


Overriding Embedded Struct Methods in Go
In Go, embedded structs inherit the fields and methods of their base structs. However, a conflict can arise when both the base and embedded structs implement a method with the same name. This article explores a solution to override the embedded struct's method without affecting the base struct.
Consider the following code:
<code class="go">package main import "fmt" type Base struct { val int } func (b *Base) Set(i int) { b.val = i } type Sub struct { Base changed bool } func (b *Sub) Set(i int) { b.val = i b.changed = true } func main() { s := &Sub{} s.Base.Set(1) var b *Base = &s.Base // Both print the same value fmt.Printf("%+v\n", b) fmt.Printf("%+v\n", s) }</code>
Here, the Sub type embeds the Base type. Both Sub and Base have a method named Set, and when you call s.Base.Set(), you are bypassing the Sub.Set() method and directly calling the Base.Set() method.
To override the embedded struct's method, you can call the Sub.Set() method instead. In Go, when a type implements a method with the same name as its embedded type, the embedded method is hidden.
Here's an updated version of the code:
<code class="go">func (b *Sub) Set(i int) { b.Base.Set(i) // Call the Base.Set() method b.changed = true } func main() { s := &Sub{} s.Set(1) var b *Base = &s.Base // Note: b.val is now 1 // s.changed is now true fmt.Printf("%+v\n", b) fmt.Printf("%+v\n", s) }</code>
In this example, when you call s.Set(1), the Sub.Set() method will be invoked, which in turn calls the Base.Set() method. This updates the val field of the Base struct embedded in Sub. The changed field will also be set to true to indicate that the value has been modified.
This solution allows you to override the embedded struct's method without affecting the base struct. It is a common technique used in Go to achieve code reusability and flexibility.
The above is the detailed content of How to Override Embedded Struct Methods in Go Without Affecting the Base Struct?. For more information, please follow other related articles on the PHP Chinese website!
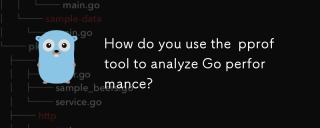
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
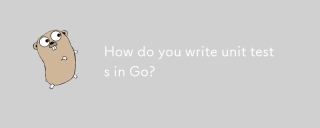
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
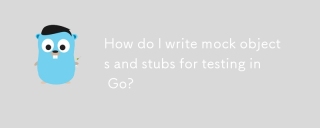
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
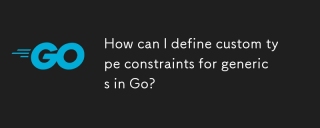
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
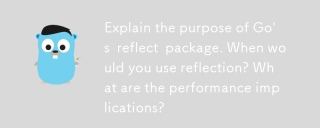
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
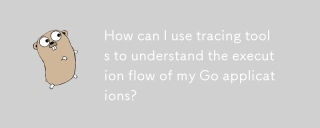
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
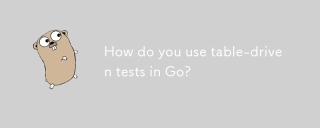
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
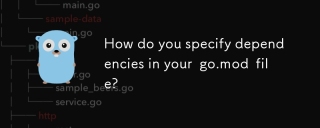
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
