


Organizing JavaScript Objects by Property Names
Sorting JavaScript objects alphabetically by property names is a common requirement, but it poses a challenge as the object keys order is not guaranteed. This article addresses this issue and provides a solution for organizing objects in a specific order.
Outdated ES5 Solution:
As the ES5 specification does not define an order for object keys, the following method can be used, but is not considered future-proof:
<code class="javascript">function sortObject(o) { var sorted = {}, key, a = []; for (key in o) { if (o.hasOwnProperty(key)) { a.push(key); } } a.sort(); for (key = 0; key <p>This solution iterates over the object's keys, sorts them in alphabetical order, and then creates a new object with the sorted keys and their corresponding values.</p> <p><strong>ES6 Compliant Solution:</strong></p> <p>With the introduction of ES6, object keys are now guaranteed to be in the order they were defined. Therefore, sorting can be achieved more concisely:</p> <pre class="brush:php;toolbar:false"><code class="javascript">const sortedObject = Object.fromEntries( Object.entries(originalObject).sort((a, b) => a[0].localeCompare(b[0])) );</code>
This method converts the object to an array of key-value pairs, sorts the array based on the keys, and then converts the sorted array back into an object using Object.fromEntries().
Conclusion:
While the order of object keys in ES5 is undefined, the provided solutions can be used to sort objects by property names. In ES6 and later, the object keys order is guaranteed, making the sorting process more straightforward.
The above is the detailed content of How to Sort JavaScript Objects by Property Names: ES5 vs. ES6 Approaches. For more information, please follow other related articles on the PHP Chinese website!
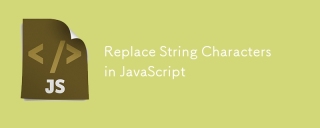
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
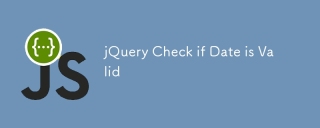
Simple JavaScript functions are used to check if a date is valid. function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] '/' bits[1] '/' bits[0]); return !!(d && (d.getMonth() 1) == bits[1] && d.getDate() == Number(bits[0])); } //test var
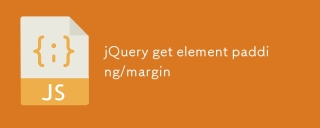
This article discusses how to use jQuery to obtain and set the inner margin and margin values of DOM elements, especially the specific locations of the outer margin and inner margins of the element. While it is possible to set the inner and outer margins of an element using CSS, getting accurate values can be tricky. // set up $("div.header").css("margin","10px"); $("div.header").css("padding","10px"); You might think this code is
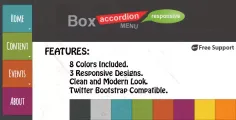
This article explores ten exceptional jQuery tabs and accordions. The key difference between tabs and accordions lies in how their content panels are displayed and hidden. Let's delve into these ten examples. Related articles: 10 jQuery Tab Plugins
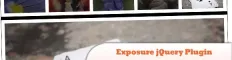
Discover ten exceptional jQuery plugins to elevate your website's dynamism and visual appeal! This curated collection offers diverse functionalities, from image animation to interactive galleries. Let's explore these powerful tools: Related Posts: 1
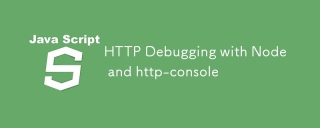
http-console is a Node module that gives you a command-line interface for executing HTTP commands. It’s great for debugging and seeing exactly what is going on with your HTTP requests, regardless of whether they’re made against a web server, web serv
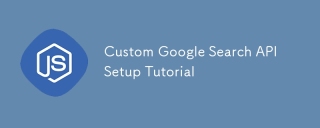
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
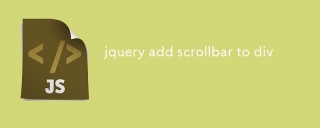
The following jQuery code snippet can be used to add scrollbars when the div content exceeds the container element area. (No demonstration, please copy it directly to Firebug) //D = document //W = window //$ = jQuery var contentArea = $(this), wintop = contentArea.scrollTop(), docheight = $(D).height(), winheight = $(W).height(), divheight = $('#c


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
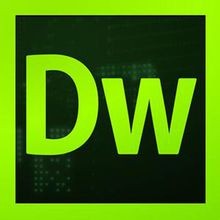
Dreamweaver CS6
Visual web development tools
