How to Dynamically Modify Route Handlers in Go's HTTP Mux
Managing routes and their associated handlers is a crucial aspect of web development in Go. The standard library's http.Mux and third-party options like gorilla/mux provide versatile routing functionality. However, there may be instances where you need to modify or replace route handlers dynamically without restarting the running application.
Approach 1: Custom Handler Wrapper
One approach is to create a custom handler wrapper that implements the http.Handler interface. This handler wrapper can encapsulate the original handler function and provide additional logic to control whether or not the handler should be executed. For example:
<code class="go">type HandlerWrapper struct { http.HandlerFunc Enabled bool } func (hw *HandlerWrapper) ServeHTTP(w http.ResponseWriter, r *http.Request) { if hw.Enabled { hw.HandlerFunc.ServeHTTP(w, r) } else { http.Error(w, "Not Found", http.StatusNotFound) } }</code>
With this wrapper, you can register the original handler function with the HTTP mux using the handler wrapper instead:
<code class="go">mux.HandleFunc("/route", &HandlerWrapper{HandlerFunc: myHandler, Enabled: true})</code>
Approach 2: Using Middleware
Alternatively, you can create a middleware function that intercepts requests and checks if the route corresponding to the request is enabled. If the route is disabled, the middleware can return an error response or skip further processing.
<code class="go">func RouteMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // Check if the route is enabled using logic // ... // If enabled, continue to the next handler next.ServeHTTP(w, r) }) }</code>
<code class="go">mux.Use(RouteMiddleware) mux.HandleFunc("/route", myHandler)</code>
Conclusion
By implementing one of these approaches, you can dynamically control the availability of routes in your Go application without the need for restarts. This flexibility is especially useful for applications where the ability to enable or disable certain features or sections of the API is required.
The above is the detailed content of How to Dynamically Modify Route Handlers in Go\'s HTTP Mux?. For more information, please follow other related articles on the PHP Chinese website!
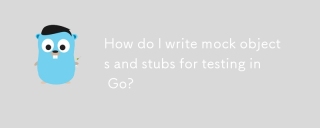
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
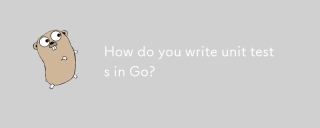
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
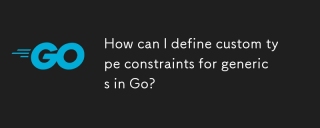
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
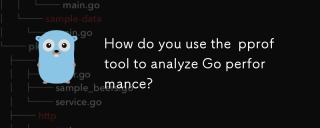
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
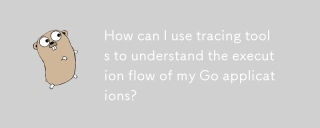
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
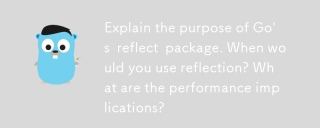
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
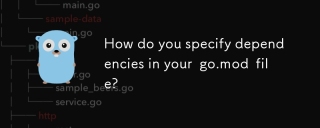
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
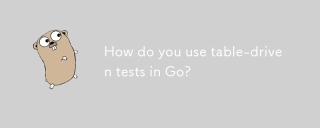
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
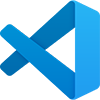
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
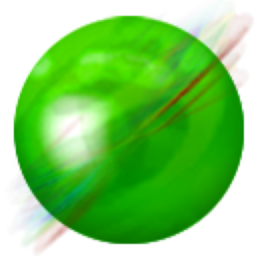
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
