


Simple Digit Recognition OCR in OpenCV-Python
Question 1
The letter_recognition.data file is a dataset that contains 20,000 samples of handwritten letters, with each letter represented by 16 features. To build a similar file from your own dataset, you can follow these steps:
- Extract the pixel values from each letter image.
- Store the pixel values in an array.
- Create a corresponding array containing the labels for each letter.
- Save both arrays to a text file using NumPy's savetxt() function.
Question 2
results.reval() is the output array returned by the find_nearest() function of OpenCV's KNearest class. It contains the predicted labels for the given samples.
Question 3
To write a simple digit recognition tool using the letter_recognition.data file, you can follow these steps:
Training:
- Load the letter_recognition.data file.
- Extract the samples and responses from the file.
- Create an instance of the KNearest classifier.
- Train the classifier using the samples and responses.
Testing:
- Load the image containing the digits to be recognized.
- Preprocess the image to extract individual digits.
- Extract pixel values from each digit and store them in an array.
- Use the trained classifier to predict the labels for each digit.
- Display the recognized digits on the image.
Below is an example code that demonstrates the training and testing process:
import numpy as np import cv2 # Load training data samples = np.loadtxt('letter_recognition.data', np.float32, delimiter=',', converters={0: lambda ch: ord(ch) - ord('A')}) responses = samples[:, 0] samples = samples[:, 1:] # Create KNearest classifier model = cv2.KNearest() # Train the classifier model.train(samples, responses) # Load test image test_image = cv2.imread('test_image.png') # Preprocess the image gray = cv2.cvtColor(test_image, cv2.COLOR_BGR2GRAY) thresh = cv2.adaptiveThreshold(gray, 255, 1, 1, 11, 2) # Extract digits contours, hierarchy = cv2.findContours(thresh, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE) digits = [] for cnt in contours: if cv2.contourArea(cnt) > 50: [x, y, w, h] = cv2.boundingRect(cnt) roi = thresh[y:y + h, x:x + w] roismall = cv2.resize(roi, (10, 10)) digits.append(roismall) # Predict labels for digits results = model.find_nearest(np.array(digits), 10) labels = [chr(ch + ord('A')) for ch in results[0]] # Display recognized digits on the image for i, label in enumerate(labels): cv2.putText(test_image, str(label), (digits[i][0], digits[i][1]), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0)) cv2.imshow('Recognized Digits', test_image) cv2.waitKey(0)
By following these steps and leveraging the KNearest classifier in OpenCV, you can create a basic digit recognition tool that can be further improved upon for more complex digit recognition tasks.
The above is the detailed content of How to Implement Simple Digit Recognition in OpenCV-Python using the letter_recognition.data Dataset?. For more information, please follow other related articles on the PHP Chinese website!
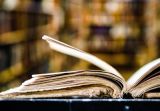
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
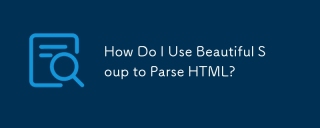
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
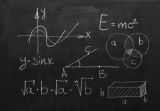
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
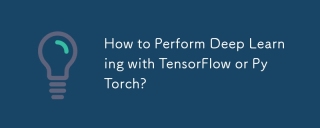
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
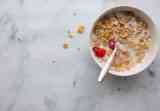
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
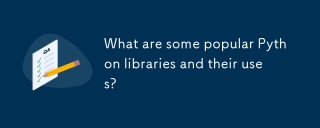
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
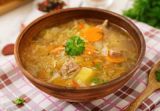
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
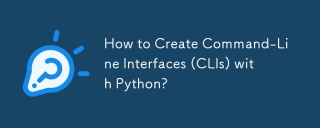
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
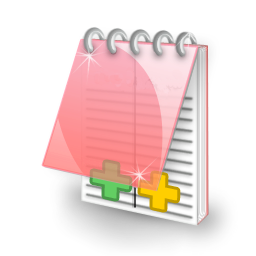
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
