


Java Runtime Error: ClassNotFoundException: com.mysql.jdbc.Driver
Problem Description:
A Java application using JDBC to connect to a MySQL database encounters a "ClassNotFoundException" when attempting to instantiate the MySQL JDBC driver.
Possible Causes:
- Missing JDBC Driver Library: The com.mysql.jdbc.Driver class is missing from the application's runtime classpath.
Solution:
-
Add JDBC Driver to Classpath: Add the MySQL JDBC driver JAR file (e.g., mysql-connector-java-5.1.25-bin.jar) to the application's classpath. This can be done when running the application using the "-cp" parameter:
- Windows: java -cp .;mysql-connector-java-5.1.25-bin.jar ClientBase
- Linux/Mac: java -cp .:mysql-connector-java-5.1.25-bin.jar ClientBase
Example Code:
<code class="java">// import packages import java.sql.*; // create class ClientBase public class ClientBase { // JDBC driver name and database URL static final String JDBC_DRIVER = "com.mysql.jdbc.Driver"; static final String DB_URL = "jdbc:mysql://localhost/CLIENTBASE"; // Database credentials static final String USER = "root"; static final String PASS = ""; // Begin method main public static void main(String[] args) { Connection conn = null; Statement stmt = null; try { // Register JDBC driver Class.forName(JDBC_DRIVER); // Add this line if the driver is not found // Open connection System.out.println("Connecting to database..."); conn = DriverManager.getConnection(DB_URL, USER, PASS); // Execute a query System.out.println("Creating statement..."); stmt = conn.createStatement(); String sql; sql = "SELECT id, name, address, address 2, city, phone, state, zip, fax FROM CLIENTBASE"; ResultSet rs = stmt.executeQuery(sql); // Extract data from result set while (rs.next()) { // Retrieve by column name int id = rs.getInt("id"); String name = rs.getString("name"); String address = rs.getString("address"); String address2 = rs.getString("address2"); String city = rs.getString("city"); String phone = rs.getString("phone"); String state = rs.getString("state"); String zip = rs.getString("zip"); String fax = rs.getString("fax"); // Display values System.out.print("ID: " + id); System.out.print(" Name: " + name); System.out.println("Address:" + address); System.out.println(address2); System.out.print("City:" + city); System.out.print(" State: " + state); System.out.println(" Zip: " + zip); System.out.print("Phone: " + phone); System.out.println(" Fax: " + fax); } // end while // clean up rs.close(); stmt.close(); conn.close(); } catch (SQLException se) { // Handle errors for JDBC se.printStackTrace(); } catch (Exception e) { // Handle errors for Class.forName e.printStackTrace(); } finally { // finally block used to close resources try { if (stmt != null) stmt.close(); } catch (SQLException se) { se.printStackTrace(); } // end finally } // end try System.out.println("Goodbye."); } // End method main } // end class ClientBase</code>
The above is the detailed content of Why am I getting a \'ClassNotFoundException: com.mysql.jdbc.Driver\' error when connecting to my MySQL database using JDBC?. For more information, please follow other related articles on the PHP Chinese website!
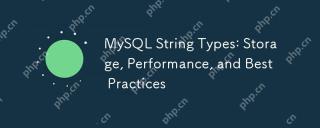
MySQLstringtypesimpactstorageandperformanceasfollows:1)CHARisfixed-length,alwaysusingthesamestoragespace,whichcanbefasterbutlessspace-efficient.2)VARCHARisvariable-length,morespace-efficientbutpotentiallyslower.3)TEXTisforlargetext,storedoutsiderows,
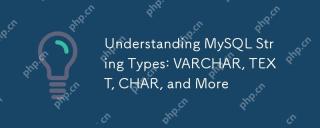
MySQLstringtypesincludeVARCHAR,TEXT,CHAR,ENUM,andSET.1)VARCHARisversatileforvariable-lengthstringsuptoaspecifiedlimit.2)TEXTisidealforlargetextstoragewithoutadefinedlength.3)CHARisfixed-length,suitableforconsistentdatalikecodes.4)ENUMenforcesdatainte
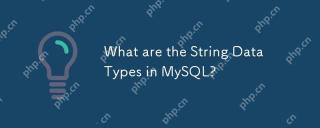
MySQLoffersvariousstringdatatypes:1)CHARforfixed-lengthstrings,2)VARCHARforvariable-lengthtext,3)BINARYandVARBINARYforbinarydata,4)BLOBandTEXTforlargedata,and5)ENUMandSETforcontrolledinput.Eachtypehasspecificusesandperformancecharacteristics,sochoose
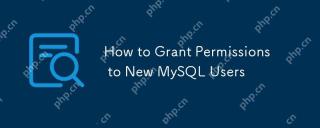
TograntpermissionstonewMySQLusers,followthesesteps:1)AccessMySQLasauserwithsufficientprivileges,2)CreateanewuserwiththeCREATEUSERcommand,3)UsetheGRANTcommandtospecifypermissionslikeSELECT,INSERT,UPDATE,orALLPRIVILEGESonspecificdatabasesortables,and4)
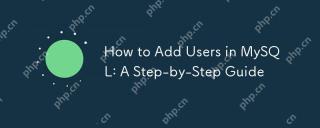
ToaddusersinMySQLeffectivelyandsecurely,followthesesteps:1)UsetheCREATEUSERstatementtoaddanewuser,specifyingthehostandastrongpassword.2)GrantnecessaryprivilegesusingtheGRANTstatement,adheringtotheprincipleofleastprivilege.3)Implementsecuritymeasuresl
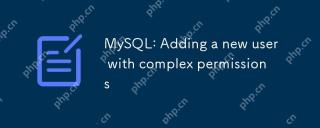
ToaddanewuserwithcomplexpermissionsinMySQL,followthesesteps:1)CreatetheuserwithCREATEUSER'newuser'@'localhost'IDENTIFIEDBY'password';.2)Grantreadaccesstoalltablesin'mydatabase'withGRANTSELECTONmydatabase.TO'newuser'@'localhost';.3)Grantwriteaccessto'
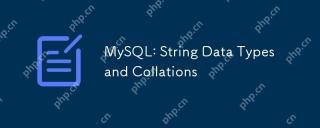
The string data types in MySQL include CHAR, VARCHAR, BINARY, VARBINARY, BLOB, and TEXT. The collations determine the comparison and sorting of strings. 1.CHAR is suitable for fixed-length strings, VARCHAR is suitable for variable-length strings. 2.BINARY and VARBINARY are used for binary data, and BLOB and TEXT are used for large object data. 3. Sorting rules such as utf8mb4_unicode_ci ignores upper and lower case and is suitable for user names; utf8mb4_bin is case sensitive and is suitable for fields that require precise comparison.
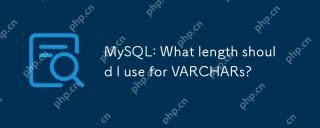
The best MySQLVARCHAR column length selection should be based on data analysis, consider future growth, evaluate performance impacts, and character set requirements. 1) Analyze the data to determine typical lengths; 2) Reserve future expansion space; 3) Pay attention to the impact of large lengths on performance; 4) Consider the impact of character sets on storage. Through these steps, the efficiency and scalability of the database can be optimized.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
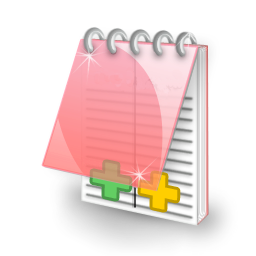
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
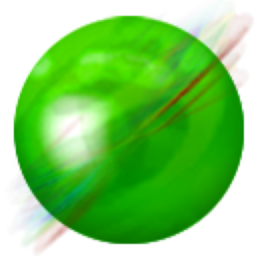
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
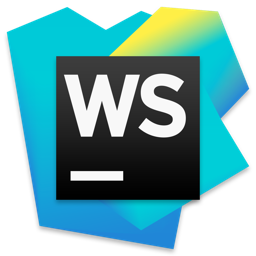
WebStorm Mac version
Useful JavaScript development tools
