"tail -f"-like Generator in Go
Problem
Tailing a file in Go requires a loop with a sleep on EOF, which can be error-prone and inefficient. Is there a cleaner way to do this?
Solution
Instead of using a goroutine, create a wrapper around a reader that sleeps on EOF:
<code class="go">type tailReader struct { io.ReadCloser } func (t tailReader) Read(b []byte) (int, error) { for { n, err := t.ReadCloser.Read(b) if n > 0 { return n, nil } else if err != io.EOF { return n, err } time.Sleep(10 * time.Millisecond) } } func newTailReader(fileName string) (tailReader, error) { f, err := os.Open(fileName) if err != nil { return tailReader{}, err } if _, err := f.Seek(0, 2); err != nil { return tailReader{}, err } return tailReader{f}, nil }</code>
Usage
This reader can be used with any io.Reader, including bufio.Scanner and json.Decoder. For example:
<code class="go">t, err := newTailReader("somefile") if err != nil { log.Fatal(err) } defer t.Close() // Use with bufio.Scanner scanner := bufio.NewScanner(t) for scanner.Scan() { fmt.Println(scanner.Text()) } // Use with json.Decoder dec := json.NewDecoder(t) for { var v SomeType if err := dec.Decode(&v); err != nil { log.Fatal(err) } fmt.Println("the value is ", v) }</code>
Advantages
- Easy Shutdown: Just close the file.
- Works with Many Packages: Many packages (like bufio.Scanner and json.Decoder) work with io.Reader.
- Configurable Sleep: Adjust the sleep time to optimize latency or CPU usage.
The above is the detailed content of How Can I Create a \'tail -f\' Like Generator in Go?. For more information, please follow other related articles on the PHP Chinese website!
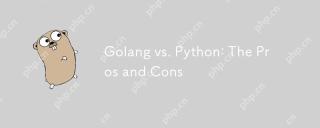
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
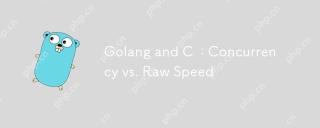
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
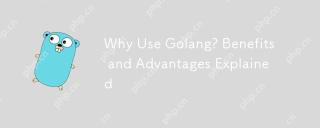
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
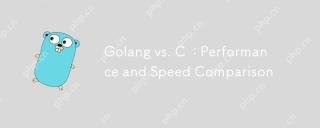
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
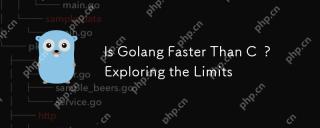
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
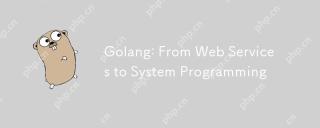
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
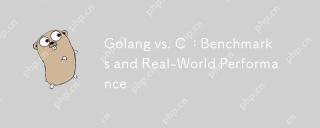
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
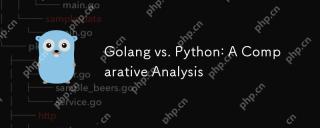
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
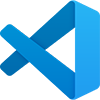
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software