Testing Coverage for Code Separated from Tests
Despite the advantage of organizing test files separately for a cleaner codebase and limiting tests to public API interactions, it poses a challenge in obtaining coverage for the target package under test (in this case, api_client).
To address this issue, we can leverage the -coverpkg flag along with the package name when running tests:
go test -cover -coverpkg "api_client" "api_client_tests"
This command will run the tests with coverage enabled for the api_client package.
While separating test files from code files is permissible, it's important to note that it deviates from Go's standard approach. This means that package-private variables or functions will not be accessible by tests outside of the package, even if they are in separate directories.
To enforce black-box testing, where tests should only access public API methods, you can still keep tests in separate packages without physically moving the files. For example:
<code class="go">// api_client.go package api_client // Private variable not accessible outside package var privateVar = 10 func PublicMethod() {}</code>
<code class="go">// api_client_test.go package api_client_tests import ( "testing" "api_client" // Import api_client package ) func TestPublicMethod(t *testing.T) { api_client.PublicMethod() }</code>
In this example, the privateVar and Method function from api_client.go are inaccessible to the test file, ensuring that tests interact only via the public API.
The above is the detailed content of How to Achieve Test Coverage for Code Separated from Tests in Go?. For more information, please follow other related articles on the PHP Chinese website!
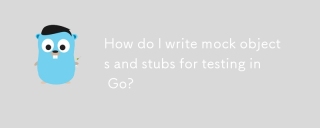
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
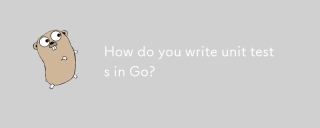
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
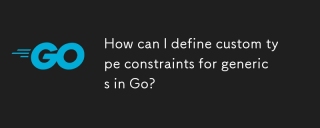
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
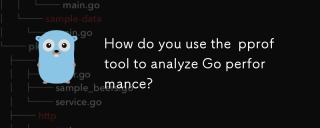
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
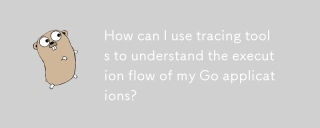
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
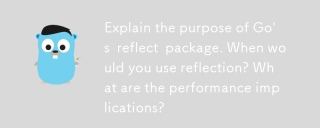
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
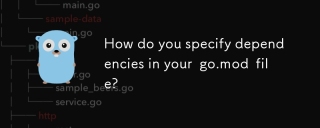
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
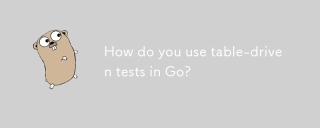
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
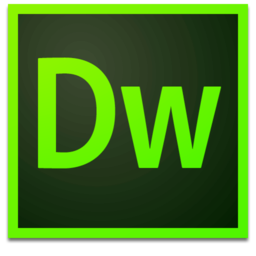
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
