


Understanding ""declared and not used" Error: Shadowing and Scoping in Go
The error message "declared and not used" in Go often occurs when a variable is declared but not utilized in the code. This can happen due to misunderstandings surrounding the := and = assignment operators.
In the provided example, the error points to the z variable within the for-loop:
func Sqrt(x float64) float64 { z := float64(x) for i := 0; i <p>The := operator, used within the for-loop, declares a new variable named z that shadows the outer z. This means that the loop's z variable is distinct from the one declared outside of it. As a result, the loop's z variable is declared but not used, triggering the error.</p><p>To resolve this issue, we should replace the := with a simple = assignment operator in the for-loop:</p><pre class="brush:php;toolbar:false">func Sqrt(x float64) float64 { z := x for i := 0; i <p>Now, both instances of z refer to the same variable, eliminating the shadowing issue and allowing the code to compile successfully.</p>
The above is the detailed content of Why Does My Go Code Show a \'declared and not used\' Error, and How Can I Fix Shadowing Issues?. For more information, please follow other related articles on the PHP Chinese website!
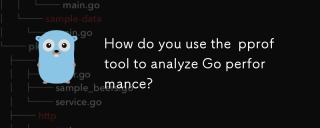
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
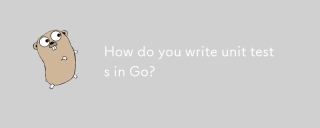
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
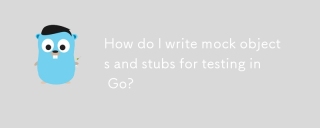
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
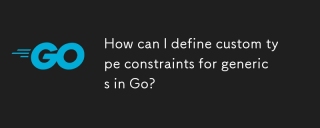
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
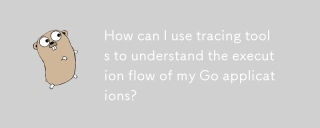
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
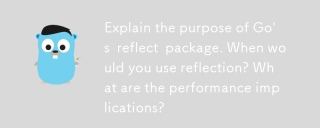
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
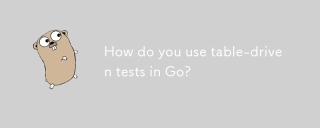
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
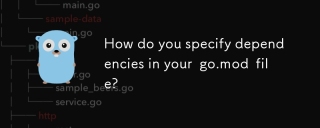
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
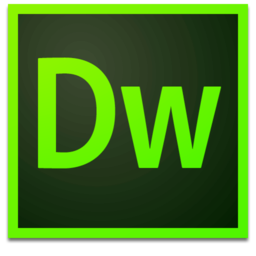
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
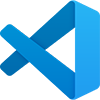
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
