Unmarshalling XML Attributes with Unknown Quantities Using Golang
In Golang, unmarshalling XML involves parsing XML data into a struct, allowing for convenient data manipulation and retrieval. However, certain scenarios require the handling of unexpected XML attributes, where the attribute names and values may vary across instances.
The encoding/xml package provides support for unmarshalling XML elements with dynamic attributes through the xml:",any,attr" annotation. This feature enables the collection of all attributes into a slice of xml.Attr within the struct.
To illustrate this capability, consider the following code:
package main import ( "encoding/xml" "fmt" ) func main() { var v struct { Attributes []xml.Attr `xml:",any,attr"` } data := `<tag attr1="VALUE1" attr2="VALUE2"></tag>` err := xml.Unmarshal([]byte(data), &v) if err != nil { panic(err) } fmt.Println(v.Attributes) }
When executed, this code will output the following:
[{ATTR1 VALUE1} {ATTR2 VALUE2}]
Each entry in the Attributes slice represents an attribute, consisting of its name (e.g., "ATTR1") and value (e.g., "VALUE1").
This enhancement empowers developers to work with XML documents containing unknown or dynamic attributes, making Go an even more versatile tool for XML processing.
The above is the detailed content of How to Handle Unknown XML Attributes During Unmarshalling in Go?. For more information, please follow other related articles on the PHP Chinese website!
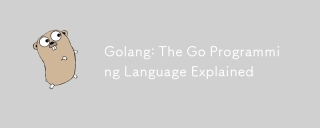
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
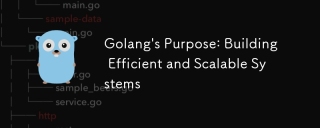
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
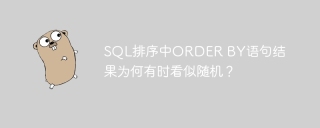
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
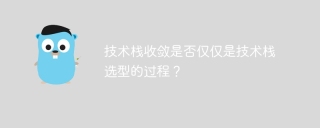
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
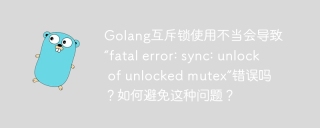
Golang ...
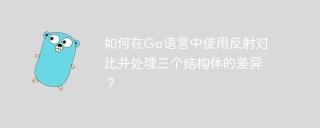
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...
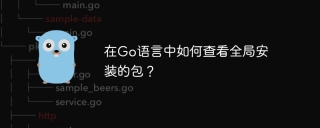
How to view globally installed packages in Go? In the process of developing with Go language, go often uses...
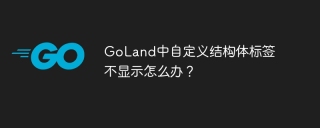
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
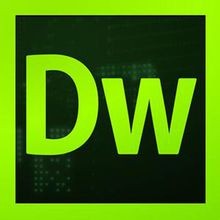
Dreamweaver CS6
Visual web development tools
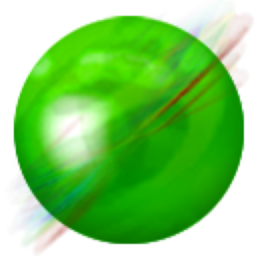
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
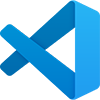
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment