C++ Lambda expressions can easily define anonymous functions and allow access to external variables. The syntax is: [capture-list] (parameter-list) -> return-type { body-statement; }. Practical examples include using lambda expressions to sort containers, handle GUI events, and perform data processing. The advantages are high readability, reusability, and expressiveness.
Application of C++ Lambda expressions in actual projects
Introduction
Lambda expression is a kind of application A convenient way to define anonymous functions. In C++, they are represented using closure syntax, which allows access to variables outside the function.
Syntax
[capture-list] (parameter-list) -> return-type { body-statement; }
- capture-list: Specify the external variables that the lambda expression can access.
- parameter-list: The parameter list received by the lambda expression.
- return-type: The return type of lambda expression.
- body-statement: The code block for lambda expression execution.
Practical case
1. Sorting container
We can use lambda expressions to define a sorting condition, In order to use std::sort
to sort the container:
std::vector<int> numbers = {1, 3, 5, 2, 4}; std::sort(numbers.begin(), numbers.end(), [](int a, int b) { return a < b; });
2. Event handling
GUI frameworks usually use lambda expressions to handle events :
button.onClick([this] { /* 处理按钮点击事件 */ });
3. Data processing
Lambda expressions can be used to process data structures:
std::vector<std::string> names = {"John", "Mary", "Bob"}; std::transform(names.begin(), names.end(), names.begin(), [](std::string& name) { return name.substr(0, 1).toUpper() + name.substr(1); });
Advantages
The advantages of using C++ lambda expressions include:
- High readability (reduces code redundancy)
- Reusability (can be easily used in different places Transfer function)
- Strong expressive ability (allows access to external variables and definition of arbitrarily complex functions)
The above is the detailed content of How to apply C++ Lambda expressions in actual projects?. For more information, please follow other related articles on the PHP Chinese website!
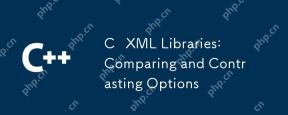
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
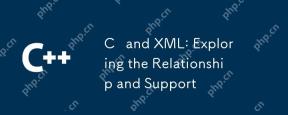
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
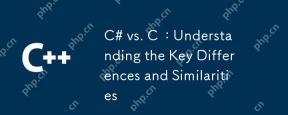
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
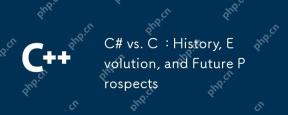
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
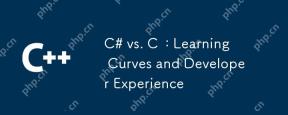
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
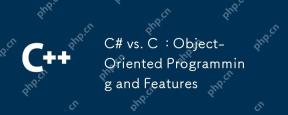
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
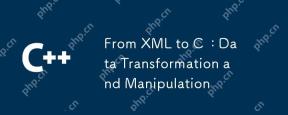
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
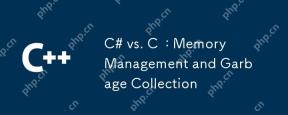
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
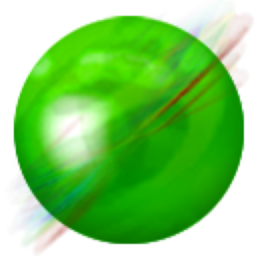
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
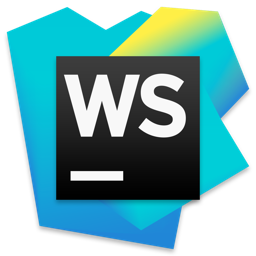
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor