Converting Comma-Delimited Strings to Lists in Python
When working with textual data, it's often necessary to extract specific values from strings. Comma-separated values (CSVs) are a common format for storing multiple values in a single string. To leverage CSVs effectively, you need to be able to convert them into more manageable data structures, such as lists.
Problem Statement
Convert a comma-delimited string into a Python list, transforming values like "A,B,C,D,E" into ['A', 'B', 'C', 'D', 'E'].
Solution Using str.split
The str.split method provides a convenient way to split a string by a given delimiter, in this case, a comma. The resulting list contains individual elements that were originally separated by commas.
<code class="python">mStr = 'A,B,C,D,E' mList = mStr.split(",") print(mList) # Output: ['A', 'B', 'C', 'D', 'E']</code>
Converting to Tuples
If you wish to create a tuple instead of a list, simply apply the tuple() function to the split result:
<code class="python">myTuple = tuple(mList) print(myTuple) # Output: ('A', 'B', 'C', 'D', 'E')</code>
Appending to an Existing List
To append comma-separated values to an existing list, use the append() method:
<code class="python">myList = ['A', 'B', 'C'] myList.append('D,E') print(myList) # Output: ['A', 'B', 'C', 'D,E']</code>
Alternatively, you can use the extend() method to append each individual value:
<code class="python">myList.extend(['D', 'E']) print(myList) # Output: ['A', 'B', 'C', 'D', 'E']</code>
Now you have the tools to efficiently convert comma-delimited strings into Python lists, tuples, or extend existing lists with new values.
The above is the detailed content of How to Convert Comma-Delimited Strings to Lists in Python?. For more information, please follow other related articles on the PHP Chinese website!
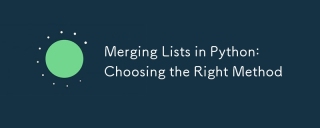
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
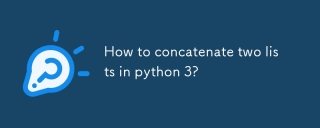
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
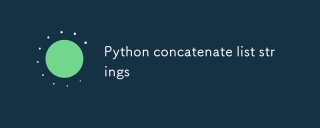
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
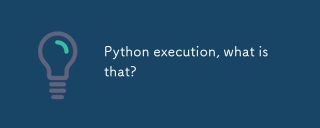
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
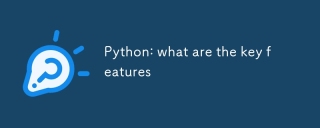
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
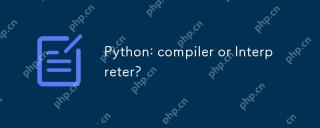
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
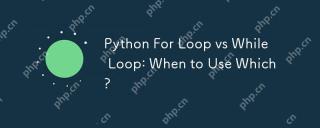
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
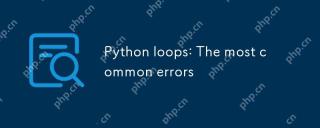
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
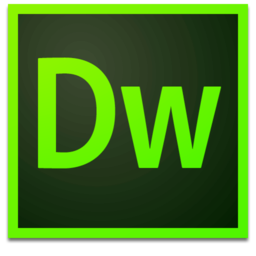
Dreamweaver Mac version
Visual web development tools
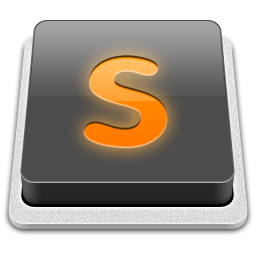
SublimeText3 Mac version
God-level code editing software (SublimeText3)
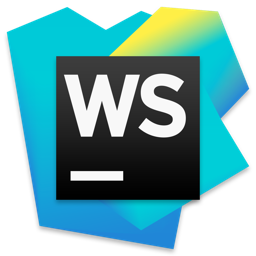
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
