Handling Panics in Go Routines
Go routines provide concurrency in Go programs, but panics within these routines can pose challenges. To recover from panics, the recover() function is typically employed. However, there are instances where recover() fails to operate as expected within goroutines.
Understanding the Failure of Recovery in Go Routines
Consider the following code block:
func main() { done := make(chan int64) defer fmt.Println("Graceful End of program") defer func() { r := recover() if _, ok := r.(error); ok { fmt.Println("Recovered") } }() go handle(done) for { select{ case <p>This code attempts to recover from a panic in a goroutine using a deferred function in the main function. However, it fails to recover the panic. Conversely, the following code block operates as expected:</p><pre class="brush:php;toolbar:false">func main() { done := make(chan int64) defer fmt.Println("Graceful End of program") defer func() { r := recover() if _, ok := r.(error); ok { fmt.Println("Recovered") } }() handle(done) for { select{ case <p><strong>Resolving the Recovery Issue</strong></p><p>The key to understanding this behavior lies in the nature of goroutines. recover() can only recover from panics that occur within the same goroutine where it is called. From the Go blog:</p><blockquote>The process continues up the stack until all functions in the current goroutine have returned, at which point the program crashes</blockquote><p>To handle panics in goroutines, one needs to use a deferred recovery function within the goroutine itself.</p><pre class="brush:php;toolbar:false">func handle(done chan int64) { defer func() { if r := recover(); r != nil { if _, ok := r.(error); ok { fmt.Println("Recovered in goroutine") } } }() var a *int64 a = nil fmt.Println(*a) done <p>By placing the recovery function within the goroutine, one can intercept panics and handle them appropriately.</p>
The above is the detailed content of Why Does `recover()` Fail to Handle Panics in Go Routines?. For more information, please follow other related articles on the PHP Chinese website!
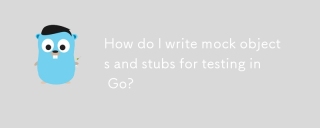
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
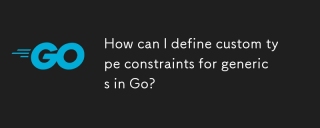
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
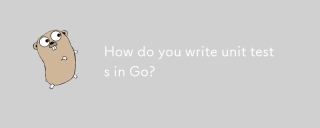
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
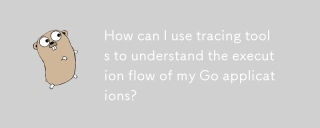
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
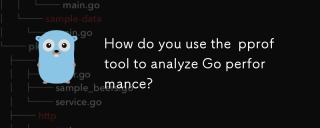
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
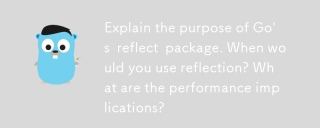
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
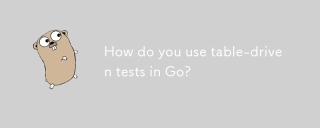
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
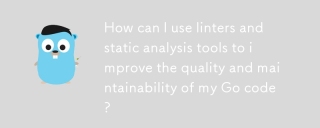
This article advocates for using linters and static analysis tools to enhance Go code quality. It details tool selection (e.g., golangci-lint, go vet), workflow integration (IDE, CI/CD), and effective interpretation of warnings/errors to improve cod


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
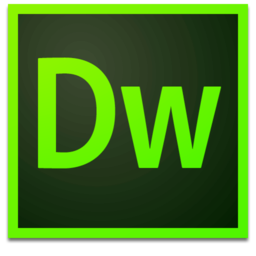
Dreamweaver Mac version
Visual web development tools
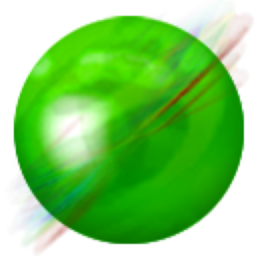
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
