Alright, let’s get real for a second. Security is a big deal, and if you’re building APIs, you can’t just let anyone waltz in and start messing with your data. That’s where JWT (JSON Web Tokens) comes in to save the day. Today, we’re leveling up our Go API by adding JWT-based authentication.
A Quick Heads-Up ?
If you’ve been using the old github.com/dgrijalva/jwt-go package, it’s time for an upgrade. The new standard is github.com/golang-jwt/jwt/v4.
Why the switch?
- The original author handed over the reins, and the new maintainers have been busy making improvements and fixing security issues.
- Starting from version 4.0.0, they added Go module support and improved token validation.
- Check out their MIGRATION_GUIDE.md if you're still using the old package.
Now, let’s get started with our fancy new JWT library!
What’s JWT Again? ?
For those new to JWT:
- It’s like a signed permission slip for accessing your API.
- The API generates a token, signs it, and the client (user, app, etc.) includes that token in every request.
- The server checks the token and says, "Yup, you’re legit."
Now that you’re up to speed, let’s dive into the code!
Setting Up the Project
We’re continuing from where we left off in last post. Let’s update our Go module and install the necessary packages:
- Add the JWT package and mux router:
go get github.com/golang-jwt/jwt/v4 go get github.com/gorilla/mux
- Open your main.go file, and let’s get coding!
Step 1: Generate a JWT Token
First, we’ll create a function that generates a JWT token when a user logs in. This token will contain the username and will be signed using a secret key.
var jwtKey = []byte("my_secret_key") type Credentials struct { Username string `json:"username"` Password string `json:"password"` } type Claims struct { Username string `json:"username"` jwt.RegisteredClaims } func generateToken(username string) (string, error) { expirationTime := time.Now().Add(5 * time.Minute) claims := &Claims{ Username: username, RegisteredClaims: jwt.RegisteredClaims{ ExpiresAt: jwt.NewNumericDate(expirationTime), }, } token := jwt.NewWithClaims(jwt.SigningMethodHS256, claims) tokenString, err := token.SignedString(jwtKey) return tokenString, err }
This function generates a token that expires after 5 minutes, signed using the HS256 algorithm.
Step 2: Create the Login Endpoint
Next, we’ll build a login endpoint where users send their credentials. If the login info checks out, we’ll generate a JWT and send it back in a cookie.
func login(w http.ResponseWriter, r *http.Request) { var creds Credentials err := json.NewDecoder(r.Body).Decode(&creds) if err != nil { w.WriteHeader(http.StatusBadRequest) return } if creds.Username != "admin" || creds.Password != "password" { w.WriteHeader(http.StatusUnauthorized) return } token, err := generateToken(creds.Username) if err != nil { w.WriteHeader(http.StatusInternalServerError) return } http.SetCookie(w, &http.Cookie{ Name: "token", Value: token, Expires: time.Now().Add(5 * time.Minute), }) }
Step 3: Middleware for JWT Validation
Now, we need a middleware function to validate JWT tokens before allowing access to protected routes.
func authenticate(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { c, err := r.Cookie("token") if err != nil { if err == http.ErrNoCookie { w.WriteHeader(http.StatusUnauthorized) return } w.WriteHeader(http.StatusBadRequest) return } tokenStr := c.Value claims := &Claims{} tkn, err := jwt.ParseWithClaims(tokenStr, claims, func(token *jwt.Token) (interface{}, error) { return jwtKey, nil }) if err != nil || !tkn.Valid { w.WriteHeader(http.StatusUnauthorized) return } next.ServeHTTP(w, r) }) }
This middleware checks if the request has a valid JWT token. If not, it returns an unauthorized response.
Step 4: Protecting Routes
Now, let’s apply our authenticate middleware to protect the /books route:
func main() { r := mux.NewRouter() r.HandleFunc("/login", login).Methods("POST") r.Handle("/books", authenticate(http.HandlerFunc(getBooks))).Methods("GET") fmt.Println("Server started on port :8000") log.Fatal(http.ListenAndServe(":8000", r)) }
Testing the API
- Login to generate a token:
curl -X POST http://localhost:8000/login -d '{"username":"admin", "password":"password"}' -H "Content-Type: application/json"
- Access the protected /books endpoint:
curl --cookie "token=<your_token>" http://localhost:8000/books </your_token>
If the token is valid, you’ll get access. If not, you’ll get a "401 Unauthorized."
What’s Next?
Next time, we’ll connect our API to a database to manage user credentials and store data. Stay tuned for more!
The above is the detailed content of Securing Your Go API with JWT Authentication. For more information, please follow other related articles on the PHP Chinese website!
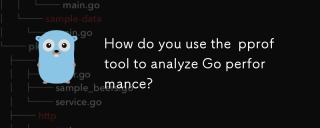
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
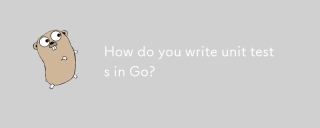
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
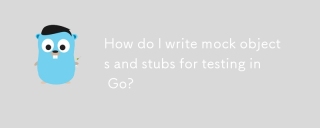
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
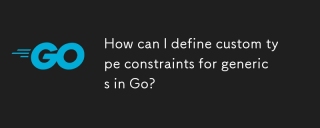
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
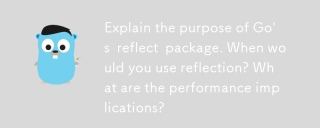
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
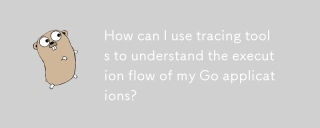
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
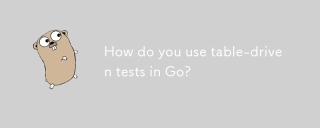
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
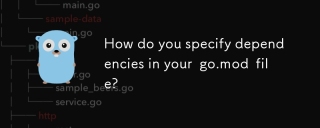
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
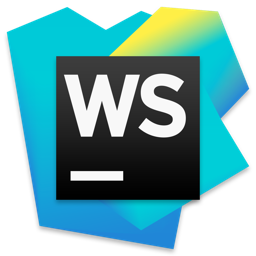
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
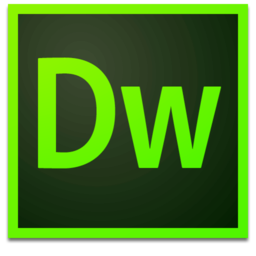
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
