C++ memory management mechanism ensures that the program uses memory efficiently through memory layout (code segment, data segment, heap, stack) and memory allocation methods (stack allocation, heap allocation). Programmers need to manually release heap memory to avoid memory leaks, which can be achieved by using the delete operator or smart pointers.
C++ memory management mechanism principle
Introduction
Memory management is in C++ An important task that ensures that programs use memory efficiently while running, thus avoiding memory leaks and segfaults.
Memory layout
The memory of a C++ program is usually divided into the following areas:
- Code segment: Stored program instructions
- Data segment: Stored program data
- Heap: Dynamically allocated memory
- Stack: Memory for local variables and function calls
Memory allocation
In C++, there are two common ways to allocate memory:
1. Stack allocation
- Use variables declared by
auto
,int
and other built-in types to allocate memory on the stack. - Memory allocation and release on the stack is automatically managed by the compiler.
2. Heap allocation
- Use the
new
operator to dynamically allocate memory on the heap. - The programmer is responsible for freeing the memory allocated on the heap using the
delete
operator.
Memory release
- Stack variable: When the function returns or ends, the stack memory will be automatically released.
-
Heap variables: Programmers must manually free heap memory using the
delete
operator. If you don't do this, a memory leak will occur.
Memory Leak
Memory leak means that memory that is no longer needed is not released, resulting in a reduction in the memory available to the system. In C++, memory leaks can be avoided by:
- Always call
delete
explicitly for heap-allocated memory. - Use smart pointers (such as
unique_ptr
andshared_ptr
) to automatically manage memory.
Practical case
Consider the following C++ code:
int* p = new int; // 在堆上分配一个整数 *p = 10; // 将值 10 存储在指向的内存中 // ... 使用 p 指针 ... delete p; // 释放堆内存
In this example, we used new
operator allocates an integer on the heap. We then use the dereference operator *
to store a value. After allocation, use delete
to free the memory to avoid memory leaks.
The above is the detailed content of C++ memory management mechanism principle. For more information, please follow other related articles on the PHP Chinese website!
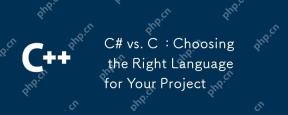
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
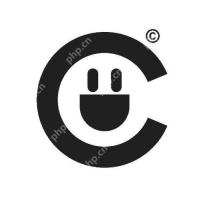
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
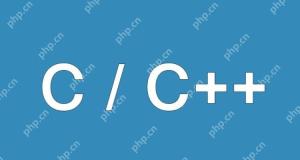
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
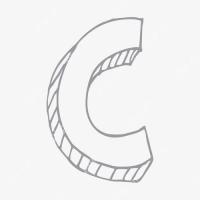
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
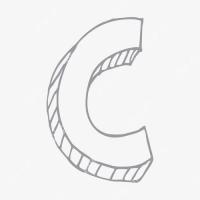
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
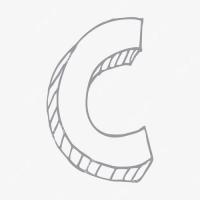
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
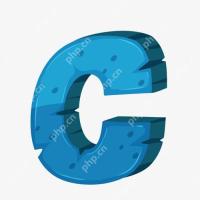
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.
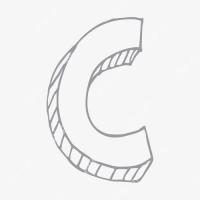
DMA in C refers to DirectMemoryAccess, a direct memory access technology, allowing hardware devices to directly transmit data to memory without CPU intervention. 1) DMA operation is highly dependent on hardware devices and drivers, and the implementation method varies from system to system. 2) Direct access to memory may bring security risks, and the correctness and security of the code must be ensured. 3) DMA can improve performance, but improper use may lead to degradation of system performance. Through practice and learning, we can master the skills of using DMA and maximize its effectiveness in scenarios such as high-speed data transmission and real-time signal processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
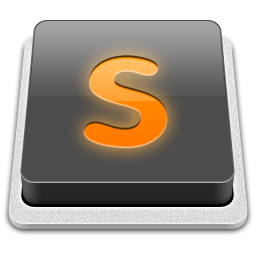
SublimeText3 Mac version
God-level code editing software (SublimeText3)
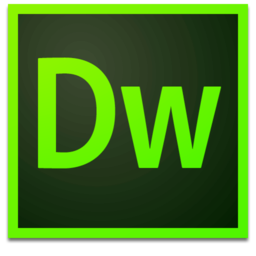
Dreamweaver Mac version
Visual web development tools
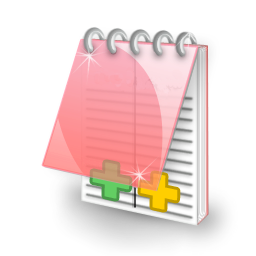
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
