


How to Effectively Test GraphQL Queries and Mutations with Testify and GQLgen in Golang?
Unit Testing GraphQL in Golang
When testing GraphQL queries and mutations in a Golang application, it's crucial to have a robust testing strategy to ensure the functionality and reliability of your API endpoints.
Nestled within the labyrinth of Golang testing frameworks, testify reigns supreme as a preferred choice for its simplicity and comprehensiveness. Combined with the gqlgen/client package, which provides invaluable assistance in testing GraphQL, you can delve into the rewarding realm of effective unit testing.
Let's embark on a practical example to illuminate the process of testing GraphQL queries and mutations:
<code class="go">// graph/resolver/root.resolver_test.go import ( "context" "testing" "github.com/99designs/gqlgen/client" "github.com/99designs/gqlgen/graphql/handler" "github.com/mrdulin/gqlgen-cnode/graph/generated" "github.com/mrdulin/gqlgen-cnode/graph/model" "github.com/mrdulin/gqlgen-cnode/mocks" "github.com/stretchr/testify/mock" "github.com/stretchr/testify/require" ) ... type MockedUserService struct { mock.Mock } func (s *MockedUserService) GetUserByLoginname(loginname string) *model.UserDetail { args := s.Called(loginname) return args.Get(0).(*model.UserDetail) } func (s *MockedUserService) ValidateAccessToken(accesstoken string) *model.UserEntity { args := s.Called(accesstoken) return args.Get(0).(*model.UserEntity) } ...</code>
Utilizing these mock objects, we can proceed to craft comprehensive unit tests that validate the functionality of our GraphQL resolvers:
<code class="go">// graph/resolver/root.resolver_test.go ... // TestMutationResolver_ValidateAccessToken is a test example for the ValidateAccessToken mutation. func TestMutationResolver_ValidateAccessToken(t *testing.T) { t.Run("should validate accesstoken correctly", func(t *testing.T) { // Create a mocked user service mockedUserService := new(mocks.MockedUserService) // Inject the mocked service into our resolver resolvers := resolver.Resolver{UserService: mockedUserService} // Create a GraphQL client c := client.New(handler.NewDefaultServer(generated.NewExecutableSchema(generated.Config{Resolvers: &resolvers}))) // Set up expected return values from the mock service ue := model.UserEntity{ID: "123", User: model.User{Loginname: &loginname, AvatarURL: &avatarURL}} mockedUserService.On("ValidateAccessToken", mock.AnythingOfType("string")).Return(&ue) // Run the GraphQL mutation query var resp struct { ValidateAccessToken struct{ ID, Loginname, AvatarUrl string } } q := ` mutation { validateAccessToken(accesstoken: "abc") { id, loginname, avatarUrl } } ` c.MustPost(q, &resp) // Assert that the mock service was called as expected mockedUserService.AssertExpectations(t) // Check the response from the GraphQL mutation require.Equal(t, "123", resp.ValidateAccessToken.ID) require.Equal(t, "mrdulin", resp.ValidateAccessToken.Loginname) require.Equal(t, "avatar.jpg", resp.ValidateAccessToken.AvatarUrl) }) } ...</code>
By implementing this testing approach, you can effectively scrutinize your GraphQL resolvers and equip your application with a solid foundation of quality and reliability.
The above is the detailed content of How to Effectively Test GraphQL Queries and Mutations with Testify and GQLgen in Golang?. For more information, please follow other related articles on the PHP Chinese website!
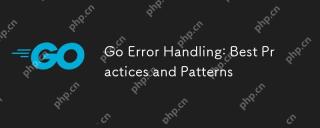
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
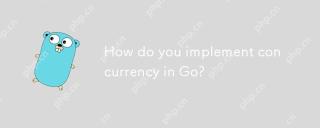
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
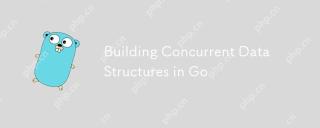
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
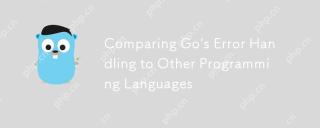
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand
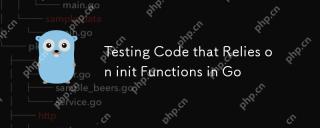
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
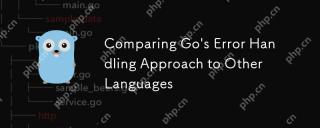
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
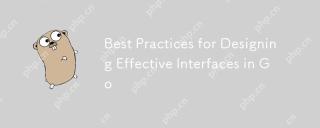
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
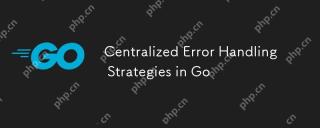
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
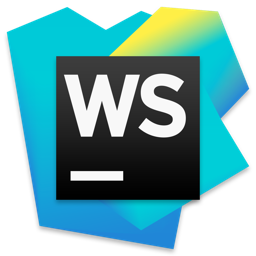
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Chinese version
Chinese version, very easy to use
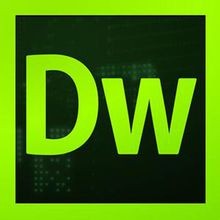
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
