Understanding complex unpacking expressions
Consider the following expression:
a, b = 1, 2 # 简单序列赋值 a, b = ['green', 'blue'] # 列表赋值 a, b = 'XY' # 字符串赋值 a, b = range(1,5,2) # 任何可迭代对象都可以 # 嵌套序列赋值 (a,b), c = "XY", "Z" # a = 'X', b = 'Y', c = 'Z' (a,b), c = "XYZ" # 错误 -- 太多值需要拆包 (a,b), c = "XY" # 错误 -- 需要拆包的数值太少 (a,b), c, = [1,2],'this' # a = '1', b = '2', c = 'this' (a,b), (c,) = [1,2],'this' # 错误 -- 太多值需要拆包 # 扩展序列拆包 a, *b = 1,2,3,4,5 # a = 1, b = [2,3,4,5] *a, b = 1,2,3,4,5 # a = [1,2,3,4], b = 5 a, *b, c = 1,2,3,4,5 # a = 1, b = [2,3,4], c = 5 a, *b = 'X' # a = 'X', b = [] *a, b = 'X' # a = [], b = 'X' a, *b, c = "XY" # a = 'X', b = [], c = 'Y' a, *b, c = "X...Y" # a = 'X', b = ['.','.','.'], c = 'Y' a, b, *c = 1,2,3 # a = 1, b = 2, c = [3] a, b, c, *d = 1,2,3 # a = 1, b = 2, c = 3, d = [] a, *b, c, *d = 1,2,3,4,5 # 错误 -- 赋值中出现了两个星号表达式 (a,b), c = [1,2],'this' # a = '1', b = '2', c = 'this' (a,b), *c = [1,2],'this' # a = '1', b = '2', c = ['this'] (a,b), c, *d = [1,2],'this' # a = '1', b = '2', c = 'this', d = [] (a,b), *c, d = [1,2],'this' # a = '1', b = '2', c = [], d = 'this' (a,b), (c, *d) = [1,2],'this' # a = '1', b = '2', c = 't', d = ['h', 'i', 's'] *a = 1 # 错误 -- 目标必须在一个列表或元组中 *a = (1,2) # 错误 -- 目标必须在一个列表或元组中 *a, = (1,2) # a = [1,2] *a, = 1 # 错误 -- 'int' 对象不可迭代 *a, = [1] # a = [1] *a = [1] # 错误 -- 目标必须在一个列表或元组中 *a, = (1,) # a = [1] *a, = (1) # 错误 -- 'int' 对象不可迭代 *a, b = [1] # a = [], b = 1 *a, b = (1,) # a = [], b = 1 (a,b),c = 1,2,3 # 错误 -- 太多值需要拆包 (a,b), *c = 1,2,3 # 错误 - 'int' 对象不可迭代 (a,b), *c = 'XY', 2, 3 # a = 'X', b = 'Y', c = [2,3] # 扩展序列拆包 -- 嵌套 (a,b),c = 1,2,3 # 错误 -- 太多值需要拆包 *(a,b), c = 1,2,3 # a = 1, b = 2, c = 3 *(a,b) = 1,2 # 错误 -- 目标必须在一个列表或元组中 *(a,b), = 1,2 # a = 1, b = 2 *(a,b) = 'XY' # 错误 -- 目标必须在一个列表或元组中 *(a,b), = 'XY' # a = 'X', b = 'Y' *(a, b) = 'this' # 错误 -- 目标必须在一个列表或元组中 *(a, b), = 'this' # 错误 -- 拆包的值太多了 *(a, *b), = 'this' # a = 't', b = ['h', 'i', 's'] *(a, *b), c = 'this' # a = 't', b = ['h', 'i'], c = 's' *(a,*b), = 1,2,3,3,4,5,6,7 # a = 1, b = [2, 3, 3, 4, 5, 6, 7] *(a,*b), *c = 1,2,3,3,4,5,6,7 # 错误 -- 赋值中出现了两个星号表达式 *(a,*b), (*c,) = 1,2,3,3,4,5,6,7 # 错误 -- 'int' 对象不可迭代 *(a,*b), c = 1,2,3,3,4,5,6,7 # a = 1, b = [2, 3, 3, 4, 5, 6], c = 7 *(a,*b), (*c,) = 1,2,3,4,5,'XY' # a = 1, b = [2, 3, 4, 5], c = ['X', 'Y'] *(a,*b), c, d = 1,2,3,3,4,5,6,7 # a = 1, b = [2, 3, 3, 4, 5], c = 6, d = 7 *(a,*b), (c, d) = 1,2,3,3,4,5,6,7 # 错误 -- 'int' 对象不可迭代 *(a,*b), (*c, d) = 1,2,3,3,4,5,6,7 # 错误 -- 'int' 对象不可迭代 *(a,*b), *(c, d) = 1,2,3,3,4,5,6,7 # 错误 -- 赋值中出现了两个星号表达式 *(a,b), c = 'XY', 3 # 错误 -- 需要拆包的数值太少 *(*a,b), c = 'XY', 3 # a = [], b = 'XY', c = 3 (a,b), c = 'XY', 3 # a = 'X', b = 'Y', c = 3 *(a,b), c = 'XY', 3, 4 # a = 'XY', b = 3, c = 4 *(*a,b), c = 'XY', 3, 4 # a = ['XY'], b = 3, c = 4 (a,b), c = 'XY', 3, 4 # 错误 -- 拆包的值太多了
Manually derive the results of these expressions
Generalizing them is not difficult once you have a few basic rules. I'll do my best to explain with some examples. Since you mentioned "manually" evaluating these expressions, I would suggest some simple replacement rules. Basically, you'll find it easier to understand expressions where all iterables are formatted the same way.
For unpacking purposes only, the following substitutions are valid in rvalues (i.e. iterable objects):
'XY' -> ('X', 'Y') ['X', 'Y'] -> ('X', 'Y')
If you find a value is not unpacked, then you need to undo the substitution. (See below for further explanation.)
Also, when you see a "bare" comma, imagine there is a tuple at the top. Do this on the left and right sides (i.e. iterable and right iterable):
'X', 'Y' -> ('X', 'Y') a, b -> (a, b)
The above is the detailed content of How to Understand Complex Unpacking Expressions in Python?. For more information, please follow other related articles on the PHP Chinese website!
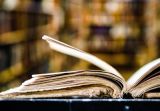
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
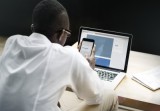
Python provides a variety of ways to download files from the Internet, which can be downloaded over HTTP using the urllib package or the requests library. This tutorial will explain how to use these libraries to download files from URLs from Python. requests library requests is one of the most popular libraries in Python. It allows sending HTTP/1.1 requests without manually adding query strings to URLs or form encoding of POST data. The requests library can perform many functions, including: Add form data Add multi-part file Access Python response data Make a request head
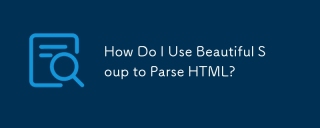
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
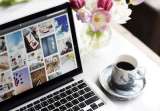
Dealing with noisy images is a common problem, especially with mobile phone or low-resolution camera photos. This tutorial explores image filtering techniques in Python using OpenCV to tackle this issue. Image Filtering: A Powerful Tool Image filter
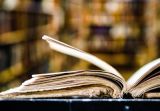
PDF files are popular for their cross-platform compatibility, with content and layout consistent across operating systems, reading devices and software. However, unlike Python processing plain text files, PDF files are binary files with more complex structures and contain elements such as fonts, colors, and images. Fortunately, it is not difficult to process PDF files with Python's external modules. This article will use the PyPDF2 module to demonstrate how to open a PDF file, print a page, and extract text. For the creation and editing of PDF files, please refer to another tutorial from me. Preparation The core lies in using external module PyPDF2. First, install it using pip: pip is P

This tutorial demonstrates how to leverage Redis caching to boost the performance of Python applications, specifically within a Django framework. We'll cover Redis installation, Django configuration, and performance comparisons to highlight the bene
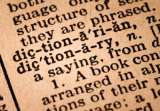
Natural language processing (NLP) is the automatic or semi-automatic processing of human language. NLP is closely related to linguistics and has links to research in cognitive science, psychology, physiology, and mathematics. In the computer science
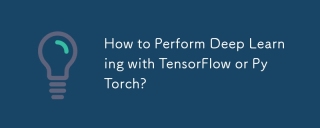
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
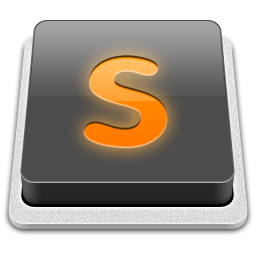
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
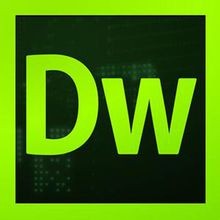
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
