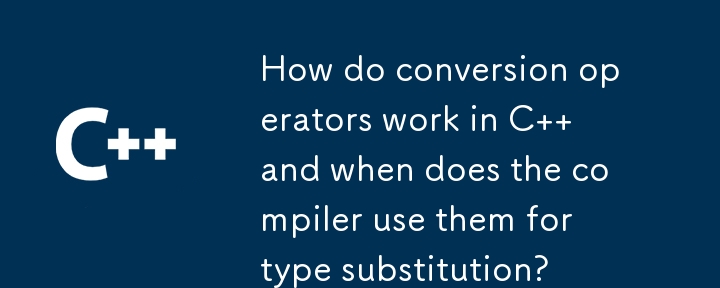
Conversion Operators in C
Question: How do conversion operators work in C , and when does the compiler attempt to substitute the type defined after the conversion operator?
Answer: Conversion operators in C facilitate type conversions between classes or from classes to fundamental types. The compiler substitutes the type specified after the conversion operator in the following situations:
Conversion during Argument Passing:
- During argument passing, the rules for copy initialization apply, considering any conversion function regardless of whether it converts to a reference or not.
- Example: void f(B); int main() { f(A()); }
Conversion to Reference:
- In conditional operators, conversion to a reference type is possible if the type being converted to is an lvalue.
- Example: B b; 0 ? b : A();
Conversion to Function Pointers:
- Conversion functions to function pointers or references can be used when a function call is made.
- Example: typedef void (*fPtr)(int); void foo(int a); struct test { operator fPtr() { return foo; } };
Conversion to Non-Class Types:
- User-defined conversion functions can be used for implicit conversions involving non-class types.
- Example: struct test { operator bool() { return true; } };
Conversion Function Templates:
- Templates can be used to create conversion functions that allow a type to be convertible to any pointer type.
- Example: struct test { template operator T*() { return 0; } };
-
Caution: These should be used cautiously as they can lead to ambiguous conversions.
The above is the detailed content of How do conversion operators work in C and when does the compiler use them for type substitution?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn