Creating a MySQL table using PHP requires the following steps: Connect to the database. Create the database if it does not exist. Select a database. Create table. Execute the query. Close the connection.
How to create a MySQL table using PHP?
Creating a MySQL table using PHP is a simple process, which involves the following steps:
Prerequisites:
- PHP is installed and configured
- MySQL database server
- MySQL database user and password
Code:
<?php // 连接到 MySQL 数据库 $servername = "localhost"; $username = "root"; $password = "mypassword"; $dbname = "myDB"; // 创建连接 $conn = new mysqli($servername, $username, $password); // 检查连接 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 创建数据库(如果不存在) $sql = "CREATE DATABASE IF NOT EXISTS $dbname"; if ($conn->query($sql) === TRUE) { echo "数据库创建成功"; } else { echo "数据库创建失败: " . $conn->error; } // 选择数据库 $conn->select_db($dbname); // 创建表 $sql = "CREATE TABLE IF NOT EXISTS users ( id INT NOT NULL AUTO_INCREMENT, username VARCHAR(255) NOT NULL, email VARCHAR(255) NOT NULL, PRIMARY KEY (id) )"; // 执行查询 if ($conn->query($sql) === TRUE) { echo "表创建成功"; } else { echo "表创建失败: " . $conn->error; } // 关闭连接 $conn->close(); ?>
Practical case:
Suppose you want to create a A table named "users" with columns "id", "username", and "email". You can use the following code:
// 连接到 MySQL 数据库 $conn = new mysqli($servername, $username, $password, $dbname); // 创建表 $sql = "CREATE TABLE IF NOT EXISTS users ( id INT NOT NULL AUTO_INCREMENT, username VARCHAR(255) NOT NULL, email VARCHAR(255) NOT NULL, PRIMARY KEY (id) )"; // 执行查询 if ($conn->query($sql) === TRUE) { echo "表创建成功"; } else { echo "表创建失败: " . $conn->error; } // 关闭连接 $conn->close();
The above is the detailed content of How to create a MySQL table using PHP?. For more information, please follow other related articles on the PHP Chinese website!
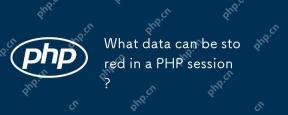
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
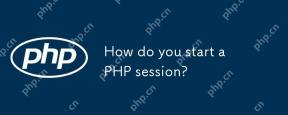
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
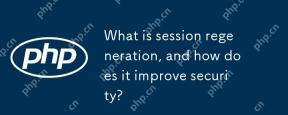
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.
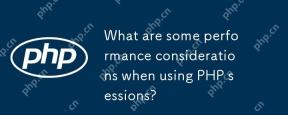
PHP sessions have a significant impact on application performance. Optimization methods include: 1. Use a database to store session data to improve response speed; 2. Reduce the use of session data and only store necessary information; 3. Use a non-blocking session processor to improve concurrency capabilities; 4. Adjust the session expiration time to balance user experience and server burden; 5. Use persistent sessions to reduce the number of data read and write times.
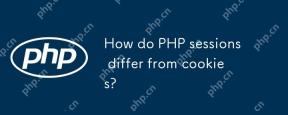
PHPsessionsareserver-side,whilecookiesareclient-side.1)Sessionsstoredataontheserver,aremoresecure,andhandlelargerdata.2)Cookiesstoredataontheclient,arelesssecure,andlimitedinsize.Usesessionsforsensitivedataandcookiesfornon-sensitive,client-sidedata.
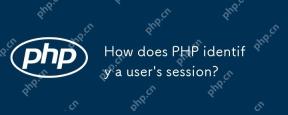
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
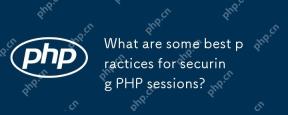
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
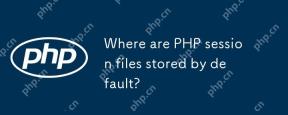
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
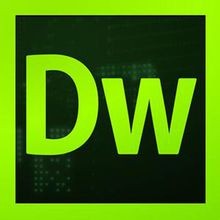
Dreamweaver CS6
Visual web development tools
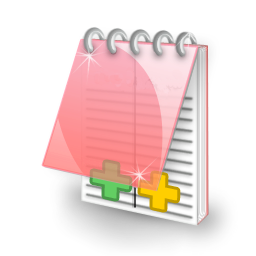
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
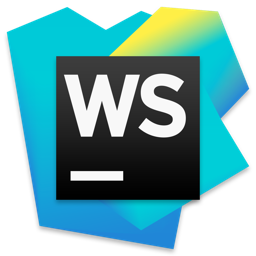
WebStorm Mac version
Useful JavaScript development tools
