Efficient Array Validation for Integer Content
In PHP, verifying whether an array exclusively contains integers can be achieved through various approaches. While manual iteration and conditional checks are a viable option, there exists a more concise solution utilizing native PHP functionality.
Filtering with array_filter
The array_filter function offers a convenient and efficient method for this task. It filters an array based on a provided callback function, returning a new array containing only the elements that satisfy the condition. In our case, we can employ the built-in is_int function as the callback to isolate integer elements:
<code class="php">$only_integers === array_filter($only_integers, 'is_int'); // true $letters_and_numbers === array_filter($letters_and_numbers, 'is_int'); // false</code>
Abstraction with Higher-Order Functions
To enhance code readability and reusability, we can define helper functions that generalize our validation process. These functions leverage higher-order functions, which operate on functions as arguments, providing greater flexibility.
<code class="php">// Check if all array elements pass the predicate function all($elems, $predicate) { foreach ($elems as $elem) { if (!call_user_func($predicate, $elem)) { return false; } } return true; } // Check if any array element passes the predicate function any($elems, $predicate) { foreach ($elems as $elem) { if (call_user_func($predicate, $elem)) { return true; } } return false; }</code>
With these helpers, our original validation becomes a concise and declarative expression:
<code class="php">all($only_integers, 'is_int'); // true any($letters_and_numbers, 'is_int'); // false</code>
Simplified Invocation
To further simplify the validation process, we can encapsulate the helpers within a custom function that accepts an array and returns a boolean flag:
<code class="php">function array_has_only_ints($array) { return all($array, 'is_int'); }</code>
This function provides a con
The above is the detailed content of How to Validate PHP Arrays for Integer Content Effectively?. For more information, please follow other related articles on the PHP Chinese website!
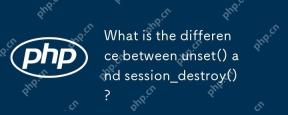
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
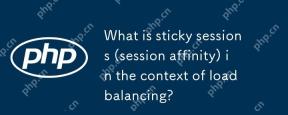
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
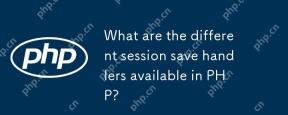
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
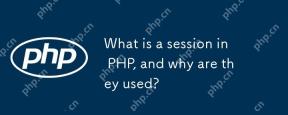
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
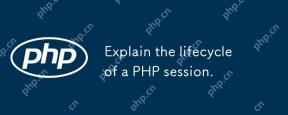
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
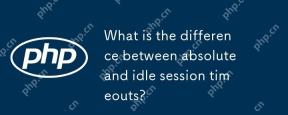
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
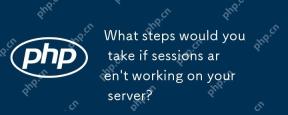
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
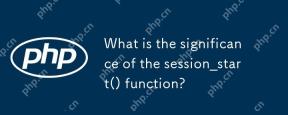
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
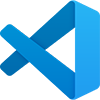
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
