What are the best practices for packages in Go?
Go language package best practices include: following naming conventions, naming packages in lowercase, and naming visible types, variables, and constants in uppercase. Organize components, including init() functions, interfaces, structures, and functions. Use relative paths to import internal packages to avoid circular dependencies. Write tests for packages covering various inputs and edge cases. Provide documentation, including documentation of package names, descriptions, types and functions, and error types in exported packages.
Best practices for packages in the Go language
In the Go language, packages are used to organize and encapsulate related code. Best practices for using packages help keep your codebase maintainable and readable. This article will introduce the best practices for using packages in the Go language and a practical case.
Naming convention
- Package names should be lowercase and unique (within a single repository).
- The first letter of names of externally visible types, variables, and constants should be capitalized.
Code Structure
-
The package should be organized by the following components:
-
init ()
Function: Executed once when the package is loaded. - Interface: Defines a set of methods that can be implemented by other types.
- Struct: Defines the data structure and can contain other types.
- Function: Implement a specific function or operation.
-
- Related functions should be placed together and arranged in a logical order.
Dependency Management
- Use dependency management tools such as Go Modules to record and track package dependencies.
- When importing internal packages into other packages, use relative paths.
- Avoid circular dependencies as much as possible.
Testing
- Write tests for the package to verify its functionality.
- Tests should cover various inputs and edge cases.
Documentation
- Provide comments for the package to explain its purpose and how to use it.
-
Package documentation should contain the following:
- Package name and description
- Documentation of externally visible types and functions
- In exported packages Error types
Practical case: String manipulation package
Let’s create a string manipulation package that demonstrates these best practices Practice:
package strutil import "strings" // TrimAllSpaces 删除字符串中的所有空格字符。 func TrimAllSpaces(s string) string { return strings.ReplaceAll(s, " ", "") } // ReverseString 反转字符串。 func ReverseString(s string) string { runes := []rune(s) for i, j := 0, len(runes)-1; i < len(runes)/2; i, j = i+1, j-1 { runes[i], runes[j] = runes[j], runes[i] } return string(runes) } // IsPalindrome 检查字符串是否为回文。 func IsPalindrome(s string) bool { return s == ReverseString(s) }
Benefits of using these best practices
- Readability: Well-organized package code is easier to read and understand.
- Maintainability: Following naming conventions and code structure helps avoid unexpected breaks when modifying the package.
- Reusability: Clear dependencies and documentation help reuse packages in other projects.
- Testability: Writing tests helps ensure that the package will work correctly in a variety of situations.
The above is the detailed content of What are the best practices for packages in Go?. For more information, please follow other related articles on the PHP Chinese website!
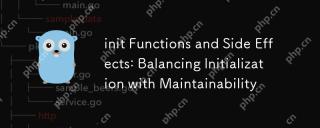
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
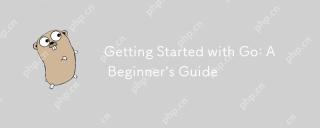
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
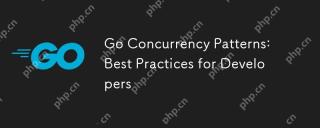
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.
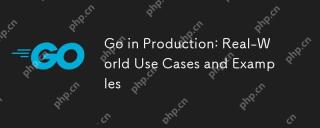
Goexcelsinproductionduetoitsperformanceandsimplicity,butrequirescarefulmanagementofscalability,errorhandling,andresources.1)DockerusesGoforefficientcontainermanagementthroughgoroutines.2)UberscalesmicroserviceswithGo,facingchallengesinservicemanageme
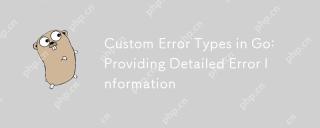
We need to customize the error type because the standard error interface provides limited information, and custom types can add more context and structured information. 1) Custom error types can contain error codes, locations, context data, etc., 2) Improve debugging efficiency and user experience, 3) But attention should be paid to its complexity and maintenance costs.
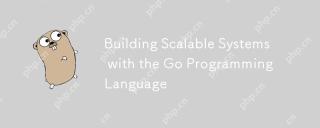
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
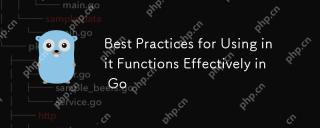
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
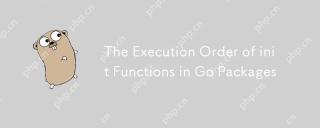
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
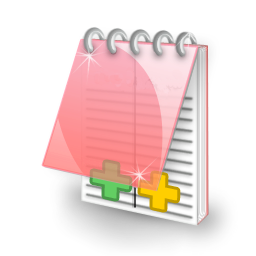
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
