


How to Convert Complex MySQL Queries with Multiple Statements to Laravel Eloquent?
Converting Complex MySQL Queries with Multiple Statements to Laravel Eloquent
In the world of database querying, the ability to convert intricate SQL queries to their Laravel Eloquent counterparts is crucial. One such challenge arises when dealing with multi-statement MySQL queries, which involve a series of statements such as PREPARE, EXECUTE, and DEALLOCATE.
Problem Statement
You have a MySQL query with multiple statements:
SELECT GROUP_CONCAT(DISTINCT CONCAT( 'ifnull(SUM(case when location_code = ''', location_code , ''' then quantity end),0) AS `', location_code , '`' ) ) INTO @sql FROM item_details; SET @sql = CONCAT('SELECT item_number,SUM(quantity) as "total_quantity", ', @sql, ' FROM item_details GROUP BY item_number'); PREPARE stmt FROM @sql; EXECUTE stmt; DEALLOCATE PREPARE stmt;
You're looking to convert this complex query to Laravel Eloquent, but you're unsure how to handle the multiple statements involved.
Solution
While some aspects of the MySQL query, such as the preparation and execution of the statement, are not directly translatable to Eloquent, the core functionality can be achieved. Here's a solution:
<code class="php">DB::table('item_details') ->selectRaw('GROUP_CONCAT(...) INTO @sql') ->get(); $sql = DB::selectOne('select @sql') ->{'@sql'}; ItemDetails::select('item_number', DB::raw('SUM(quantity) as total_quantity')) ->selectRaw($sql) ->groupBy('item_number') ->get();</code>
Explanation
This solution is a blend of Eloquent and raw SQL queries.
- DB::table('item_details')->selectRaw('GROUP_CONCAT(...) INTO @sql')->get(); executes the first statement, which creates a temporary SQL variable named @sql.
- $sql = DB::selectOne('select @sql')->{'@sql'}; retrieves the value of the @sql variable and stores it in the $sql variable.
- ItemDetails::select('item_number', DB::raw('SUM(quantity) as total_quantity'))->selectRaw($sql)->groupBy('item_number')->get(); constructs the main Eloquent query. It uses selectRaw($sql) to insert the dynamic SQL string obtained from $sql.
This approach allows you to perform the complex MySQL query within the context of Laravel Eloquent, simplifying your code and maintaining maintainability.
The above is the detailed content of How to Convert Complex MySQL Queries with Multiple Statements to Laravel Eloquent?. For more information, please follow other related articles on the PHP Chinese website!
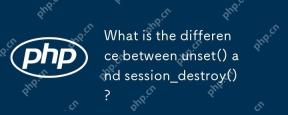
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
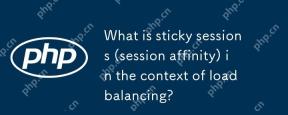
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
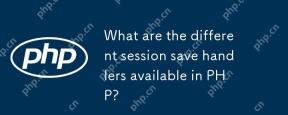
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
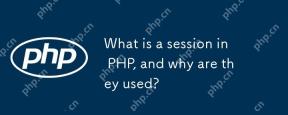
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
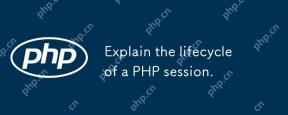
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
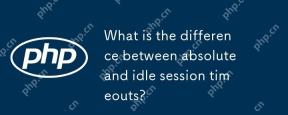
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
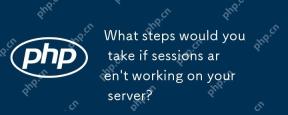
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
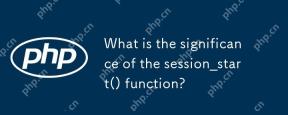
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
