


LLM Parallel Processing in Practice: Key Techniques for Performance Enhancement
Key Points
- Master parallel processing strategies in LLM applications
- Implement efficient batch processing mechanisms
- Build scalable document processing systems
- Optimize system performance and resource utilization
Parallel Processing Use Cases
In LLM applications, parallel processing is particularly suitable for:
- Batch document processing
- Multi-model parallel inference
- Large-scale data analysis
- Real-time stream processing
Batch Processing Strategy Design
1. Basic Architecture
from typing import List, Dict, Any from dataclasses import dataclass import asyncio from langchain.chat_models import ChatOpenAI from langchain.callbacks import AsyncCallbackHandler @dataclass class BatchConfig: """Batch processing configuration""" batch_size: int = 5 max_concurrent_tasks: int = 3 timeout_seconds: int = 30 retry_attempts: int = 2 class BatchProcessor: def __init__(self, config: BatchConfig): self.config = config self.llm = ChatOpenAI( temperature=0, request_timeout=config.timeout_seconds ) self.semaphore = asyncio.Semaphore( config.max_concurrent_tasks ) async def process_batch( self, items: List[Any] ) -> List[Dict]: """Main batch processing function""" batches = self._create_batches(items) results = [] for batch in batches: batch_results = await self._process_batch_with_semaphore( batch ) results.extend(batch_results) return results
2. Asynchronous Processing Implementation
class AsyncBatchProcessor(BatchProcessor): async def _process_single_item( self, item: Any ) -> Dict: """Process single item""" async with self.semaphore: for attempt in range(self.config.retry_attempts): try: return await self._execute_processing(item) except Exception as e: if attempt == self.config.retry_attempts - 1: return self._create_error_response(item, e) await asyncio.sleep(2 ** attempt) async def _execute_processing( self, item: Any ) -> Dict: """Execute specific processing logic""" task = asyncio.create_task( self.llm.agenerate([item]) ) try: result = await asyncio.wait_for( task, timeout=self.config.timeout_seconds ) return { "status": "success", "input": item, "result": result } except asyncio.TimeoutError: task.cancel() raise
Real-world Case: Batch Document Processing System
1. System Architecture
class DocumentBatchProcessor: def __init__(self): self.config = BatchConfig( batch_size=10, max_concurrent_tasks=5 ) self.processor = AsyncBatchProcessor(self.config) self.results_manager = ResultsManager() async def process_documents( self, documents: List[str] ) -> Dict: """Process document batches""" try: preprocessed = await self._preprocess_documents( documents ) results = await self.processor.process_batch( preprocessed ) return await self.results_manager.merge_results( results ) except Exception as e: return self._handle_batch_error(e, documents)
2. Resource Control Mechanism
class ResourceController: def __init__(self): self.token_limit = 4096 self.request_limit = 100 self._request_count = 0 self._token_count = 0 self._reset_time = None async def check_limits(self) -> bool: """Check resource limits""" await self._update_counters() return ( self._request_count <h3> 3. Results Merging Strategy </h3> <pre class="brush:php;toolbar:false">class ResultsManager: def __init__(self): self.merge_strategies = { "text": self._merge_text_results, "embeddings": self._merge_embedding_results, "classifications": self._merge_classification_results } async def merge_results( self, results: List[Dict] ) -> Dict: """Merge processing results""" merged = { "success_count": 0, "error_count": 0, "results": [] } for result in results: if result["status"] == "success": merged["success_count"] += 1 merged["results"].append( await self._process_result(result) ) else: merged["error_count"] += 1 return merged
Performance Optimization Guide
1. Memory Management
class MemoryManager: def __init__(self, max_memory_mb: int = 1024): self.max_memory = max_memory_mb * 1024 * 1024 self.current_usage = 0 async def monitor_memory(self): """Monitor memory usage""" import psutil process = psutil.Process() memory_info = process.memory_info() if memory_info.rss > self.max_memory: await self._trigger_memory_cleanup() async def _trigger_memory_cleanup(self): """Trigger memory cleanup""" import gc gc.collect()
2. Performance Monitoring
class PerformanceMonitor: def __init__(self): self.metrics = { "processing_times": [], "error_rates": [], "throughput": [] } async def record_metrics( self, batch_size: int, duration: float, errors: int ): """Record performance metrics""" self.metrics["processing_times"].append(duration) self.metrics["error_rates"].append(errors / batch_size) self.metrics["throughput"].append( batch_size / duration )
Best Practices
-
Batch Processing Optimization
- Dynamically adjust batch size based on system resources
- Implement intelligent retry mechanisms
- Monitor and optimize memory usage
-
Concurrency Control
- Use semaphores to limit concurrency
- Implement request rate limiting
- Set reasonable timeout values
-
Error Handling
- Implement tiered error handling
- Record detailed error information
- Provide graceful degradation options
Performance Tuning Points
-
System Level
- Monitor system resource usage
- Optimize memory management
- Implement load balancing
-
Application Level
- Optimize batch processing strategies
- Adjust concurrency parameters
- Implement caching mechanisms
Summary
Parallel processing is crucial for building high-performance LLM applications. Key takeaways:
- Design efficient batch processing strategies
- Implement robust resource management
- Monitor and optimize system performance
- Handle errors gracefully
The above is the detailed content of LLM Parallel Processing in Practice: Key Techniques for Performance Enhancement. For more information, please follow other related articles on the PHP Chinese website!
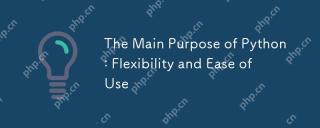
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
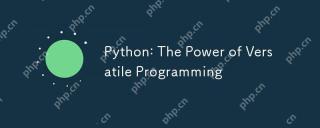
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
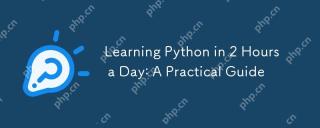
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.
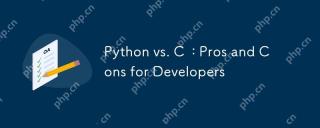
Python is suitable for rapid development and data processing, while C is suitable for high performance and underlying control. 1) Python is easy to use, with concise syntax, and is suitable for data science and web development. 2) C has high performance and accurate control, and is often used in gaming and system programming.
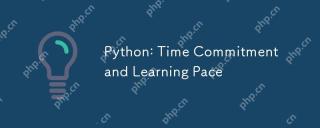
The time required to learn Python varies from person to person, mainly influenced by previous programming experience, learning motivation, learning resources and methods, and learning rhythm. Set realistic learning goals and learn best through practical projects.
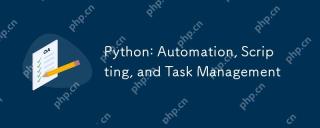
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
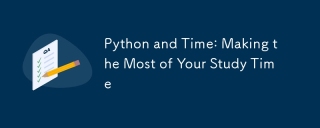
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
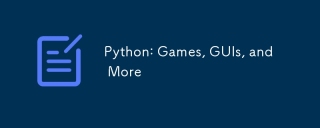
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!
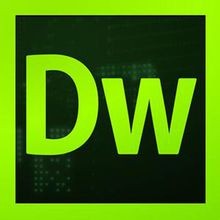
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
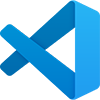
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft