In Golang CGo, Converting Union Field to Go Type
In Go's CGo, a C union is represented as an array of bytes, with the size determined by the union's largest member. This enables direct access to the union's contents. However, converting a union field to a Go type requires pointer operations.
Pointers and Union Interpretation
Consider the following C struct containing a union:
<code class="C">struct _GNetSnmpVarBind { guint32 *oid; /* name of the variable */ gsize oid_len; /* length of the name */ GNetSnmpVarBindType type; /* variable type / exception */ union { gint32 i32; /* 32 bit signed */ guint32 ui32; /* 32 bit unsigned */ gint64 i64; /* 64 bit signed */ guint64 ui64; /* 64 bit unsigned */ guint8 *ui8v; /* 8 bit unsigned vector */ guint32 *ui32v; /* 32 bit unsigned vector */ } value; /* value of the variable */ gsize value_len; /* length of a vector in bytes */ };</code>
To access the ui32v field in Go, one could attempt to convert the byte array to a pointer using:
<code class="go">func union_to_guint32_ptr(cbytes [8]byte) (result *_Ctype_guint32) { buf := bytes.NewBuffer(cbytes[:]) var ptr uint64 if err := binary.Read(buf, binary.LittleEndian, &ptr); err == nil { return (*_Ctype_guint32)(unsafe.Pointer(ptr)) } return nil }</code>
However, this approach fails due to type conversion issues. To resolve this, we can directly use the address of the byte array as the pointer:
<code class="go">guint32_star := *(**C.guint32)(unsafe.Pointer(&data.value[0]))</code>
This operation extracts the address of the first element in the byte array, effectively obtaining the address of the union itself. By manipulating the pointer type using unsafe.Pointer, we reinterpret the memory as a pointer to the desired type, (*C.guint32). Finally, dereferencing the result gives us access to the union member's contents.
By understanding the representation and manipulation of unions in CGo, developers can effectively work with complex C data structures in their Go programs.
The above is the detailed content of How do you convert a C union field to a Go type in CGo?. For more information, please follow other related articles on the PHP Chinese website!
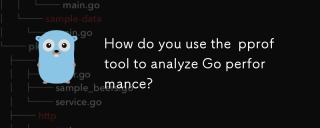
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
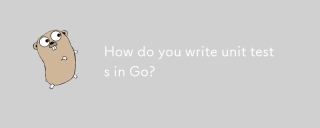
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
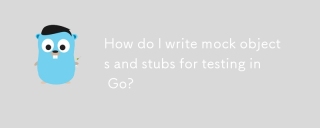
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
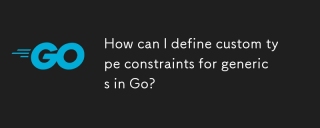
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
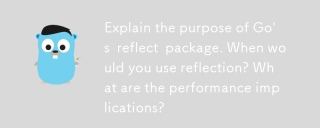
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
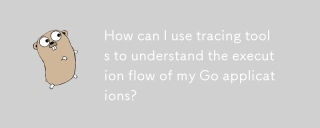
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
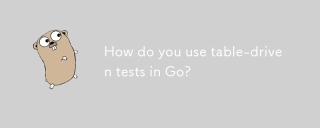
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
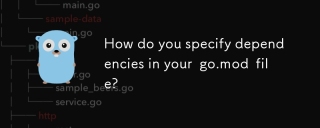
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
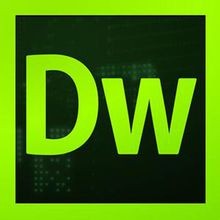
Dreamweaver CS6
Visual web development tools
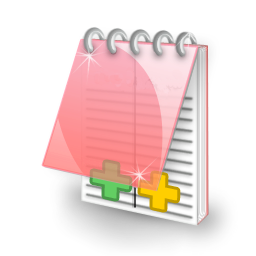
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
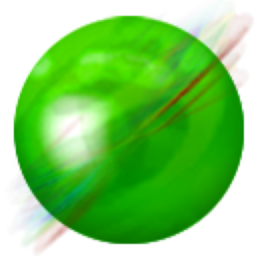
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
