Unit Testing Command Line Flags in Go
In Go, defining custom flags for command-line applications requires a thorough testing process to ensure their correctness. One specific scenario involves verifying that a particular flag's value falls within a predefined enumeration.
Code to Test
<code class="go">var formatType string const ( text = "text" json = "json" hash = "hash" ) func init() { const ( defaultFormat = "text" formatUsage = "desired output format" ) flag.StringVar(&formatType, "format", defaultFormat, formatUsage) flag.StringVar(&formatType, "f", defaultFormat, formatUsage+" (shorthand)") } func main() { flag.Parse() }</code>
Testing Approach
To unit test the desired behavior, we can harness the power of the flag.Var function, which allows us to define custom types and validation rules for flags.
<code class="go">type formatType string func (f *formatType) String() string { return fmt.Sprint(*f) } func (f *formatType) Set(value string) error { if len(*f) > 0 && *f != "text" { return errors.New("format flag already set") } if value != "text" && value != "json" && value != "hash" { return errors.New("Invalid Format Type") } *f = formatType(value) return nil }</code>
In this custom type implementation:
- String() returns the current value of the flag in string form.
- Set() updates the flag's value and ensures that it falls within the permitted enumeration.
By employing this custom formatType flag, the testing process can now validate the flag's behavior when set to various values.
Example Testing
<code class="go">package main import "testing" func TestFormatType(t *testing.T) { tests := []struct { args []string expect string }{ {[]string{"-format", "text"}, "text"}, {[]string{"-f", "json"}, "json"}, {[]string{"-format", "foo"}, "Invalid Format Type"}, } for _, test := range tests { t.Run(test.args[0], func(t *testing.T) { flag.Parse() if typeFlag != test.expect { t.Errorf("Expected %s got %s", test.expect, typeFlag) } }) } }</code>
In this example:
- TestFormatType iterates over a list of tests.
- Each test sets up command-line arguments, parses the flags, and compares the resulting typeFlag value with the expected results.
- If any test fails, it reports an error.
The above is the detailed content of How to Unit Test Command Line Flag Enumeration Validation in Go?. For more information, please follow other related articles on the PHP Chinese website!
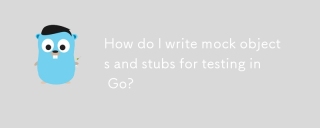
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
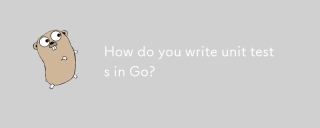
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
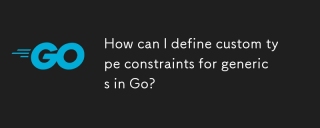
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
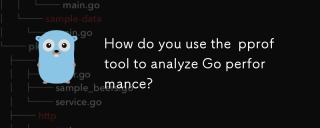
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
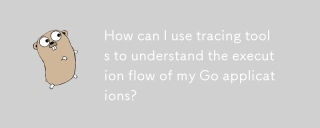
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
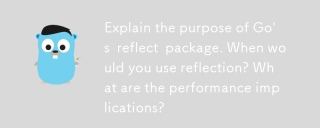
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
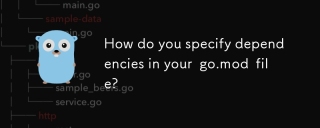
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
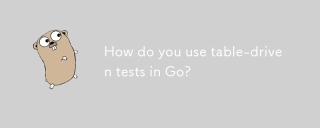
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
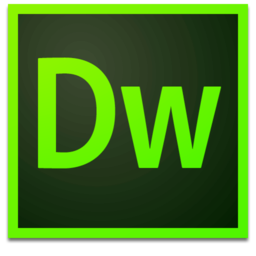
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
