Custom Exception Handling in Python
Creating custom exception classes in Python allows developers to handle specific errors gracefully and provide additional information about the cause of the issue.
Custom Exception Declaration
In modern Python, it's recommended to define custom exceptions by extending the Exception class:
class MyException(Exception): pass
This approach aligns with the standard for other exception classes and ensures that any additional attributes included in the exception will be accessible to tools handling the exception.
Custom Exception Initialization
To store additional data in the exception, override the __init__ method and pass the relevant information as parameters:
class ValidationError(Exception): def __init__(self, message, errors): super().__init__(message) self.errors = errors
This way, you can access the extra data via e.errors when handling the exception.
Avoidance of BaseException.message
In Python 2.5, the BaseException class had a special attribute message that stored the exception message. However, this attribute has been deprecated in Python 2.6 and should not be used. Instead, the message attribute should be added to the custom exception class explicitly.
Magic Arguments
The args argument in the Exception constructor is used to store the arguments passed to the exception during initialization. However, it's not recommended to use args for storing additional data, as it may be confusing and inconsistent with other exception classes.
Overriding Methods
While overriding __init__ and __str__ (or __unicode__ and __repr__ in Python 2) is commonly suggested, it is not necessary for simple custom exceptions. The provided examples demonstrate how to create custom exceptions without overriding these methods.
The above is the detailed content of How Can I Create and Handle Custom Exceptions in Python?. For more information, please follow other related articles on the PHP Chinese website!
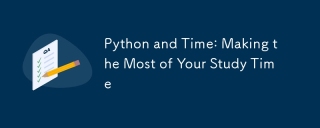
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
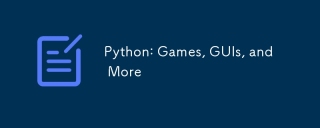
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
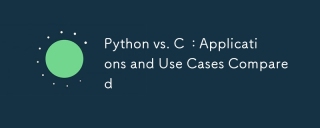
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
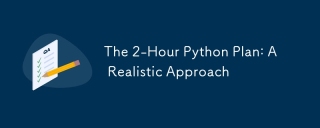
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
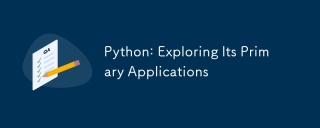
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
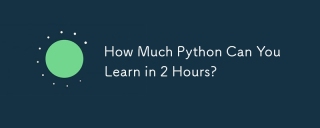
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
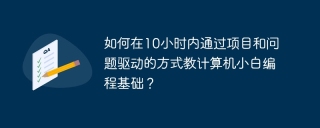
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
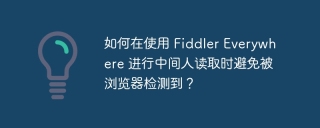
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
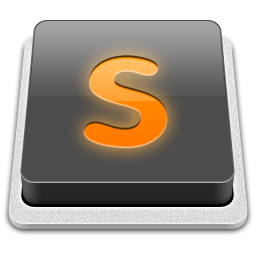
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
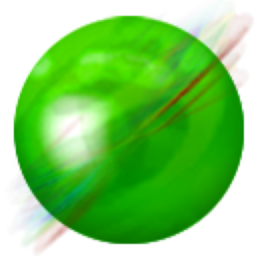
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment