['suitability'] retrieves the nested suitability array, and $array[1]['suitability'][0] finally gets the first item in that nested array.
Looping through Nested Arrays
To loop through all the elements in the suitability array, you can use a nested foreach loop:
foreach ($array as $topLevelArray) { if (isset($topLevelArray['suitability'])) { foreach ($topLevelArray['suitability'] as $suitabilityItem) { echo $suitabilityItem['species_name'] . PHP_EOL; } } }
This loop checks if the top-level array contains a suitability key and then iterates over the items in that nested array, printing the species_name property for each item.
By understanding the syntax and structure of multidimensional arrays, you can efficiently access and manipulate their elements to obtain the data you need.
The above is the detailed content of How Do I Access Values in Nested PHP Arrays?. For more information, please follow other related articles on the PHP Chinese website!
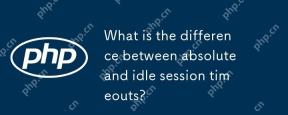
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
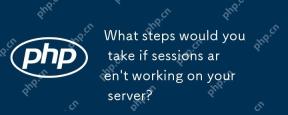
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
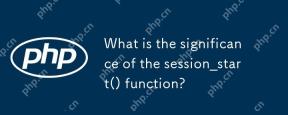
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.
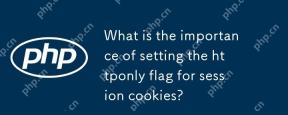
Setting the httponly flag is crucial for session cookies because it can effectively prevent XSS attacks and protect user session information. Specifically, 1) the httponly flag prevents JavaScript from accessing cookies, 2) the flag can be set through setcookies and make_response in PHP and Flask, 3) Although it cannot be prevented from all attacks, it should be part of the overall security policy.
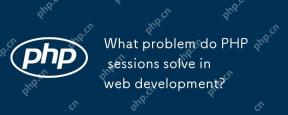
PHPsessionssolvetheproblemofmaintainingstateacrossmultipleHTTPrequestsbystoringdataontheserverandassociatingitwithauniquesessionID.1)Theystoredataserver-side,typicallyinfilesordatabases,anduseasessionIDstoredinacookietoretrievedata.2)Sessionsenhances
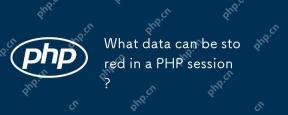
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
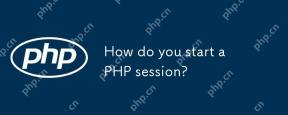
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
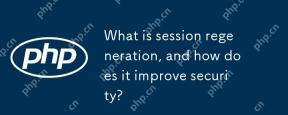
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
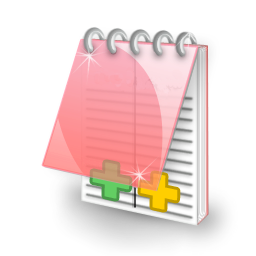
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
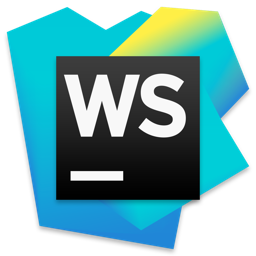
WebStorm Mac version
Useful JavaScript development tools
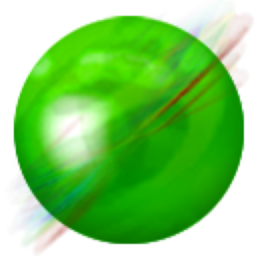
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
