


Comparing Error Messages in Go
When handling errors in Java, developers often rely on the Exception.GetMessage() method to extract error descriptions. Golang, however, has no built-in method to retrieve such messages.
To address this, Go developers employ a custom approach to comparing error messages. Instead of storing messages within the error object, they create package-level error variables that encapsulate specific error codes or descriptions:
var errExample = errors.New("this is an example")
When returning errors, developers assign these variables to indicate the specific type of error that occurred. The following snippet demonstrates this approach:
if some_err := some_package.DoSomething(); some_err != nil { if some_err == errExample { // handle it } }
In this case, the if statement checks if the error returned by DoSomething() is identical to the errExample variable. If so, the error is handled accordingly.
To extend the reach of these error variables, developers can export them outside the package using the export keyword:
var ErrExample = errors.New("this is an example")
This allows code in different packages to compare against the exported error variable:
if err == somepackage.ErrExample { // handle it }
By following this approach, developers can effectively compare error messages in Golang, even though the language lacks a dedicated GetMessage() method.
Additional Considerations:
It's crucial to avoid comparing error messages against the string returned by the error.Error() method. This can make code brittle, as error messages are subject to change over time. Instead, it is recommended to use the established error variables to ensure consistency in error handling.
The above is the detailed content of How Do Go Developers Compare Error Messages Without a `GetMessage()` Method?. For more information, please follow other related articles on the PHP Chinese website!
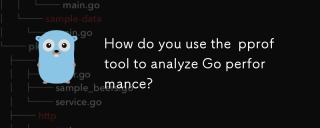
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
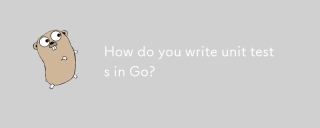
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
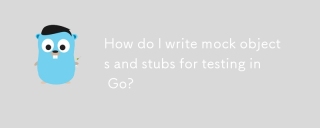
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
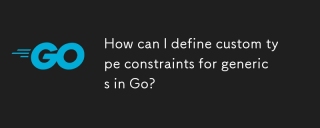
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
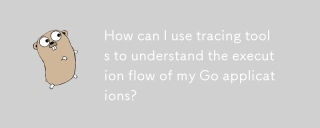
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
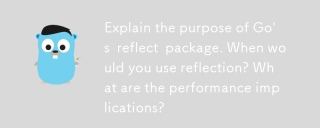
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
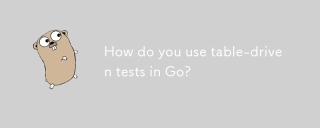
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
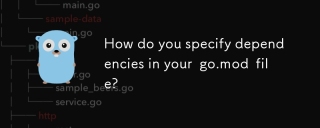
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
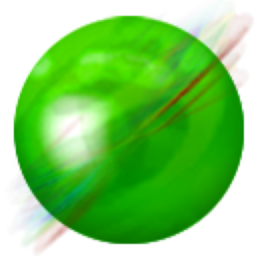
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
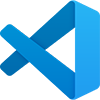
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
