


Calculating Time Difference between LocalDateTime Instances
In Java 8, calculating the difference between two LocalDateTime instances can be achieved using the Period and Duration classes. However, your code currently faces some inaccuracies.
Original Code Analysis
Your code uses the Period class to calculate the years, months, and days between the two LocalDateTime instances. For the time difference, you convert the instances to LocalDateTime with only the time components and calculate the difference using Duration.
The issue arises because your time calculation assumes that the fromDateTime is before the toDateTime. If the dates are out of order, negative numbers will result, leading to incorrect time values.
Improved Algorithm Using ChronoUnit
A more robust approach involves using the ChronoUnit enum to calculate the time difference. Here's the improved portion of your code:
long hours = ChronoUnit.HOURS.between(fromDateTime, toDateTime); long minutes = ChronoUnit.MINUTES.between(fromDateTime, toDateTime);
ChronoUnit provides a set of predefined units that accurately calculate the difference between two DateTime instances. By specifying the unit as HOURS or MINUTES, the time difference can be obtained in the desired unit without the need for manual conversions or handling of negative values.
Updated Example
Using the ChronoUnit method, the code will then correctly calculate the difference between the input LocalDateTime instances, regardless of their order:
LocalDateTime toDateTime = LocalDateTime.of(2014, 9, 9, 19, 46, 45); LocalDateTime fromDateTime = LocalDateTime.of(1984, 12, 16, 7, 45, 55); long years = Period.between(fromDateTime.toLocalDate(), toDateTime.toLocalDate()).getYears(); long months = Period.between(fromDateTime.toLocalDate(), toDateTime.toLocalDate()).getMonths(); long days = Period.between(fromDateTime.toLocalDate(), toDateTime.toLocalDate()).getDays(); long hours = ChronoUnit.HOURS.between(fromDateTime, toDateTime); long minutes = ChronoUnit.MINUTES.between(fromDateTime, toDateTime); System.out.println(years + " years " + months + " months " + days + " days " + hours + " hours " + minutes + " minutes.");
This code correctly calculates the time difference as "29 years 8 months 24 days 12 hours 0 minutes."
The above is the detailed content of How to Accurately Calculate the Time Difference Between Two Java LocalDateTime Instances?. For more information, please follow other related articles on the PHP Chinese website!
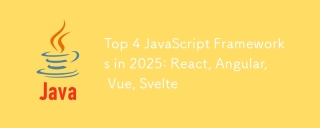
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
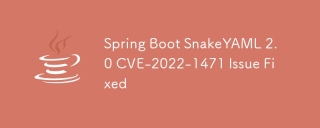
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
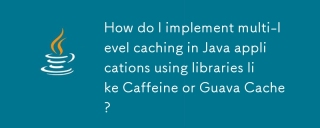
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
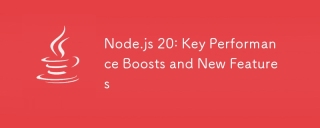
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
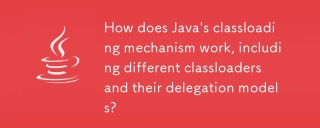
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
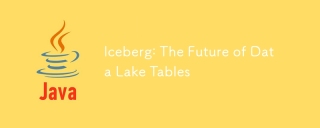
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
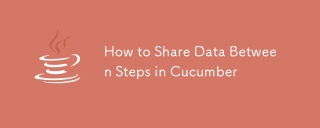
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
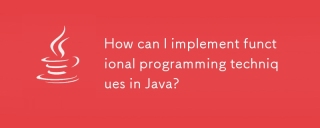
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
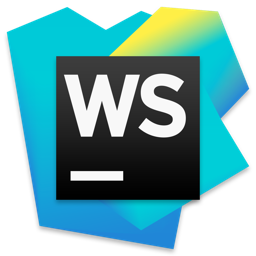
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
