


Interpolating Colors in a Color Space
In various artistic and design contexts, the need often arises to blend or interpolate different colors to create a desired shade. While digital color mixing is straightforward for emissive colors, such as those displayed on RGB screens, simulating the mixing of physical paints presents unique challenges.
Traditional paint mixing relies on absorption, where pigments selectively absorb specific wavelengths of light. When blue and yellow paints are mixed, the resulting color is determined by the absorption properties of each pigment. Blue paint absorbs red and green light, while yellow paint absorbs blue light. In a perfect scenario, this absorption process would result in a dark or muddy color, rather than a vibrant green.
However, in practice, physical pigments deviate from ideal absorption characteristics, leading to a wide range of possible outcomes when mixing colors. To address this complexity, researchers have developed color models that more accurately represent the behavior of physical paints. One such model is the subtractive color mixing model.
Subtractive Color Mixing
Subtractive color mixing is used to simulate the behavior of physical pigments, particularly when working with subtractive color systems such as paints, dyes, and inks. In this model, the result of mixing two colors is determined by subtracting the absorption factors of each pigment from a white light source.
For example, when mixing blue and yellow pigments, the blue pigment absorbs red and green light, while the yellow pigment absorbs blue light. The resulting color is a muddy green, which represents the remaining light that has not been absorbed by either pigment.
Interpolation in the Color Space
While subtractive color mixing is a realistic representation of physical paint mixing, it can be computationally complex to simulate. Therefore, alternative methods are often used to interpolate colors in a color space, such as RGB or HLS.
Interpolation in the RGB color space involves blending the individual color components (red, green, and blue) between two given colors. This approach is straightforward but can produce colors that do not accurately reflect physical paint mixing.
Interpolation in the HLS color space, on the other hand, involves blending the hue, lightness, and saturation components of two given colors. This method offers more flexibility and produces more intuitive results for mixing different colors, including intermediate shades of green when mixing blue and yellow.
Sample Implementation
The following Python code snippet demonstrates color interpolation using the HLS color space:
import colorsys def average_colors(rgb1, rgb2): h1, l1, s1 = colorsys.rgb_to_hls(rgb1[0]/255., rgb1[1]/255., rgb1[2]/255.) h2, l2, s2 = colorsys.rgb_to_hls(rgb2[0]/255., rgb2[1]/255., rgb2[2]/255.) s = 0.5 * (s1 + s2) l = 0.5 * (l1 + l2) x = cos(2*pi*h1) + cos(2*pi*h2) y = sin(2*pi*h1) + sin(2*pi*h2) if x != 0.0 or y != 0.0: h = atan2(y, x) / (2*pi) else: h = 0.0 s = 0.0 r, g, b = colorsys.hls_to_rgb(h, l, s) return (int(r*255.), int(g*255.), int(b*255.)) print(average_colors((255,255,0),(0,0,255))) print(average_colors((255,255,0),(0,255,255)))
This implementation demonstrates the interpolation of blue (0,0,255) and yellow (255,255,0) using the HLS color space, resulting in shades of green.
It is important to note that this method and other color interpolation techniques do not fully emulate physical paint mixing. However, they provide a convenient way to generate realistic-looking color transitions within a digital environment.
The above is the detailed content of How Can Color Interpolation in Different Color Spaces Accurately Simulate Physical Paint Mixing?. For more information, please follow other related articles on the PHP Chinese website!
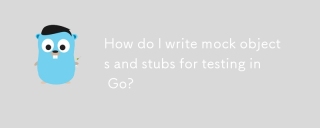
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
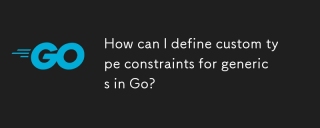
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
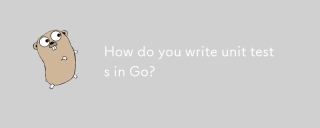
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
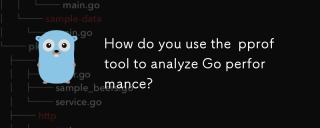
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
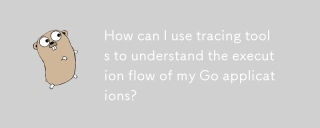
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
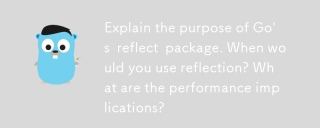
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
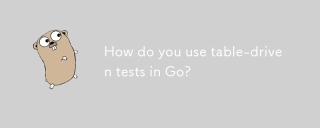
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
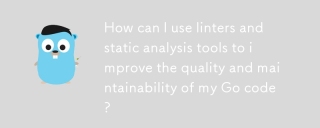
This article advocates for using linters and static analysis tools to enhance Go code quality. It details tool selection (e.g., golangci-lint, go vet), workflow integration (IDE, CI/CD), and effective interpretation of warnings/errors to improve cod


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
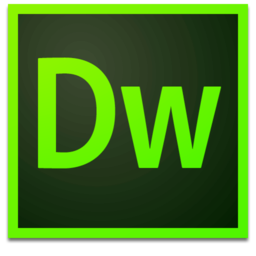
Dreamweaver Mac version
Visual web development tools
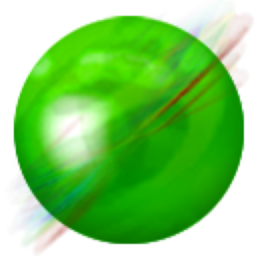
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
