Cloud Firestore Case-Insensitive Sorting with Query
Cloud Firestore requires both queries and filters to be case-sensitive, leaving developers to seek alternative approaches for case-insensitive sorting. To address this, a popular technique involves creating duplicate fields while maintaining case-insensitive copies of the data.
Creating a Case-Insensitive Field
By creating a secondary field with a lowercase version of the original data, sorting and filtering can be performed based on this case-insensitive field. For example, a field called myData could have a corresponding myData_insensitive field with all values converted to lowercase.
DocA: -> myData = 'AAA' -> myData_insensitive = 'aaa' DocB: -> myData = 'aaa' -> myData_insensitive = 'aaa' DocC: -> myData = 'BBB' -> myData_insensitive = 'bbb' DocD: -> myData = 'bbb' -> myData_insensitive = 'bbb'
Case-Folding for Unicode
Case-folding is recommended for Unicode support, as it handles complex character normalization and case-insensitive sorting. The following JavaScript snippet provides an example of how to implement case-folding:
<code class="javascript">caseFoldNormalize = function (s){ return s.normalize('NFKC').toLowerCase().toUpperCase().toLowerCase() };</code>
Sample Code
This code snippet demonstrates how to create a Firestore document with case-insensitive fields:
<code class="javascript">var raw_document = { name: "Los Angeles", state: "CA", country: "USA", structure: 'Waſſerſchloß', message: 'quıt quit' // Notice the different i's }; var field_options = { name: 'case_fold', country: 'case_fold', structure: 'case_fold', message: 'case_fold' } var firestore_document = caseFoldDoc(raw_document, field_options); db.collection("cities").doc("LA").set(firestore_document).then(function() { console.log("Document successfully written!"); }).catch(function(error) { console.error("Error writing document: ", error); });</code>
This will result in a document with both case-sensitive and case-insensitive fields:
<code class="javascript">{ "name": "Los Angeles", "state": "CA", "country": "USA", "structure": "Waſſerſchloß", "message": "quıt quit", "name_casefold": "los angeles", "country_casefold": "usa", "structure_casefold": "wasserschloss", "message_casefold": "quit quit" }</code>
Remember, while this technique offers a solution for case-insensitive sorting in Firestore, it requires extra storage space and incurs processing costs for every document update. As with any solution, consider the trade-offs and choose the approach that best aligns with your application requirements.
The above is the detailed content of How Can I Achieve Case-Insensitive Sorting in Cloud Firestore?. For more information, please follow other related articles on the PHP Chinese website!
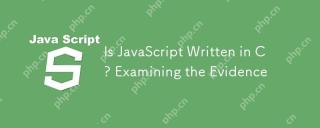
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
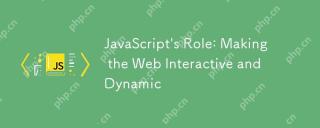
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
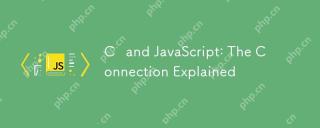
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
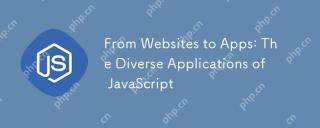
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
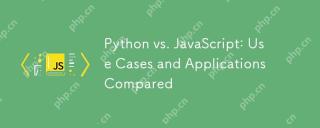
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
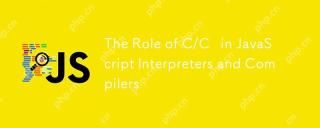
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
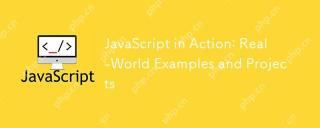
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
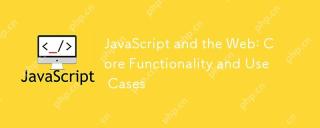
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor
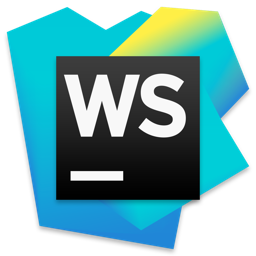
WebStorm Mac version
Useful JavaScript development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
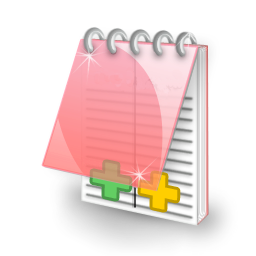
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
