


Infinity Loop Slider Concepts
This article discusses the best practices, such as readability, code reusability, and good coding practices, for building an Infinity-Image-Loop-Slider for a website using JavaScript/jQuery. The focus is on how to arrange the pictures to create the illusion of an unending loop slider.
Implementing an Infinity Loop Slider
One straightforward method for creating an infinite image slider is as follows: Assume you have "n" images to slide in a loop, with the first image following the nth image and vice-versa. Create a clone of the first and last images and do the following:
- Append the clone of the last image before the first image.
- Append the clone of the first image after the last image.
Regardless of the number of images, you only need to insert two cloned items at most.
Assuming all images are 100px wide and displayed within a container with overflow: hidden, display: inline-block, and white-space: nowrap, the container holding the images can be easily aligned in a row.
For n = 4, the DOM structure would appear as follows:
offset(px) 0 100 200 300 400 500 images | 4c | 1 | 2 | 3 | 4 | 1c /* ^^ ^^ [ Clone of the last image ] [ Clone of the 1st image ] */
Initially, the container is positioned with left: -100px, allowing the first image to be displayed. To switch between images, apply a JavaScript animation to the CSS property you originally selected.
- When the slider is on the 4th image, moving to image 1c involves animating from image 4 to 1c. Once the animation is complete, instantly reposition the slider wrapper at the actual offset of the 1st image (e.g., set left: -100px to the container).
- Similarly, when the slider is positioned on the 1st element, performing the previous animation from image 1 to 4c and moving the container to the 4th image offset (left: -400px to the container) is sufficient to display the previous image.
Orchestrating the Slide Loop
The accompanying fiddle demonstrates this effect. Below is the basic JavaScript/jQuery code used:
$(function() { var gallery = $('#gallery ul'), items = gallery.find('li'), len = items.length, current = 1, /* the item we're currently looking */ first = items.filter(':first'), last = items.filter(':last'), triggers = $('button'); /* 1. Cloning first and last item */ first.before(last.clone(true)); last.after(first.clone(true)); /* 2. Set button handlers */ triggers.on('click', function() { var cycle, delta; if (gallery.is(':not(:animated)')) { cycle = false; delta = (this.id === "prev")? -1 : 1; /* in the example buttons have id "prev" or "next" */ gallery.animate({ left: "+=" + (-100 * delta) }, function() { current += delta; /** * we're cycling the slider when the the value of "current" * variable (after increment/decrement) is 0 or when it exceeds * the initial gallery length */ cycle = (current === 0 || current > len); if (cycle) { /* we switched from image 1 to 4-cloned or from image 4 to 1-cloned */ current = (current === 0)? len : 1; gallery.css({left: -100 * current }); } }); } }); });
Conclusion
This solution is relatively straightforward and efficient, requiring only two additional DOM insertion operations and simple loop management logic compared to a non-looping slider.
While alternative approaches may exist, this method provides a practical and effective solution for creating an infinity loop slider.
The above is the detailed content of How can I create an infinite image loop slider using JavaScript/jQuery?. For more information, please follow other related articles on the PHP Chinese website!
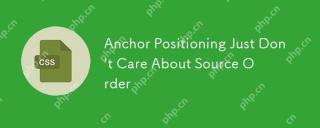
The fact that anchor positioning eschews HTML source order is so CSS-y because it's another separation of concerns between content and presentation.
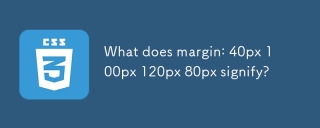
Article discusses CSS margin property, specifically "margin: 40px 100px 120px 80px", its application, and effects on webpage layout.
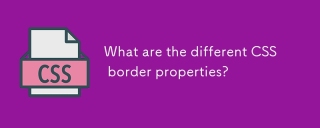
The article discusses CSS border properties, focusing on customization, best practices, and responsiveness. Main argument: border-radius is most effective for responsive designs.
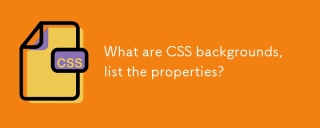
The article discusses CSS background properties, their uses in enhancing website design, and common mistakes to avoid. Key focus is on responsive design using background-size.
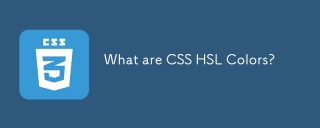
Article discusses CSS HSL colors, their use in web design, and advantages over RGB. Main focus is on enhancing design and accessibility through intuitive color manipulation.

The article discusses the use of comments in CSS, detailing single-line and multi-line comment syntaxes. It argues that comments enhance code readability, maintainability, and collaboration, but may impact website performance if not managed properly.
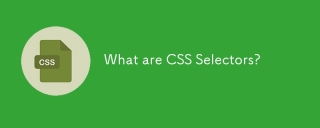
The article discusses CSS Selectors, their types, and usage for styling HTML elements. It compares ID and class selectors and addresses performance issues with complex selectors.
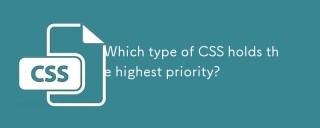
The article discusses CSS priority, focusing on inline styles having the highest specificity. It explains specificity levels, overriding methods, and debugging tools for managing CSS conflicts.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
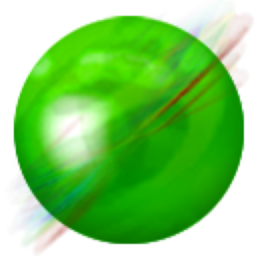
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
