What are the use cases and best practices for smart pointers in C++?
Smart pointers in C++ are used to manage dynamically allocated memory, prevent memory leaks and wild pointers, and improve code security. Use cases include preventing memory leaks, avoiding wild pointers, managing shared ownership, and exception safety. Best practices include using appropriate smart pointer types, following Rule 5, avoiding circular references, being careful with copies and assignments, and controlling destruction order.
Use cases and best practices of smart pointers in C++
Smart pointers are a method used to manage dynamic allocation in C++ A unique pointer to the object in memory. They help eliminate memory leaks and wild pointer issues, making your code more robust and secure.
Use cases
- Prevent memory leaks: Smart pointers ensure that memory is automatically released when the object goes out of scope.
- Avoid wild pointers: Smart pointers always point to a valid object to prevent programs from accessing invalid memory.
- Manage shared ownership: Smart pointers can share access to objects across threads and classes, simplifying memory management.
- Exception safety: Smart pointers ensure that memory is released when an object throws an exception, thus preventing memory leaks.
Best Practices
- Use appropriate smart pointer types: There are 4 main smart pointer types (unique_ptr , shared_ptr, weak_ptr, auto_ptr), each type has different ownership semantics. Choosing a type that fits your use case is critical.
- Follow Rule 5: The number of smart pointers pointing to an object must not exceed 5. This helps prevent circular references and memory leaks.
- Avoid circular references: Two or more objects pointing to each other will create circular references, leading to memory leaks. Use weak_ptr to break reference cycles.
- Be careful with copies and assignments: When you copy or assign a smart pointer, the ownership rules are also passed along. Use appropriate patterns (e.g. copy constructors, move semantics) to properly handle ownership.
- Destruction order control: Use a custom destructor to control the order of smart pointer destruction to avoid accidentally releasing objects.
Practical case
// 不使用智能指针的示例 int* ptr = new int; *ptr = 10; // 使用该指针 delete ptr; // 手动释放内存 // 使用 unique_ptr 的示例 std::unique_ptr<int> ptr(new int); *ptr = 10; // 使用该指针 // ptr 超出作用域后自动释放内存
Notes
- Smart pointers increase overhead and should be used with caution .
- Abuse of smart pointers can cause performance problems because it requires additional indirect references.
- The type of smart pointers should be chosen carefully to avoid overuse or underuse and to ensure proper resource management.
The above is the detailed content of What are the use cases and best practices for smart pointers in C++?. For more information, please follow other related articles on the PHP Chinese website!
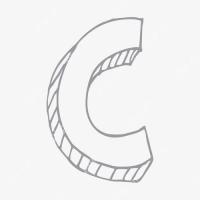
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
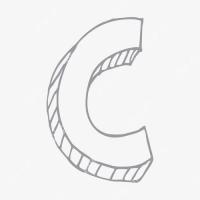
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
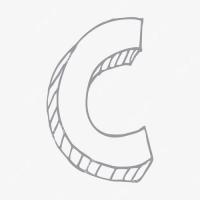
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
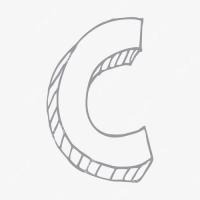
To implement loose coupling design in C, you can use the following methods: 1. Use interfaces, such as defining the Logger interface and implementing FileLogger and ConsoleLogger; 2. Dependency injection, such as the DataAccess class receives Database pointers through the constructor; 3. Observer mode, such as the Subject class notifies ConcreteObserver and AnotherObserver. Through these technologies, dependencies between modules can be reduced and code maintainability and flexibility can be improved.
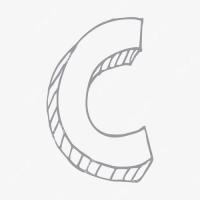
Exception-neutral code refers to a snippet of code that neither throws nor handles exceptions. In C programming, applying exception neutral code can simplify exception handling logic and improve code maintainability and reliability.
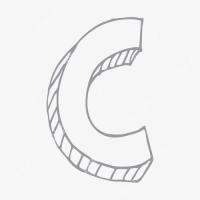
C templates are used to implement generic programming, allowing for the writing of general code. 1) Define template functions, such as max functions, which are suitable for any type. 2) Create template classes, such as general container classes. 3) Pay attention to template instantiation, compilation time, template specialization, debugging and error information. 4) Follow best practices, keep the code simple, and consider using constraint template parameters.
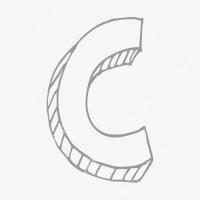
Implementing lock-free data structures in C can be achieved by using atomic operations and CAS operations. The specific steps include: 1. Use std::atomic to ensure the atomic operation of head and tail; 2. Use compare_exchange_strong to perform CAS operations to ensure data consistency; 3. Use std::shared_ptr to manage node data to avoid memory leakage.
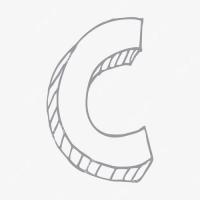
The main steps and precautions for using string streams in C are as follows: 1. Create an output string stream and convert data, such as converting integers into strings. 2. Apply to serialization of complex data structures, such as converting vector into strings. 3. Pay attention to performance issues and avoid frequent use of string streams when processing large amounts of data. You can consider using the append method of std::string. 4. Pay attention to memory management and avoid frequent creation and destruction of string stream objects. You can reuse or use std::stringstream.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
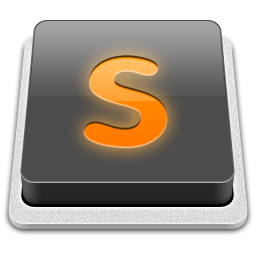
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!
