


How to Merge Associative Arrays with Missing Columns and Provide Default Values?
Merging Associative Arrays with Missing Columns and Default Values
Given an array of associative arrays, the task is to combine them, merging the array keys and filling the missing columns with a default value.
<code class="php">$a = array('a' => 'some value', 'b' => 'some value', 'c' => 'some value'); $b = array('a' => 'another value', 'd' => 'another value', 'e' => 'another value', 'f' => 'another value'); $c = array('b' => 'some more value', 'x' => 'some more value', 'y' => 'some more value', 'z' => 'some more value'); $d = array($a, $b, $c);</code>
var_export($d)
will output:
array ( 0 => array ( 'a' => 'some value', 'b' => 'some value', 'c' => 'some value', ), 1 => array ( 'a' => 'another value', 'd' => 'another value', 'e' => 'another value', 'f' => 'another value', ), 2 => array ( 'b' => 'some more value', 'x' => 'some more value', 'y' => 'some more value', 'z' => 'some more value', ), )
The desired output is:
Array ( [0] => Array ( [a] => some value [b] => some value [c] => some value [d] => [e] => [f] => [x] => [y] => [z] => ) [1] => Array ( [a] => another value [b] => [c] => [d] => another value [e] => another value [f] => another value [x] => [y] => [z] => ) [2] => Array ( [a] => [b] => some more value [c] => [d] => [e] => [f] => [x] => some more value [y] => some more value [z] => some more value ) )
To achieve this, array_merge() can be used.
<code class="php">$keys = array(); foreach(new RecursiveIteratorIterator(new RecursiveArrayIterator($d)) as $key => $val) $keys[$key] = ''; $data = array(); foreach($d as $values) { $data[] = array_merge($keys, $values); } echo '<pre class="brush:php;toolbar:false">'; print_r($data);
An alternative method is to create key pair values and then map each $d and merge.
<code class="php">$keys = array_keys(call_user_func_array('array_merge', $d)); $key_pair = array_combine($keys, array_fill(0, count($keys), null)); $values = array_map(function($e) use ($key_pair) { return array_merge($key_pair, $e); }, $d);</code>
The above is the detailed content of How to Merge Associative Arrays with Missing Columns and Provide Default Values?. For more information, please follow other related articles on the PHP Chinese website!
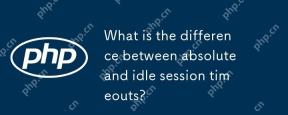
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
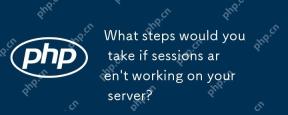
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
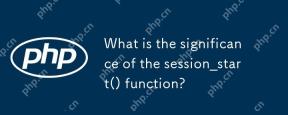
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.
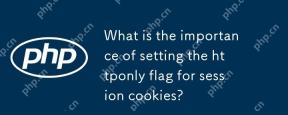
Setting the httponly flag is crucial for session cookies because it can effectively prevent XSS attacks and protect user session information. Specifically, 1) the httponly flag prevents JavaScript from accessing cookies, 2) the flag can be set through setcookies and make_response in PHP and Flask, 3) Although it cannot be prevented from all attacks, it should be part of the overall security policy.
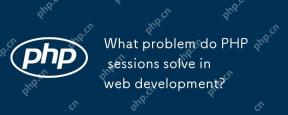
PHPsessionssolvetheproblemofmaintainingstateacrossmultipleHTTPrequestsbystoringdataontheserverandassociatingitwithauniquesessionID.1)Theystoredataserver-side,typicallyinfilesordatabases,anduseasessionIDstoredinacookietoretrievedata.2)Sessionsenhances
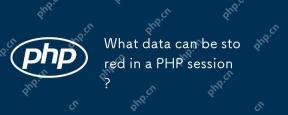
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
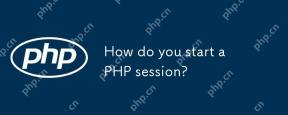
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
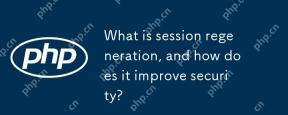
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
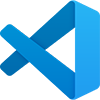
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
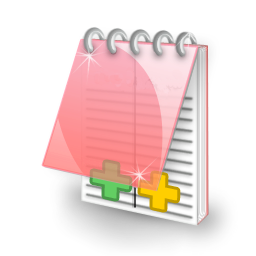
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
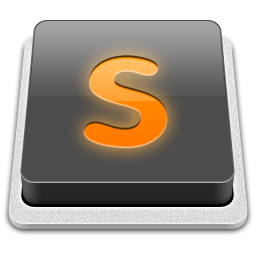
SublimeText3 Mac version
God-level code editing software (SublimeText3)
