How to optimize network performance in Java to improve efficiency?
How to optimize network performance in Java for greater efficiency Using non-blocking I/O: allows applications to wait for I/O operations while continuing to perform other tasks. Tuning TCP buffer sizes: Optimize performance in high-throughput environments by adjusting buffer sizes. Use connection pooling: Reuse existing connections to reduce overhead and improve performance. Use a CDN: Caches content to improve response times and download speeds. Compressed transfer: Reduce the amount of data transferred to increase transfer speed.
Optimizing Network Performance for Efficiency in Java
In today’s fast-paced digital environment, network performance is crucial for any application is crucial to success. In Java, you can improve the efficiency and responsiveness of your applications by adopting the following best practices to optimize network performance.
1. Use non-blocking I/O
Non-blocking I/O allows an application to continue performing other tasks while waiting for an I/O operation to complete. The java.nio
package in Java provides support for non-blocking I/O. By using non-blocking I/O, an application can significantly increase throughput because it does not need to block on I/O operations.
Code example:
import java.nio.channels.AsynchronousSocketChannel; import java.net.InetSocketAddress; import java.util.concurrent.Future; public class NonBlockingClient { public static void main(String[] args) throws Exception { AsynchronousSocketChannel client = AsynchronousSocketChannel.open(); Future<Void> future = client.connect(new InetSocketAddress("localhost", 8080)); future.get(); // 等待连接建立 // 发送数据并接收响应 ... client.close(); } }
2. Tuning TCP buffer size
TCP buffer size control is used for sending and buffer size for receiving data. In high-throughput environments, larger buffers can improve performance. The buffer size can be adjusted through the Socket.setReceiveBufferSize()
and Socket.setSendBufferSize()
methods.
Code sample:
import java.net.Socket; public class BufferSizeTuning { public static void main(String[] args) throws Exception { Socket socket = new Socket("localhost", 8080); // 设置接收缓冲区大小为 16KB socket.setReceiveBufferSize(16 * 1024); // 设置发送缓冲区大小为 32KB socket.setSendBufferSize(32 * 1024); } }
3. Using connection pool
Creating and closing socket connections consumes a lot of resources . By using connection pools, applications can reuse existing connections, reducing overhead and improving performance. In Java, you can use the java.sql.ConnectionPoolDataSource
interface or a third-party library (such as HikariCP) to manage the connection pool.
Code example:
import javax.sql.ConnectionPoolDataSource; import javax.sql.PooledConnection; public class ConnectionPooling { public static void main(String[] args) throws Exception { ConnectionPoolDataSource dataSource = ...; // 从连接池获取连接 PooledConnection connection = dataSource.getPooledConnection(); // 使用连接 ... // 将连接归还给连接池 connection.close(); } }
4. Using CDN
Content Delivery Network (CDN) can be used to Caching content on edge servers to improve response times and download speeds. For applications that need to serve large amounts of static content, a CDN can significantly improve performance.
5. Compressed transmission
Compressed network transmission can reduce the amount of data to be sent, thereby increasing the transmission speed. You can use the java.util.zip
package to compress and decompress data in Java.
Code example:
import java.io.ByteArrayInputStream; import java.io.ByteArrayOutputStream; import java.io.IOException; import java.util.zip.GZIPOutputStream; import java.util.zip.GZIPInputStream; public class DataCompression { public static void main(String[] args) throws IOException { // 压缩数据 ByteArrayOutputStream compressedBytes = new ByteArrayOutputStream(); try (GZIPOutputStream gzip = new GZIPOutputStream(compressedBytes)) { gzip.write("Hello, world!".getBytes()); } // 解压缩数据 ByteArrayInputStream compressedStream = new ByteArrayInputStream(compressedBytes.toByteArray()); try (GZIPInputStream gzip = new GZIPInputStream(compressedStream)) { byte[] decompressedBytes = new byte[1024]; int count = gzip.read(decompressedBytes); String decompressedString = new String(decompressedBytes, 0, count); } } }
The above is the detailed content of How to optimize network performance in Java to improve efficiency?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
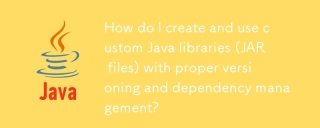
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
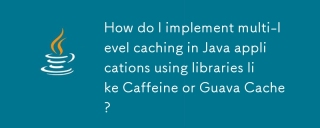
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
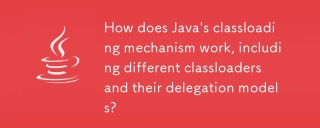
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
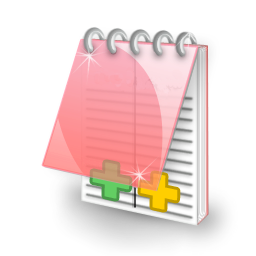
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.