


Bridging the Gap: Implementing SHA1 Encryption in Laravel, Despite BCrypt Limitations
Developing an Automatic Account Creator (AAC) for a game requires handling sensitive data securely, and encryption plays a crucial role in this process. However, when faced with server constraints that only support SHA1 encryption, leveraging the more secure BCrypt becomes challenging.
To address this dilemma, Laravel, with its adherence to IoC and Dependency Injection, offers a solution. By extending the Hash module, you can modify the default encryption mechanism to support SHA1.
Creating the SHAHasher Class
Within the app/libraries folder, create a SHAHasher class that implements the IlluminateHashingHasherInterface (or Illuminate/Contracts/Hashing/Hasher for Laravel 5). This class defines three essential methods:
- make(): Generates a hash using SHA1 encryption.
- check(): Compares a plain value with a hashed value using SHA1.
- needsRehash(): Confirms if the hash requires re-hashing with different options.
Example SHAHasher Class Code:
<code class="php">namespace App\Libraries; use Illuminate\Hashing\HasherInterface; class SHAHasher implements HasherInterface { public function make($value, array $options = []) { return hash('sha1', $value); } public function check($value, $hashedValue, array $options = []) { return $this->make($value) === $hashedValue; } public function needsRehash($hashedValue, array $options = []) { return false; } }</code>
Registering the Service Provider
To enable the SHAHasher class as the default hash component in Laravel, create a SHAHashServiceProvider class that extends IlluminateSupportServiceProvider. This class defines the registration process for the 'hash' service.
Example SHAHashServiceProvider Class Code:
<code class="php">namespace App\Libraries; use Illuminate\Support\ServiceProvider; class SHAHashServiceProvider extends ServiceProvider { public function register() { $this->app['hash'] = $this->app->share(function () { return new SHAHasher(); }); } public function provides() { return ['hash']; } }</code>
Adjusting the app.php Configuration
Finally, in app/config/app.php, modify the 'providers' array to replace the default 'IlluminateHashingHashServiceProvider' with 'SHAHashServiceProvider'. This ensures that your custom SHA1 encryption implementation is used throughout the Laravel application.
With these modifications, Laravel will now leverage SHA1 encryption seamlessly, allowing you to integrate it into your AAC while adhering to the encryption constraints of the remote server.
The above is the detailed content of How to Implement SHA1 Encryption in Laravel for Automatic Account Creation with Server Constraints?. For more information, please follow other related articles on the PHP Chinese website!
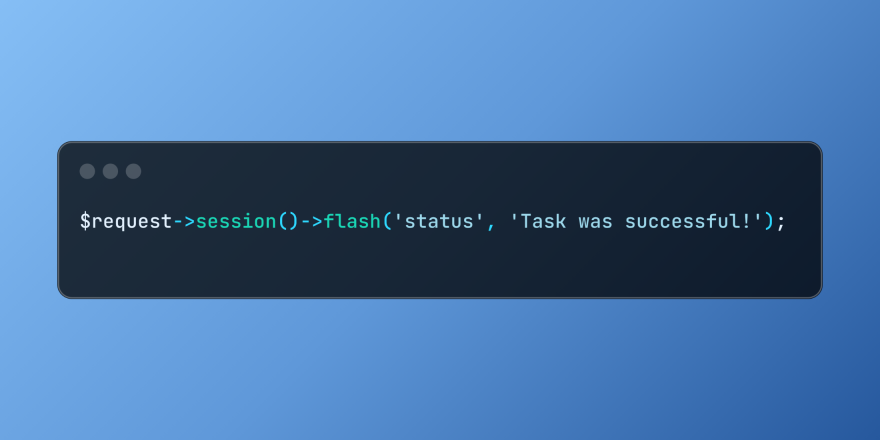
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
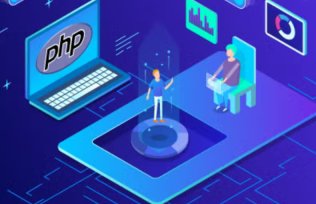
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
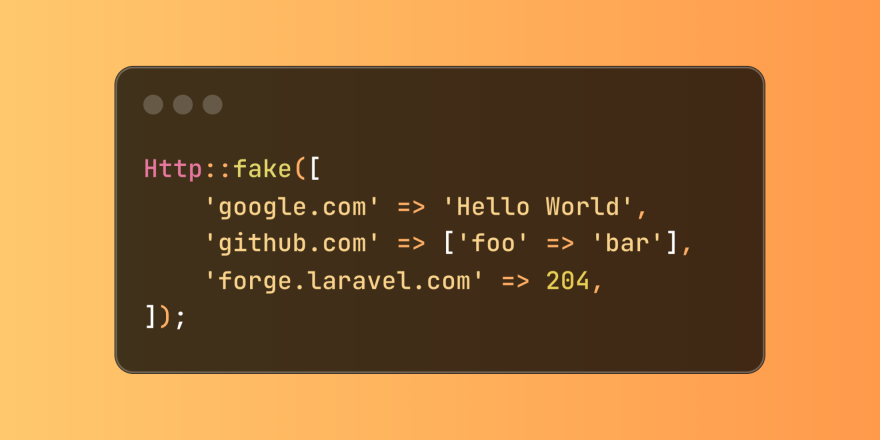
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
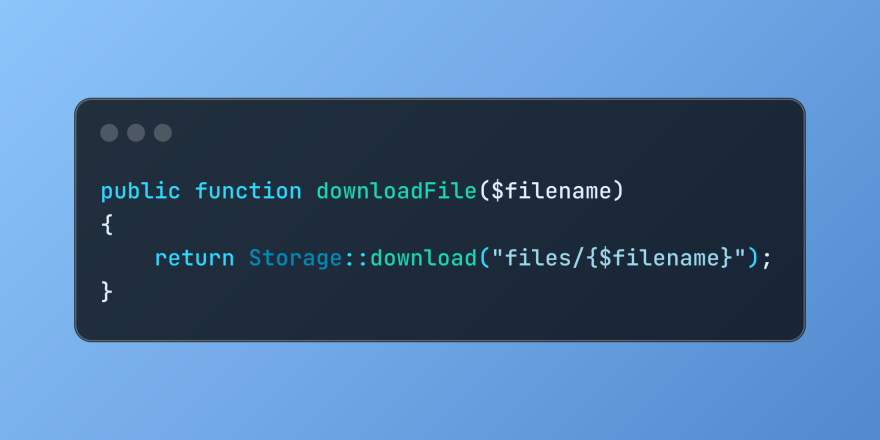
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
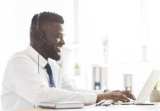
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
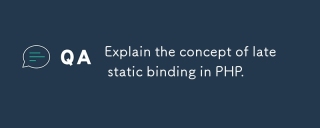
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
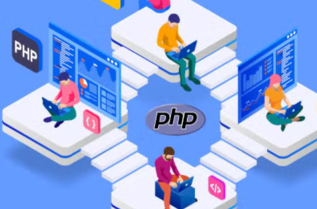
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
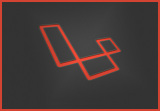
Laravel's service container and service providers are fundamental to its architecture. This article explores service containers, details service provider creation, registration, and demonstrates practical usage with examples. We'll begin with an ove


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
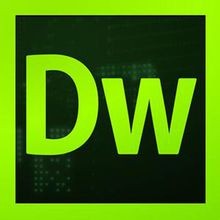
Dreamweaver CS6
Visual web development tools
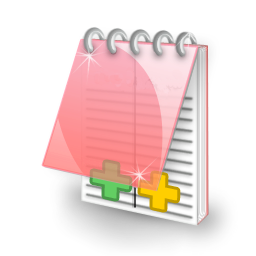
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
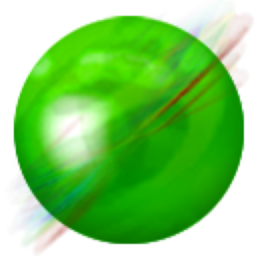
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
