


How can I create a realistic atmosphere around a rendered Earth in Three.js?
How can I render an 'atmosphere' over a rendering of the Earth in Three.js?
In Three.js, you can simulate an atmosphere using the following techniques:
- Atmospheric Scattering: This technique simulates the scattering of light in the atmosphere, creating a realistic glow around objects. To achieve this, you can use the ShaderMaterial and write a custom shader that implements atmospheric scattering.
Here is an example of a shader that implements atmospheric scattering:
// Vertex shader varying vec3 vWorldPosition; void main() { vWorldPosition = (modelMatrix * vec4(position, 1.0)).xyz; gl_Position = projectionMatrix * modelViewMatrix * vec4(position, 1.0); } // Fragment shader uniform vec3 cameraPosition; uniform float scattering; uniform float extinction; uniform float turbidity; varying vec3 vWorldPosition; void main() { vec3 rayDirection = normalize(vWorldPosition - cameraPosition); vec3 attenuation = exp(-scattering * extinction * rayDirection.y * vWorldPosition.y); vec3 scatteringColor = attenuation * vec3(0.2, 0.5, 1.0); vec3 extinctionColor = vec3(0.0, 0.0, 0.0); gl_FragColor = vec4(scatteringColor + extinctionColor, 1.0); }
- Vertex Displacement: This technique displaces the vertices of objects to create a shimmering effect, simulating the effects of the atmosphere. To do this, you can use the VertexDisplacementShader and write a custom shader that distorts the vertices.
Here is an example of a shader that implements vertex displacement:
// Vertex shader uniform float time; uniform float amplitude; uniform float frequency; varying vec3 vWorldPosition; void main() { vec3 noise = vec3(perlinNoise(vWorldPosition * frequency)); vec3 displacement = noise * amplitude; vWorldPosition = (modelMatrix * vec4(position + displacement, 1.0)).xyz; gl_Position = projectionMatrix * modelViewMatrix * vec4(position, 1.0); } // Fragment shader void main() { gl_FragColor = vec4(0.0, 0.0, 0.0, 1.0); }
By combining these techniques, you can create a realistic representation of an atmosphere in Three.js.
Here are some additional tips for creating an atmospheric effect in Three.js:
- Use a gradient texture to create a smooth transition between the atmosphere and the sky.
- Add a slight haze to the atmosphere to create a more realistic scattering effect.
- Adjust the parameters of the shaders to fine-tune the appearance of the atmosphere.
The above is the detailed content of How can I create a realistic atmosphere around a rendered Earth in Three.js?. For more information, please follow other related articles on the PHP Chinese website!
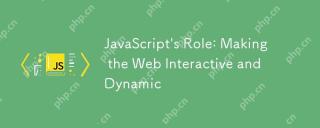
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
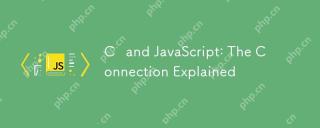
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
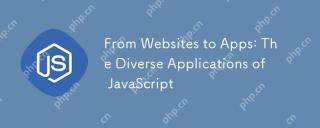
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
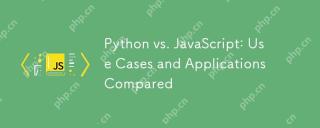
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
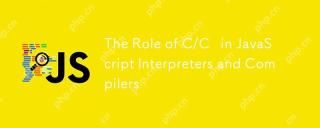
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
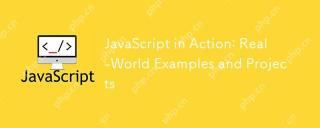
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
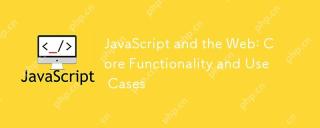
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
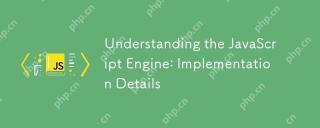
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
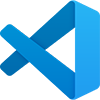
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
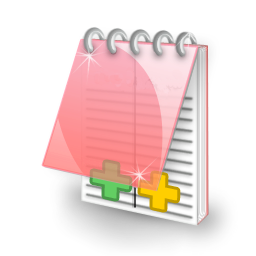
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
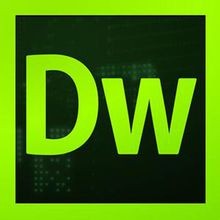
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
