The key role of function pointers in C++ code is to improve extensibility and allow functions to be called without specifying the function name. Its usage includes declaring, allocating, and calling function pointers. Function pointers play a vital role in sorting algorithms by passing different comparators to implement multiple sorting methods. This makes C++ code more flexible and reusable, greatly improving code quality.
The key role of function pointers in C++ code extensibility
A function pointer is a pointer to a function that allows Call a function without specifying a function name. This is useful when highly flexible and scalable code is required.
Usage
To declare a function pointer, use the following syntax:
type (*function_pointer_name)(arguments);
For example, declare a pointer to a function that takes two int parameters and returns an int Pointer to a function:
int (*func_ptr)(int, int);
To assign a function pointer to a function, use the function address operator (&):
func_ptr = &my_function;
Calling a function pointer is like calling a normal function:
int result = func_ptr(1, 2);
Practical case
Sort algorithm
Consider a sorting algorithm that needs to sort an array based on a specific comparator. Using function pointers, you can easily pass different comparators to the sorting algorithm, allowing for multiple sorting methods.
typedef int (*Comparator)(const int&, const int&); void Sort(int* array, int size, Comparator comparator) { for (int i = 0; i < size; ++i) { for (int j = i + 1; j < size; ++j) { if (comparator(array[i], array[j])) { swap(array[i], array[j]); } } } } int Ascending(const int& a, const int& b) { return a < b; } int Descending(const int& a, const int& b) { return a > b; } int main() { int array[] = {5, 2, 8, 3, 1}; int size = sizeof(array) / sizeof(array[0]); Sort(array, size, Ascending); for (int num : array) { cout << num << " "; } cout << endl; Sort(array, size, Descending); for (int num : array) { cout << num << " "; } return 0; }
In this example, the Sort
function accepts a Comparator
function pointer that defines how to compare two elements. The Ascending
and Descending
functions are two comparators used to sort arrays in ascending and descending order respectively.
Conclusion
Function pointers are an extensibility tool in C++ that can greatly improve code quality by making the code more flexible and reusable. By understanding the use of function pointers, you can take full advantage of the power of C++ to build complex and maintainable applications.
The above is the detailed content of The key role of function pointers in C++ code extensibility. For more information, please follow other related articles on the PHP Chinese website!
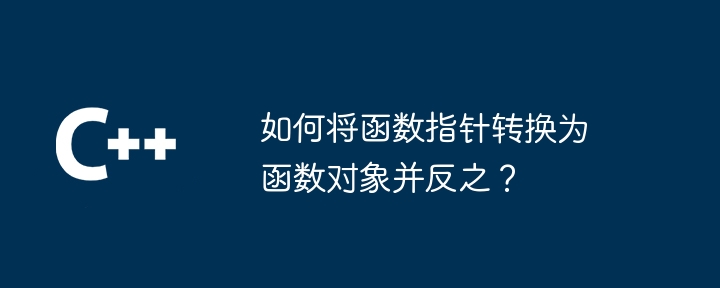
在C++中,通过std::function模板可将函数指针转换为函数对象:使用std::function将函数指针包装成函数对象。使用std::function::target成员函数将函数对象转换为函数指针。此转换在事件处理、函数回调和泛型算法等场景中很有用,提供了更大的灵活性和代码重用性。
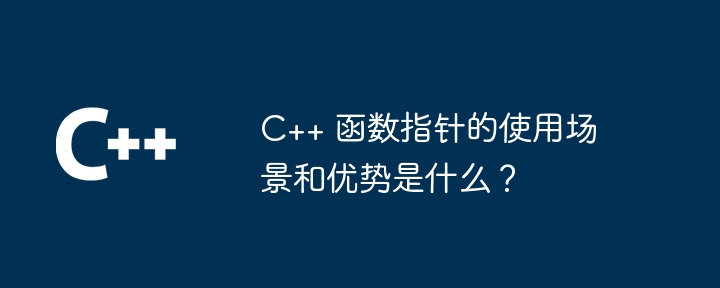
函数指针允许存储对函数的引用,提供额外的灵活性。使用场景包括事件处理、算法排序、数据转换和动态多态。优势包括灵活性、解耦、代码重用和性能优化。实际应用包括事件处理、算法排序和数据转换。凭借函数指针,C++程序员可以创建灵活且动态的代码。
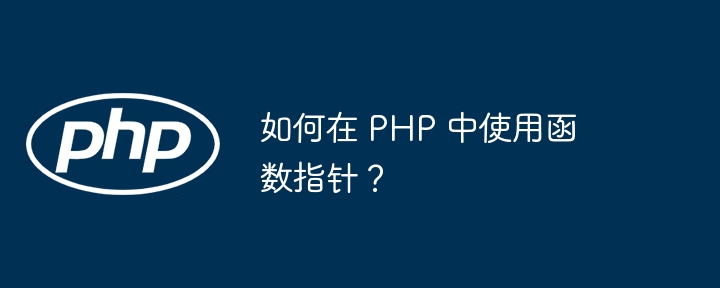
在PHP中,函数指针是称为回调函数的变量,指向函数地址。它允许动态处理函数:语法:$functionPointer='function_name'实战案例:对数组执行操作:usort($numbers,'sortAscending')作为函数参数:array_map(function($string){...},$strings)注意:函数指针指向函数名称,必须与指定的类型匹配,并确保指向的函数始终存在。
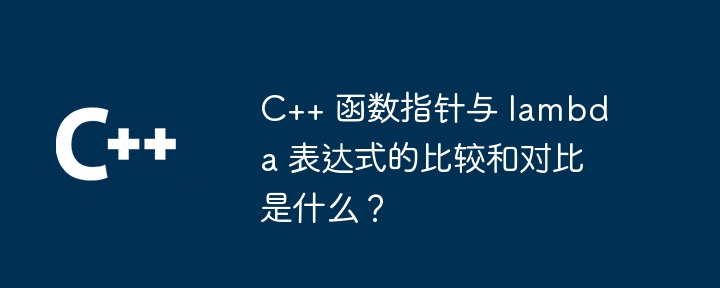
函数指针和Lambda表达式都是C++中封装代码块的技术,各有不同。函数指针是指向函数内存地址的常量指针,而Lambda表达式是匿名函数,语法更灵活,可捕获外部变量。函数指针适合类型安全和低开销的场景,Lambda表达式适合需要匿名性和捕获外部变量的场景。
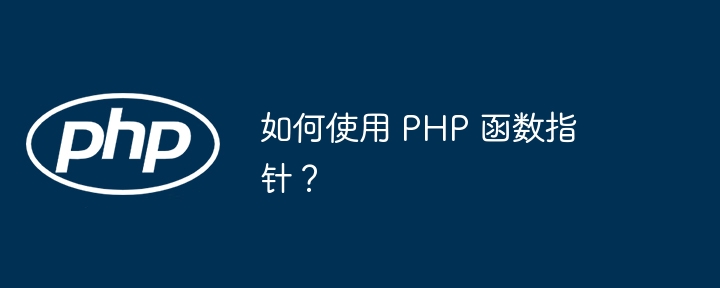
PHP函数指针允许将函数作为参数传递,可用于创建回调函数或重用代码。语法:$functionPointer=function_name;或匿名函数:$functionPointer=function($arg1,$arg2){...};通过call_user_func($function,$a,$b)调用函数指针,例如applyFunction()函数接收函数指针参数并使用call_user_func()来调用函数。注意:函数指针必须是有效函数或匿名函数;无法指向私有方法;如果函数不存在则会产生
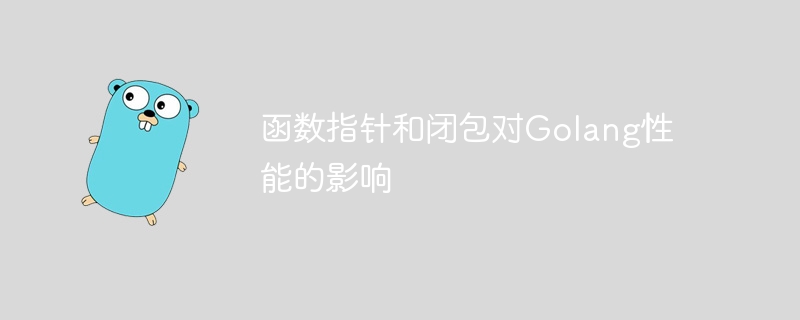
函数指针和闭包对Go性能的影响如下:函数指针:稍慢于直接调用,但可提高可读性和可复用性。闭包:通常更慢,但可封装数据和行为。实战案例:函数指针可优化排序算法,闭包可创建事件处理程序,但会带来性能损失。
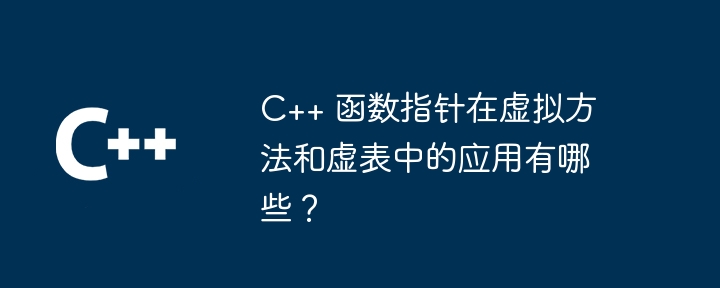
C++函数指针在虚拟方法中用于存储指向派生类重写方法实现的指针,在虚表中用于初始化虚表并存储指向虚拟方法实现的指针,从而实现运行时多态,允许派生类重写基类中的虚拟方法,并根据运行时对象的实际类型调用正确的实现。
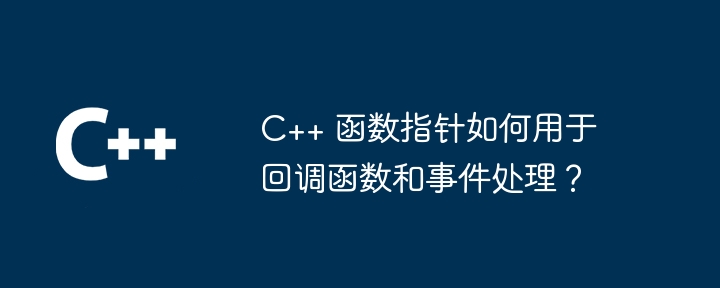
函数指针在C++中用于回调函数和事件处理,通过指向函数,允许函数传递其对方法的引用给其他函数。使用函数指针的优势包括:灵活性、可扩展性、代码解耦、可重用性以及异步通信。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
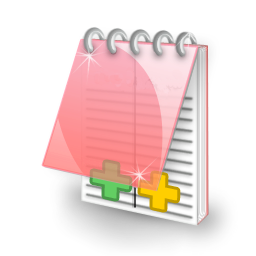
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
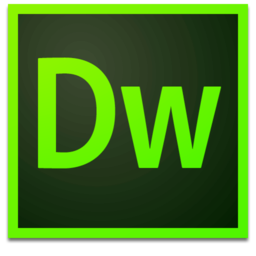
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
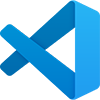
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
