How to use MPI to implement distributed multi-threading in C++?
The method to use MPI to implement distributed multi-threading is as follows: Specify the multi-threading level: When initializing the MPI environment, use MPI_Init_thread() to specify the thread level (such as MPI_THREAD_MULTIPLE). Create threads: Use the standard std::thread mechanism to create threads, but use MPI thread-safe functions for MPI communication. Distribution tasks: Distribute data to different MPI processes and threads for parallel computation.
How to use MPI to implement distributed multi-threading in C++
Introduction
MPI (Message Passing Interface) is a widely used programming model for writing distributed parallel programs. It allows programmers to use message passing mechanisms to execute code in parallel on multiple computers, enabling high-performance computing. In addition to distributed parallelism, MPI also supports multi-threaded programming, which can further improve code efficiency. This article will introduce how to use MPI to implement distributed multi-threading in C++, and provide practical cases for demonstration.
MPI Multithreaded Programming
MPI_THREAD_* Options
The MPI specification defines the following options to specify the multithreading level of a program :
-
MPI_THREAD_SINGLE
: The program will use only one thread. -
MPI_THREAD_FUNNELED
: All MPI calls of the program will be serialized, allowing only one thread to execute MPI calls at the same time. -
MPI_THREAD_SERIALIZED
: The program's MPI calls will be serialized and can only be made by the main thread. -
MPI_THREAD_MULTIPLE
: The program can make MPI calls in parallel and can use multiple threads.
Initialize MPI environment
To use multi-threading in MPI programs, you need to specify the thread level when initializing the MPI environment. This can be done with the following code:
int provided; MPI_Init_thread(&argc, &argv, MPI_THREAD_MULTIPLE, &provided);
Parameters provided
Indicates the level of multithreading provided by the MPI library. If provided
is equal to MPI_THREAD_MULTIPLE
, it indicates that the MPI library supports multi-threaded programming.
Creating threads
Using std::thread
The standard method of creating threads is also available in MPI programs, but requires additional considerations. To ensure that MPI calls are synchronized correctly across threads, MPI thread-safe functions are required for MPI communication.
The following is an example of creating a thread:
std::thread thread([&]() { // 在新线程中执行 MPI 调用 });
Practical case
Now let’s look at a practical case to demonstrate how to use MPI multi-thread acceleration Matrix multiplication calculation.
Matrix multiplication
Given two matrices A
and B
, where the size of A
is m x n
, B
has size n x p
, and the result of matrix multiplication C = A * B
has size C
m x p.
MPI Parallelization
Using MPI to parallelize matrix multiplication calculations, you can assign the rows of theA matrix to different MPI processes and let each A process computes the product of a local submatrix and the
B matrix.
Multi-thread acceleration
In each MPI process, multi-threading can be used to further accelerate calculations. Assign the columns of theB matrix to different threads, making each thread responsible for computing the product of the local submatrix and a column of the
B matrix.
// MPI 主程序 int main(int argc, char** argv) { // 初始化 MPI 环境 int provided; MPI_Init_thread(&argc, &argv, MPI_THREAD_MULTIPLE, &provided); // 创建 MPI 通信器 MPI_Comm comm = MPI_COMM_WORLD; int rank, size; MPI_Comm_rank(comm, &rank); MPI_Comm_size(comm, &size); // 分配矩阵行并广播矩阵 B ... // 创建线程池 std::vector<std::thread> threads; // 计算局部子矩阵乘积 for (int i = 0; i < columns_per_thread; i++) { threads.push_back(std::thread([&, i]() { ... })); } // 等待所有线程完成 for (auto& thread : threads) { thread.join(); } // 汇总局部结果并输出 C 矩阵 ... // 结束 MPI 环境 MPI_Finalize(); return 0; }
conclusion
By using MPI multithreading, you can combine the advantages of distributed parallelism and multithreaded programming to significantly improve the performance of C++ programs. The above practical case shows how to apply MPI multithreading to matrix multiplication calculations to parallelize and accelerate the calculation process.The above is the detailed content of How to use MPI to implement distributed multi-threading in C++?. For more information, please follow other related articles on the PHP Chinese website!
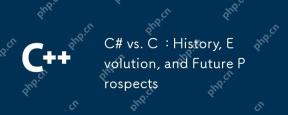
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
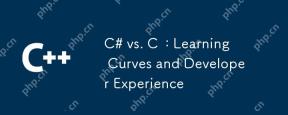
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
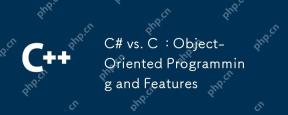
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
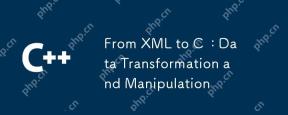
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
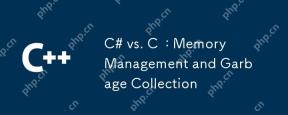
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
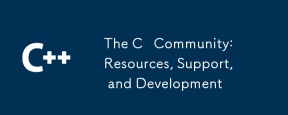
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
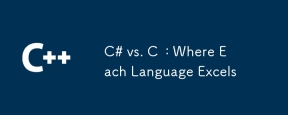
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
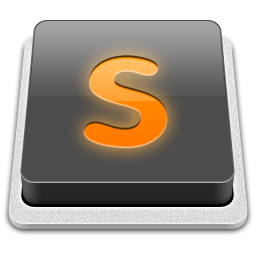
SublimeText3 Mac version
God-level code editing software (SublimeText3)
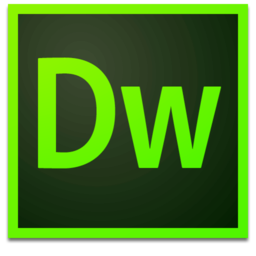
Dreamweaver Mac version
Visual web development tools
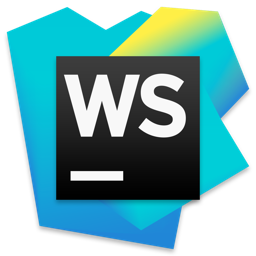
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment