Python lists are one of the most fundamental and versatile data structures in programming. They allow you to store and manage collections of data efficiently. In this article, we’ll dive into what lists are, how to use them, and some common operations and examples.
What is a Python List?
A list in Python is an ordered collection of elements, enclosed in square brackets []. Lists can contain elements of different types, such as integers, strings, floats, or even other lists. The best part? Lists are mutable, meaning their contents can be modified.
# Example of a list with integers numbers = [1, 2, 3, 4, 5] # Example of a list with mixed data types mixed_list = [1, "hello", 3.14, True]
How to Access List Elements
You can access elements of a list by using their index. Python uses zero-based indexing, meaning the first element is accessed with an index of 0.
print(numbers[0]) # Output: 1 print(mixed_list[1]) # Output: "hello"
Modifying List Elements
Since lists are mutable, you can modify an element at a specific index:
numbers[2] = 10 print(numbers) # Output: [1, 2, 10, 4, 5]
Adding Elements to a List
You can easily add elements to a list using methods like append() and insert():
# Using append to add an element to the end numbers.append(6) print(numbers) # Output: [1, 2, 10, 4, 5, 6] # Using insert to add an element at a specific index numbers.insert(1, 20) print(numbers) # Output: [1, 20, 2, 10, 4, 5, 6]
Removing Elements from a List
Python provides several ways to remove elements from a list:
- remove(): Removes the first occurrence of a specified value.
- pop(): Removes the last element or the element at a specified index.
- del: Deletes an element at a specified index.
numbers.remove(20) print(numbers) # Output: [1, 2, 10, 4, 5, 6] numbers.pop(2) # Removes element at index 2 print(numbers) # Output: [1, 2, 4, 5] del numbers[1] # Deletes element at index 1 print(numbers) # Output: [1, 4, 5]
Slicing a List
You can create a sublist from a list using slicing:
subset = numbers[1:3] print(subset) # Output: [4, 5]
List Comprehension
List comprehension offers a concise way to create lists:
doubled = [x * 2 for x in numbers] print(doubled) # Output: [2, 8, 10]
Checking for Element Existence
You can check if an item exists in a list using the in keyword:
print(4 in numbers) # Output: True
Common List Methods
- len(list): Returns the number of elements.
- sort(): Sorts the list in place.
- reverse(): Reverses the list in place.
numbers = [3, 1, 4, 1, 5, 9] numbers.sort() print(numbers) # Output: [1, 1, 3, 4, 5, 9] numbers.reverse() print(numbers) # Output: [9, 5, 4, 3, 1, 1] print(len(numbers)) # Output: 6
Conclusion
Python lists are a powerful tool for managing collections of data. Whether you need to store numbers, strings, or more complex objects, lists provide the flexibility and functionality to meet your needs. From adding, removing, and modifying elements to slicing and using list comprehension, there are countless ways to work with lists in Python.
With the examples and tips provided, you should now have a solid understanding of how to create and manipulate lists effectively in Python.
The above is the detailed content of Python Lists Explained: A Beginners Guide with Examples. For more information, please follow other related articles on the PHP Chinese website!
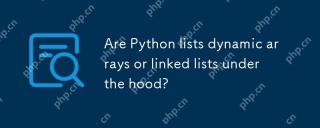
Pythonlistsareimplementedasdynamicarrays,notlinkedlists.1)Theyarestoredincontiguousmemoryblocks,whichmayrequirereallocationwhenappendingitems,impactingperformance.2)Linkedlistswouldofferefficientinsertions/deletionsbutslowerindexedaccess,leadingPytho
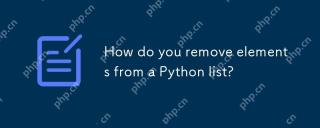
Pythonoffersfourmainmethodstoremoveelementsfromalist:1)remove(value)removesthefirstoccurrenceofavalue,2)pop(index)removesandreturnsanelementataspecifiedindex,3)delstatementremoveselementsbyindexorslice,and4)clear()removesallitemsfromthelist.Eachmetho
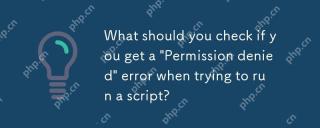
Toresolvea"Permissiondenied"errorwhenrunningascript,followthesesteps:1)Checkandadjustthescript'spermissionsusingchmod xmyscript.shtomakeitexecutable.2)Ensurethescriptislocatedinadirectorywhereyouhavewritepermissions,suchasyourhomedirectory.
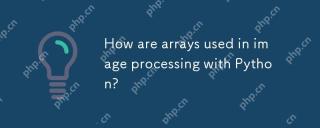
ArraysarecrucialinPythonimageprocessingastheyenableefficientmanipulationandanalysisofimagedata.1)ImagesareconvertedtoNumPyarrays,withgrayscaleimagesas2Darraysandcolorimagesas3Darrays.2)Arraysallowforvectorizedoperations,enablingfastadjustmentslikebri
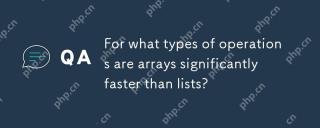
Arraysaresignificantlyfasterthanlistsforoperationsbenefitingfromdirectmemoryaccessandfixed-sizestructures.1)Accessingelements:Arraysprovideconstant-timeaccessduetocontiguousmemorystorage.2)Iteration:Arraysleveragecachelocalityforfasteriteration.3)Mem
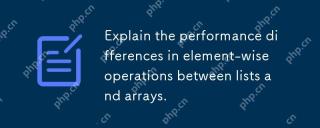
Arraysarebetterforelement-wiseoperationsduetofasteraccessandoptimizedimplementations.1)Arrayshavecontiguousmemoryfordirectaccess,enhancingperformance.2)Listsareflexiblebutslowerduetopotentialdynamicresizing.3)Forlargedatasets,arrays,especiallywithlib
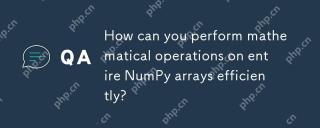
Mathematical operations of the entire array in NumPy can be efficiently implemented through vectorized operations. 1) Use simple operators such as addition (arr 2) to perform operations on arrays. 2) NumPy uses the underlying C language library, which improves the computing speed. 3) You can perform complex operations such as multiplication, division, and exponents. 4) Pay attention to broadcast operations to ensure that the array shape is compatible. 5) Using NumPy functions such as np.sum() can significantly improve performance.
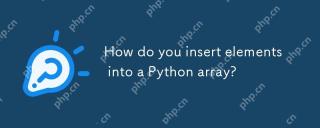
In Python, there are two main methods for inserting elements into a list: 1) Using the insert(index, value) method, you can insert elements at the specified index, but inserting at the beginning of a large list is inefficient; 2) Using the append(value) method, add elements at the end of the list, which is highly efficient. For large lists, it is recommended to use append() or consider using deque or NumPy arrays to optimize performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
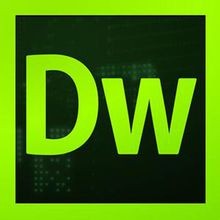
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
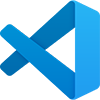
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
