Reading and Writing Non-UTF-8 Text Files in Go
Background
By default, the standard library in Go assumes that text files are encoded in UTF-8. However, there are scenarios where you may encounter text files encoded in different encodings, such as GBK.
Solution
To read and write non-UTF-8 text files in Go, you can use the following steps:
Reading Non-UTF-8 Files
-
Import the necessary package: import "golang.org/x/text/encoding/simplifiedchinese"
This package provides GB18030, GBK, and HZ-GB2312 encoding implementations. -
Create an io.Reader using transform.NewReader:
f, err := os.Open(filename) if err != nil { log.Fatal(err) } r := transform.NewReader(f, simplifiedchinese.GBK.NewDecoder())
Writing Non-UTF-8 Files
- Import the same package: import "golang.org/x/text/encoding/simplifiedchinese"
-
Create an io.Writer using transform.NewWriter:
f, err := os.Create(filename) if err != nil { log.Fatal(err) } w := transform.NewWriter(f, simplifiedchinese.GBK.NewEncoder())
Example
The following example shows how to read and write a GBK-encoded text file:
import ( "bufio" "fmt" "log" "os" "golang.org/x/text/encoding/simplifiedchinese" "golang.org/x/text/transform" ) func main() { const filename = "example_GBK_file" exampleWriteGBK(filename) exampleReadGBK(filename) } func exampleReadGBK(filename string) { f, err := os.Open(filename) if err != nil { log.Fatal(err) } r := transform.NewReader(f, simplifiedchinese.GBK.NewDecoder()) sc := bufio.NewScanner(r) for sc.Scan() { fmt.Printf("Read line: %s\n", sc.Bytes()) } if err := sc.Err(); err != nil { log.Fatal(err) } if err := f.Close(); err != nil { log.Fatal(err) } } func exampleWriteGBK(filename string) { f, err := os.Create(filename) if err != nil { log.Fatal(err) } w := transform.NewWriter(f, simplifiedchinese.GBK.NewEncoder()) // Write some text from the Wikipedia GBK page that includes Chinese _, err = fmt.Fprintln(w, `In 1995, China National Information Technology Standardization Technical Committee set down the Chinese Internal Code Specification (Chinese: 汉字内码扩展规范(GBK); pinyin: Hànzì Nèimǎ Kuòzhǎn Guīfàn (GBK)), Version 1.0, known as GBK 1.0, which is a slight extension of Codepage 936. The newly added 95 characters were not found in GB 13000.1-1993, and were provisionally assigned Unicode PUA code points.`) if err != nil { log.Fatal(err) } if err := f.Close(); err != nil { log.Fatal(err) } }
This code opens a GBK-encoded text file, reads its contents, and writes them to another GBK-encoded text file.
The above is the detailed content of How to Read and Write Non-UTF-8 Encoded Text Files in Go?. For more information, please follow other related articles on the PHP Chinese website!
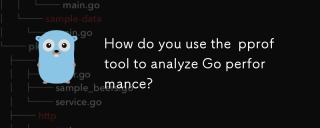
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
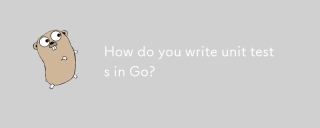
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
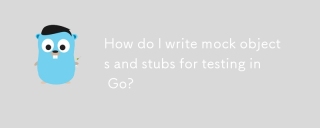
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
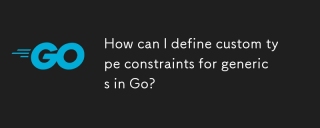
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
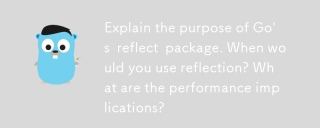
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
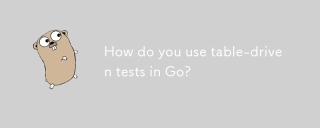
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
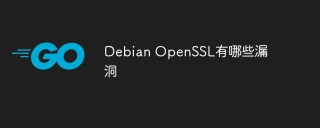
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
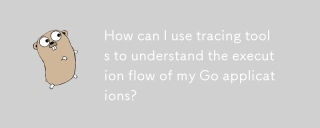
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
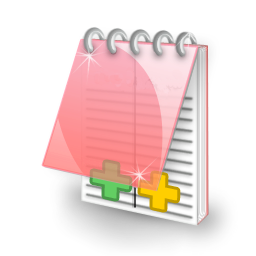
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool