


Why am I getting a \'Using the variable on range scope x in function literal\' error in Go?
Using Variables on Range Scope x in Function Literals
Problem:
In the following code snippet, the go vet tool is reporting an error: "Using the variable on range scope x in function literal (scopelint)".
<code class="go">func TestGetUID(t *testing.T) { for _, x := range tests { t.Run(x.description, func(t *testing.T) { client := fake.NewSimpleClientset(x.objs...) actual := getUID(client, x.namespace) assert.Equal(t, x.expected, actual) }) } }</code>
Explanation:
The error message indicates that x, which is a loop variable, is being used in a function literal that is passed to t.Run(). The compiler cannot guarantee that the function literal will not be called after t.Run() returns, which could lead to a data race or other unexpected behavior.
Solution:
To resolve this issue, you can make a copy of x and use that copy in the function literal:
<code class="go">func TestGetUID(t *testing.T) { for _, x := range tests { x2 := x // Copy the value of x t.Run(x2.description, func(t *testing.T) { client := fake.NewSimpleClientset(x2.objs...) actual := getUID(client, x2.namespace) assert.Equal(t, x2.expected, actual) }) } }</code>
Alternatively, you can shadow the loop variable x by assigning it to a new variable of the same name within the function literal:
<code class="go">func TestGetUID(t *testing.T) { for _, x := range tests { t.Run(x.description, func(t *testing.T) { x := x // Shadow the loop variable client := fake.NewSimpleClientset(x.objs...) actual := getUID(client, x.namespace) assert.Equal(t, x.expected, actual) }) } }</code>
The above is the detailed content of Why am I getting a \'Using the variable on range scope x in function literal\' error in Go?. For more information, please follow other related articles on the PHP Chinese website!
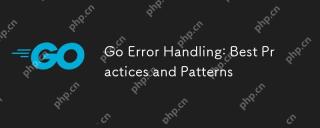
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
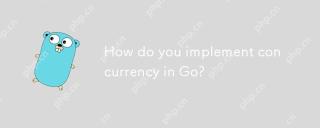
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
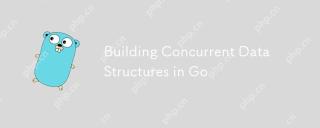
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
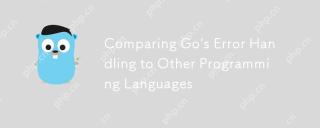
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand
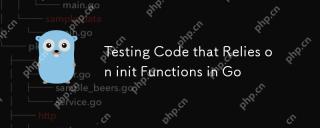
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
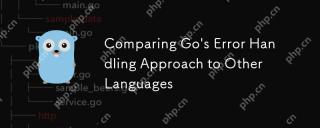
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
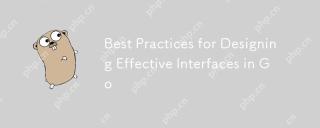
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
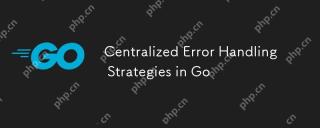
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
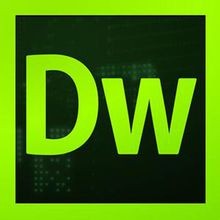
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
