PHP is commonly paired with MySQL, one of the most widely used open-source relational database management systems, to handle data with speed and efficiency in both small and large-scale projects.
Whether you're creating a simple website or an advanced web application, you need to know how to connect PHP to a MySQL database.
Overview of MySQL and PHP Integration
MySQL, known for being an open-source relational database management system or RDBMS, is widely appreciated for its performance, dependability, and ease of use.
It’s highly adaptable for applications of all kinds, ranging from small-scale websites to large, intricate enterprise systems. Its flexibility and efficiency make MySQL a preferred choice for developers seeking a solid database foundation.
PHP, a server-side scripting language, works seamlessly with MySQL to build interactive, data-driven web pages. Together, they provide a powerful backend solution that makes them the perfect option for websites and web applications.
Setting Up the MySQL Database
To set up your MySQL database, start by creating it using either the MySQL command-line interface (CLI) or a web tool such as phpMyAdmin. Using the CLI, leverage the CREATE DATABASE command to initiate a new database.
Using this straightforward command will create an empty database ready for your application's data. If you prefer a graphical interface, phpMyAdmin offers an easy way to accomplish the same task through a few clicks.
After creating the database, you need to set up users with the appropriate permissions for security purposes. You can use the CREATE USER command to add a new user and the GRANT command to assign specific permissions to them.
Choosing a PHP MySQL Extension: MySQLi vs. PDO
When choosing a PHP extension for MySQL, you have two primary options: MySQLi and PDO. Each offers distinct features and benefits, so the decision will depend on your specific needs and potential future requirements.
MySQLi Extension
MySQLi, which stands for MySQL Improved, provides an upgraded way to work with MySQL databases, offering better features compared to older methods.
You can choose between object-oriented and procedural APIs with MySQLi, which makes it quite flexible depending on your coding style. However, MySQLi only works with MySQL databases, limiting its use if you plan to expand beyond that.
PDO (PHP Data Objects)
PDO is a more versatile option, as it supports 12 different database systems, not just MySQL. This makes it a more valuable choice if you anticipate switching databases in the future.
PDO uses an entirely object-oriented approach and offers advanced features like prepared statements, enhancing security and portability across different database environments.
Establishing a Connection
Establishing a reliable connection to your MySQL database is the first step in building dynamic PHP applications.
Connecting to MySQL with MySQLi
To connect to a MySQL database using MySQLi, you can opt for either an object-oriented or procedural approach. In the object-oriented method, simply create a new instance of the mysqli class using your server name, username, and password.
With the procedural approach, call the ‘mysqli_connect()’ function to establish the connection. Once connected, it’s important to check the connection status. You can do this with ‘$conn->connect_error’ in the object-oriented version or ‘mysqli_connect_error()’ procedurally.
Connecting to MySQL with PDO
When connecting with PDO, create a new instance of the PDO class, specifying the server and database details directly in the connection string. To handle exceptions effectively, use the ‘PDO::ATTR_ERRMODE’ attribute to set the error mode to ‘PDO::ERRMODE_EXCEPTION’.
Now, an exception will be thrown if an error ensues, allowing you to manage it much more precisely. Also, keep in mind that PDO requires you to specify a valid database during the connection setup; otherwise, an exception will be thrown, and the connection will fail.
Performing Basic Database Operations
To effectively manage data in your MySQL database, you need to understand how to execute basic SQL operations and how the operations function.
Executing Queries
To interact with your MySQL database, you need to execute basic SQL queries such as SELECT, INSERT, UPDATE, and DELETE.
Using MySQLi, you can run these queries with ‘$conn->query($sql)’ for the object-oriented approach or ‘mysqli_query($conn, $sql)’ for the procedural one.
For PDO, use ‘$conn->exec($sql)’ for queries that modify the database or ‘$conn->query($sql)’ for retrieval. Each method provides a simple way to execute commands, helping you manage your database efficiently.
Prepared Statements
Prepared statements help keep your application safe from SQL injection. With MySQLi, you can prepare a statement using ‘$conn->prepare("INSERT INTO users (name, email) VALUES (?, ?)")’ and then bind parameters using ‘$stmt->bind_param("ss", $name, $email)’.
For PDO, use ‘$conn->prepare("INSERT INTO users (name, email) VALUES (:name, :email)")’ and execute with ‘$stmt->execute(['name' => $name, 'email' => $email])’.
Error Handling and Debugging
Effectively handling and debugging errors is essential for maintaining a stable connection to your MySQL database and identifying issues with SQL queries, whether you are using MySQLi or PDO.
- MySQLi: To handle errors when using MySQLi, you can use ‘$conn->error’ for the object-oriented approach or ‘mysqli_error($conn)’ for the procedural method. These functions help you capture and display database errors, making it easier to debug issues related to connections or SQL queries so that you can identify and address problems effectively.
- PDO: PDO uses its own exception class, PDOException, to manage errors efficiently. You could utilize a ‘try...catch’ block so you can detect exceptions and respond accordingly. When an error occurs, ‘catch(PDOException $e)’ allows you to output a specific error message, providing more refined control over how errors are handled, which makes debugging and maintaining your code much easier.
Closing the Database Connection
You can explicitly close the database connection using ‘$conn->close()’ for MySQLi in an object-oriented style, ‘mysqli_close($conn)’ for the procedural approach, or setting ‘$conn = null’ for PDO. Although PHP will automatically close the connection at the end of the script, closing it manually is a good practice to free up server resources and enhance performance.
Always close your database connection when it’s no longer needed. Utilize prepared statements to defend against SQL injection and strengthen your application’s security.
The above is the detailed content of Connecting to a MySQL Database in PHP. For more information, please follow other related articles on the PHP Chinese website!
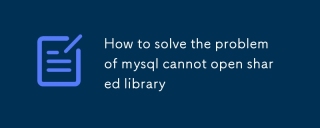
This article addresses MySQL's "unable to open shared library" error. The issue stems from MySQL's inability to locate necessary shared libraries (.so/.dll files). Solutions involve verifying library installation via the system's package m
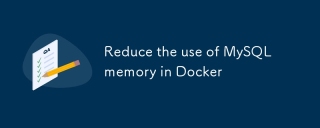
This article explores optimizing MySQL memory usage in Docker. It discusses monitoring techniques (Docker stats, Performance Schema, external tools) and configuration strategies. These include Docker memory limits, swapping, and cgroups, alongside
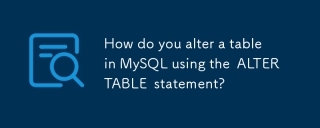
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
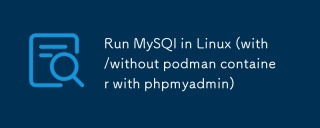
This article compares installing MySQL on Linux directly versus using Podman containers, with/without phpMyAdmin. It details installation steps for each method, emphasizing Podman's advantages in isolation, portability, and reproducibility, but also
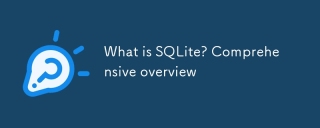
This article provides a comprehensive overview of SQLite, a self-contained, serverless relational database. It details SQLite's advantages (simplicity, portability, ease of use) and disadvantages (concurrency limitations, scalability challenges). C
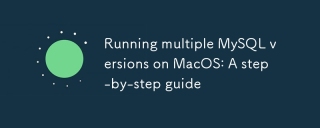
This guide demonstrates installing and managing multiple MySQL versions on macOS using Homebrew. It emphasizes using Homebrew to isolate installations, preventing conflicts. The article details installation, starting/stopping services, and best pra
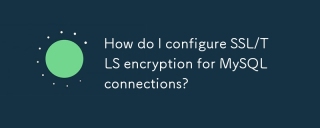
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
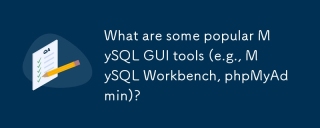
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
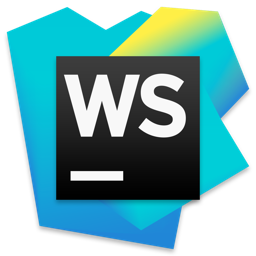
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
