What is the Comparable Interface Called?
In Go, there is no explicit comparable interface. To compare values of different types, you can use the Less function.
Here's how you can implement a Less function:
func Less(a, b interface{}) bool { switch a.(type) { case int: if ai, ok := a.(int); ok { if bi, ok := b.(int); ok { return ai <p>You can use the Less function to compare values in a linked list and insert new elements in a sorted manner:</p><pre class="brush:php;toolbar:false">func Insert(val interface{}, l *list.List) *list.Element { e := l.Front() if e == nil { return l.PushFront(val) } for ; e != nil; e = e.Next() { if Less(val, e.Value) { return l.InsertBefore(val, e) } } return l.PushBack(val) }
The above is the detailed content of How Can I Compare Values of Different Types in Go?. For more information, please follow other related articles on the PHP Chinese website!
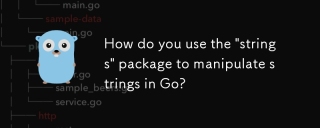
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
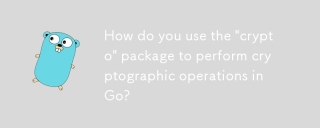
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
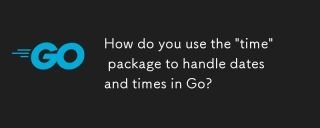
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
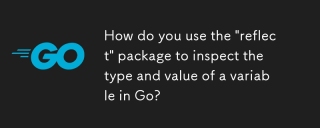
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.
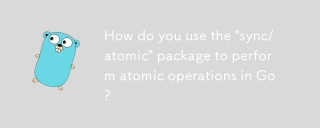
The article discusses using Go's "sync/atomic" package for atomic operations in concurrent programming, detailing its benefits like preventing race conditions and improving performance.
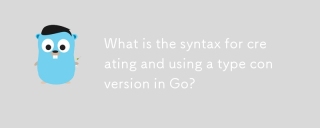
The article discusses type conversions in Go, including syntax, safe conversion practices, common pitfalls, and learning resources. It emphasizes explicit type conversion and error handling.[159 characters]
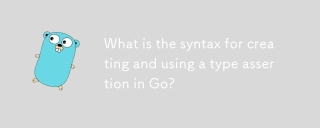
The article discusses type assertions in Go, focusing on syntax, potential errors like panics and incorrect types, safe handling methods, and performance implications.
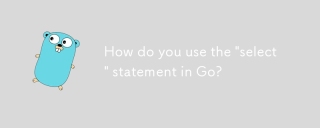
The article explains the use of the "select" statement in Go for handling multiple channel operations, its differences from the "switch" statement, and common use cases like handling multiple channels, implementing timeouts, non-b


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
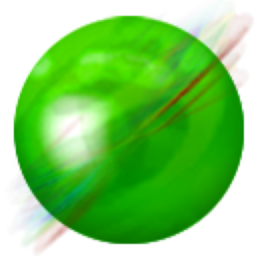
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
